7.3. Variables#
While basf2
operates on ParticleList s, it is also important to calculate physics quantities associated with a given candidate or event.
In basf2
analysis, variables are handled by the VariableManager
.
There are many variables available for use in analysis.
Probably the most obvious, and useful are: p
, E
, Mbc
, and deltaE
.
You can search the variables in an alphabetical basf2 Variable Index, or browse Variables by group.
7.3.1. VariableManager#
The VariableManager handles all variables in basf2
analysis.
It is implemented as a singleton
C++ class with a python interface.
The C++ documentation is here.
Tip
For (unfortunate) historical reasons, the python accessor to the VariableManager
singleton is called variables
and is in the python variables
module.
This leads to strange-looking python import
commands.
For example:
from variables import variables
To avoid confusion, example/tutorial scripts often use a namespace alias vm
.
You might want to use this in your scripts.
from variables import variables as vm # shorthand for VariableManager
- class VariableManager#
Singleton class to hold all variables and aliases in the current scope.
- addAlias(alias, expression)#
Create a new alias.
Variable names are deliberately verbose and explicit (to avoid ambiguity). However, it is often not desirable to deal with long unwieldy variable names particularly in the context of Variable Manager Output.
Example:
Aliases to a verbose variable may be set with:
>>> from variables import variables as vm >>> vm.addAlias("shortname", "aReallyLongAndSpecificVariableName(1, 2, 3)")
See also
variables.utils.create_aliases
andvariables.utils.create_aliases_for_selected
might be helpful if you find yourself setting many aliases in your analysis script.Warning
The VariableManager instance is configured independently of the
basf2.Path
. In case of adding the same alias twice, the configuration just before callingbasf2.process
is what wins.
- getAliasNames()#
Get a list of all alias names (in reverse order added)
Tip
This returns a
ROOT.vector
which you will probably need to convert into a pythonlist(str)
.>>> my_aliases = list(vm.getAliasNames())
- Returns:
ROOT.vector
list of alias names
- addCollection(collection, variables)#
Create a new variable collection.
Tip
This method takes a
ROOT.vector<string>
as input. It’s probably easier to usevariables.utils.add_collection
which wraps this function for you.- Parameters:
collection (str) – The new collection to create.
variables – A
ROOT.std.vector(string)
instance of variables to add as the variable collection.
- Returns:
True if the collection was successfully added
- getCollection(collection)#
Get a list of all variables in the
collection
.- Parameters:
collection (str) – The name of the existing variable collection
- Returns:
ROOT.vector
list of variable names
- printAliases()#
Prints all aliases currently registered. Useful to call just before calling
basf2.process
on an analysisbasf2.Path
when debugging.
7.3.2. Variables by group#
Here is a categorised list of variables known to basf2
.
You can also look at the alphabetical index: basf2 Variable Index.
Kinematics#
- ArmenterosDaughter1Qt#
Transverse momentum of the first daughter with respect to the V0 mother. The mother is required to have exactly two daughters
- Unit:
GeV/c
- ArmenterosDaughter2Qt#
Transverse momentum of the second daughter with respect to the V0 mother. The mother is required to have exactly two daughters
- Unit:
GeV/c
- ArmenterosLongitudinalMomentumAsymmetry#
Longitudinal momentum asymmetry of V0’s daughters. The mother (V0) is required to have exactly two daughters
- E#
energy
- Unit:
GeV
- ECLClusterE_uncertainty#
energy uncertainty as given by the underlying ECL cluster
- Unit:
GeV
- E_uncertainty#
energy uncertainty (\(\sqrt{\sigma^2}\))
- Unit:
GeV
- ErrM#
uncertainty of invariant mass
- Unit:
GeV/\(\text{c}^2\)
- ImpactXY#
The impact parameter of the given particle in the xy plane
- Unit:
cm
- InvM#
invariant mass (determined from particle’s daughter 4-momentum vectors). If this particle is V0 or decays at rho > 5 mm, its daughter 4-momentum vectors at fitted vertex are taken. If this particle has no daughters, defaults to
M
.- Unit:
GeV/\(\text{c}^2\)
- InvMLambda#
Invariant mass (determined from particle’s daughter 4-momentum vectors), assuming the first daughter is a pion and the second daughter is a proton. If the particle has not 2 daughters, it returns just the mass value.
- Unit:
GeV/\(\text{c}^2\)
- M#
The particle’s mass.
Note that this is context-dependent variable and can take different values depending on the situation. This should be the “best” value possible with the information provided.
If this particle is track- or cluster- based, then this is the value of the mass hypothesis.
If this particle is an MC particle then this is the mass of that particle.
If this particle is composite, then initially this takes the value of the invariant mass of the daughters.
If this particle is composite and a mass or vertex fit has been performed then this may be updated by the fit.
You will see a difference between this mass and the
InvM
.
- Unit:
GeV/\(\text{c}^2\)
- M2#
The particle’s mass squared.
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- Mbc#
beam constrained mass
- Unit:
GeV/\(\text{c}^2\)
- PDG#
PDG code
- Q#
energy released in decay
- Unit:
GeV
- SigM#
signed deviation of particle’s invariant mass from its nominal mass in units of the uncertainty on the invariant mass (
dM
/ErrM
)
- b2bClusterPhi#
Azimuthal angle in the lab system that is back-to-back to the particle’s associated ECLCluster in the CMS. Returns NAN if no cluster is found. Useful for low multiplicity studies.
- Unit:
rad
- b2bClusterTheta#
Polar angle in the lab system that is back-to-back to the particle’s associated ECLCluster in the CMS. Returns NAN if no cluster is found. Useful for low multiplicity studies.
- Unit:
rad
- b2bPhi#
Azimuthal angle in the lab system that is back-to-back to the particle in the CMS. Useful for low multiplicity studies.
- Unit:
rad
- b2bTheta#
Polar angle in the lab system that is back-to-back to the particle in the CMS. Useful for low multiplicity studies.
- Unit:
rad
- cosAngleBetweenMomentumAndVertexVector#
cosine of the angle between momentum and vertex vector (vector connecting ip and fitted vertex) of this particle
- cosAngleBetweenMomentumAndVertexVectorInXYPlane#
cosine of the angle between momentum and vertex vector (vector connecting ip and fitted vertex) of this particle in xy-plane
- cosTheta#
momentum cosine of polar angle
- cosThetaBetweenParticleAndNominalB#
cosine of the angle in CMS between momentum the particle and a nominal B particle. It is somewhere between -1 and 1 if only a massless particle like a neutrino is missing in the reconstruction.
- cosThetaErr#
error of momentum cosine of polar angle
- cosToThrustOfEvent#
Returns the cosine of the angle between the particle and the thrust axis of the event, as calculate by the EventShapeCalculator module. buildEventShape() must be run before calling this variable
- dM#
mass minus nominal mass
- Unit:
GeV/\(\text{c}^2\)
- eRecoil#
energy recoiling against given Particle
- Unit:
GeV
- m2Recoil#
invariant mass squared of the system recoiling against given Particle
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- m2RecoilSignalSide#
Squared recoil mass of the signal side which is calculated in the CMS frame under the assumption that the signal and tag side are produced back to back and the tag side energy equals the beam energy. The variable must be applied to the Upsilon and the tag side must be the first, the signal side the second daughter
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- mRecoil#
Invariant mass of the system recoiling against given Particle
- Unit:
GeV/\(\text{c}^2\)
- momDevChi2#
momentum deviation \(\chi^2\) value calculated as \(\chi^2 = \sum_i (p_i - mc(p_i))^2/\sigma(p_i)^2\), where \(\sum\) runs over i = px, py, pz and \(mc(p_i)\) is the mc truth value and \(\sigma(p_i)\) is the estimated error of i-th component of momentum vector
- momVertCovM(i, j)#
returns the (i,j)-th element of the MomentumVertex Covariance Matrix (7x7). Order of elements in the covariance matrix is: px, py, pz, E, x, y, z.
- Unit:
GeV/c, GeV/c, GeV/c, GeV, cm, cm, cm
- p#
momentum magnitude
- Unit:
GeV/c
- pErr#
error of momentum magnitude
- Unit:
GeV/c
- pRecoil#
magnitude of 3 - momentum recoiling against given Particle
- Unit:
GeV/c
- pRecoilPhi#
Azimuthal angle of a particle’s missing momentum
- Unit:
rad
- pRecoilTheta#
Polar angle of a particle’s missing momentum
- Unit:
rad
- phi#
momentum azimuthal angle
- Unit:
rad
- phiErr#
error of momentum azimuthal angle
- Unit:
rad
- pt#
transverse momentum
- Unit:
GeV/c
- ptErr#
error of transverse momentum
- Unit:
GeV/c
- px#
momentum component x
- Unit:
GeV/c
- pxErr#
error of momentum component x
- Unit:
GeV/c
- pxRecoil#
component x of 3-momentum recoiling against given Particle
- Unit:
GeV/c
- py#
momentum component y
- Unit:
GeV/c
- pyErr#
error of momentum component y
- Unit:
GeV/c
- pyRecoil#
component y of 3-momentum recoiling against given Particle
- Unit:
GeV/c
- pz#
momentum component z
- Unit:
GeV/c
- pzErr#
error of momentum component z
- Unit:
GeV/c
- pzRecoil#
component z of 3-momentum recoiling against given Particle
- Unit:
GeV/c
- theta#
polar angle
- Unit:
rad
- thetaErr#
error of polar angle
- Unit:
rad
- xp#
scaled momentum: the momentum of the particle in the CMS as a fraction of its maximum available momentum in the collision
Helicity#
- acoplanarityAngle#
Acoplanarity angle (see
Particle::getAcoplanarity
) assuming a two body decay of the particle and its daughters. See PDG Polarization Review for the definition of the acoplanarity angle.- Unit:
rad
- cosAcoplanarityAngle(i, j)#
Cosine of the acoplanarity angle (\(\Phi\) in the PDG Polarization Review). Given a two-body decay, the acoplanarity angle is defined as the angle between the two decay planes in the reference frame of the mother.
We calculate the acoplanarity angle as the angle between the two normal vectors of the decay planes. Each normal vector is the cross product of the momentum of one daughter (in the frame of the mother) and the momentum of one of the granddaughters (in the reference frame of the daughter).
This variable needs two integer arguments: the first one,
i
is the index of the first granddaughter, and the second one,j
the index of the second granddaughter.For example, in the decay \(B^0 \to \left(J/\psi \to \mu^+ \mu^-\right) \left(K^{*0} \to K^+ \pi^-\right)\), if the provided particle is \(B^0\) and the selected indices are (0, 0), the variable will return the acoplanarity using the \(\mu^+\) and the \(K^+\) granddaughters.
- cosHelicityAngle(i, j)#
Cosine of the helicity angle between the momentum of the selected granddaughter and the direction opposite to the momentum of the provided particle in the reference frame of the selected daughter (\(\theta_1\) and \(\theta_2\) in the PDG 2018, p. 722).
This variable needs two integer arguments: the first one,
i
, is the index of the daughter and the second one,j
is the index of the granddaughter.For example, in the decay \(B^0 \to \left(J/\psi \to \mu^+ \mu^-\right) \left(K^{*0} \to K^+ \pi^-\right)\), if the provided particle is \(B^0\) and the selected indices are (0, 0), the variable will return the angle between the momentum of the \(\mu^+\) and the direction opposite to the momentum of the \(B^0\), both momenta in the rest frame of the \(J/\psi\).
This variable is needed for angular analyses of \(B\)-meson decays into two vector particles.
- cosHelicityAngleBeamMomentum(i)#
Cosine of the helicity angle of the \(i\)-th daughter of the particle provided, assuming that the mother of the provided particle corresponds to the centre-of-mass system, whose parameters are automatically loaded by the function, given the accelerator’s conditions.
- cosHelicityAngleDaughter(i [, j])#
Cosine of the helicity angle of the i-th daughter (see
Particle::getCosHelicityDaughter
). The optional second argument is the index of the granddaughter that defines the angle, default is 0.For example, in the decay: \(B^0 \to \left(J/\psi \to \mu^+ \mu^-\right) \left(K^{*0} \to K^+ \pi^-\right)\), if the provided particle is \(B^0\) and the selected index is 0, the variable will return the helicity angle of the \(\mu^+\). If the selected index is 1 the variable will return the helicity angle of the \(K^+\) (defined via the rest frame of the \(K^{*0}\)). In rare cases if one wanted the helicity angle of the second granddaughter, indices 1,1 would return the helicity angle of the \(\pi^-\)).
See PDG Polarization Review for the definition of the helicity angle.
- cosHelicityAngleForQuasiTwoBodyDecay(i, j)#
Cosine of the helicity angle between the momentum of the provided particle and the momentum of the first selected daughter (i-th) in the reference frame of the sum of two selected daughters (i-th + j-th).
The variable is supposed to be used for the analysis of a quasi-two-body decay. The number of daughters of the given particle must be three. Otherwise, the variable returns NaN.
For example, in the decay \(\bar{B}^0 \to D^+ K^- K^{*0}\), if the provided particle is \(\bar{B}^0\) and the selected indices are (1, 2), the variable will return the angle between the momentum of the \(\bar{B}^0\) and the momentum of the \(K^-\), both momenta in the rest frame of the \(K^- K^{*0}\).
- cosHelicityAngleMomentum#
If the given particle has two daughters: cosine of the angle between the line defined by the momentum difference of the two daughters in the frame of the given particle (mother) and the momentum of the given particle in the lab frame.
If the given particle has three daughters: cosine of the angle between the normal vector of the plane defined by the momenta of the three daughters in the frame of the given particle (mother) and the momentum of the given particle in the lab frame.
Otherwise, it returns 0.
- cosHelicityAngleMomentumPi0Dalitz#
To be used for the decay \(\pi^0 \to e^+ e^- \gamma\): cosine of the angle between the momentum of the gamma in the frame of the given particle (mother) and the momentum of the given particle in the lab frame.
One can call the variable for the decay \(\pi^0 \to \gamma \gamma, \gamma \to e^+ e^-\) as well.
Otherwise, it returns 0.
- cosHelicityAnglePrimary#
Cosine of the helicity angle (see``Particle::getCosHelicity``) assuming the center of mass system as mother rest frame. See PDG Polarization Review for the definition of the helicity angle.
- momentaTripleProduct(i, j, k)#
a triple-product of three momenta of offspring in the mother rest frame: \(C_T=\vec{p}_i\cdot(\vec{p}_j\times\vec{p}_k)\). For examples, In a four-body decay M->D1D2D3D4, momentaTripleProduct(0,1,2) returns CT using the momenta of D1D2D3 particles. In other decays involving secondary decay, e.g. for M->(R->D1D2)D3D4, momentaTripleProduct(0:0,1,2) returns C_T using momenta of D1D3D4 particles.
Tracking#
Here is a list of track variables. In the following descriptions, PR refers to “pattern recognition” tracks (i.e. reconstructed). And MC refers to MC tracks. This notation follows the convention of the tracking paper.
See also
For more details see: “Track finding at Belle II” Comput.Phys.Commun. 259 (2021), 107610
- chi2#
Returns the \(\chi^2\) of the track fit. This is actually computed based on
pValue
andndf
.Note
Note that for
pValue
exactly equal to 0 it returns infinity.Returns NaN if called for something other than a track-based particle, or for mdst files processed with basf2 versions older than
release-05-01
.
- d0#
Returns the tracking parameter \(d_0\), the signed distance to the point-of-closest-approach (POCA) in the \(r-\phi\) plane.
Note
Tracking parameters are with respect to the origin (0,0,0). For the POCA with respect to the measured beam interaction point, see
dr
(you probably want this unless you’re doing a tracking study or some debugging) andd0FromIP
.Returns NaN if called for something other than a track-based particle.
- Unit:
cm
- d0Err#
Returns the uncertainty on \(d_0\), the signed distance to the point-of-closest-approach (POCA) in the \(r-\phi\) plane.
Returns NaN if called for something other than a track-based particle.
- Unit:
cm
- d0FromIP#
Returns the tracking parameter \(d_0\), the signed distance to the point-of-closest-approach (POCA) in the \(r-\phi\) plane, with respect to the measured beam interaction point.
Returns NaN if called for something other than a track-based particle.
- Unit:
cm
- d0Pull#
The pull of the tracking parameter \(d_0\) for the reconstructed pattern-recognition track, with respect to the MC track. That is:
\[\frac{d_0^\textrm{MC} - d_0^\textrm{PR}}{\sigma_{d_0; \textrm{PR}}}\]Returns NaN if no MC particle is related or if called on something other than a track-based particle.
- firstCDCLayer#
The first activated CDC layer associated to the track. Returns NaN if called for something other than a track-based particle.
- firstPXDLayer#
The first activated PXD layer associated to the track. Returns NaN if called for something other than a track-based particle.
- firstSVDLayer#
The first activated SVD layer associated to the track. Returns NaN if called for something other than a track-based particle.
- hasExtraCDCHitsInLayer(i)#
[Eventbased] Returns 1 if a non-assigned hit exists in the specified CDC layer, 0 otherwise.
Returns NaN if there is no event-level tracking information available.
- hasExtraCDCHitsInSuperLayer(i)#
[Eventbased] Returns 1 if a non-assigned hit exists in the specified CDC SuperLayer, 0 otherwise.
Returns NaN if there is no event-level tracking information available.
- helixExtPhi(radius, z fwd, z bwd, useHighestProbMass=0)#
Returns phi of extrapolated helix parameters. If
useHighestProbMass=1
is set, the extrapolation will use the track fit result for the mass hypothesis with the highest pValue.- Unit:
rad
- helixExtPhiOnDet(detector_surface_name, useHighestProbMass=0)#
- Returns phi of extrapolated helix parameters on the given detector surface. The unit of angle is
rad
. If
useHighestProbMass=1
is set, the extrapolation will use the track fit result for the mass hypothesis with the highest pValue. The supported detector surface names are{'CDC', 'TOP', 'ARICH', 'ECL', 'KLM'}
. Also, the detector name with number of meaningful-layer is supported, e.g.'CDC8'
: last superlayer of CDC,'ECL1'
: mid-point of ECL...note:: You can find more information in
modularAnalysis.calculateTrackIsolation
.
- Returns phi of extrapolated helix parameters on the given detector surface. The unit of angle is
- helixExtTheta(radius [cm], z fwd [cm], z bwd [cm], useHighestProbMass=0)#
- Returns theta of extrapolated helix parameters. If
useHighestProbMass=1
is set, the extrapolation will use the track fit result for the mass hypothesis with the highest pValue.
- Unit:
rad
- Returns theta of extrapolated helix parameters. If
- helixExtThetaOnDet(detector_surface_name, useHighestProbMass=0)#
- Returns theta of extrapolated helix parameters on the given detector surface. The unit of angle is
rad
. If
useHighestProbMass=1
is set, the extrapolation will use the track fit result for the mass hypothesis with the highest pValue. The supported detector surface names are{'CDC', 'TOP', 'ARICH', 'ECL', 'KLM'}
. Also, the detector name with number of meaningful-layer is supported, e.g.'CDC8'
: last superlayer of CDC,'ECL1'
: mid-point of ECL...note:: You can find more information in
modularAnalysis.calculateTrackIsolation
.
- Returns theta of extrapolated helix parameters on the given detector surface. The unit of angle is
- isTrackFlippedAndRefitted#
Returns 1 if the charged final state particle comes from a track that has been flipped and refitted at the end of the reconstruction chain, in particular after the outer detector reconstruction.
- lastCDCLayer#
The last CDC layer associated to the track. Returns NaN if called for something other than a track-based particle.
- mcD0#
Returns the MC value of \(d_0\), the signed distance to the point-of-closest-approach (POCA) in the \(r-\phi\) plane.
See also
Returns NaN if the particle is not related to any MCParticle.
- Unit:
cm
- mcOmega#
Returns the MC value of \(\omega\), the curvature of the track.
See also
Returns NaN if the particle is not related to any MCParticle.
- Unit:
\(\text{cm}^{-1}\)
- mcPhi0#
Returns the MC value of \(\phi_0\), the angle of the transverse momentum in the \(r-\phi\) plane.
See also
Returns NaN if the particle is not related to any MCParticle.
- Unit:
rad
- mcTanLambda#
Returns the MC value of \(\tan\lambda\), the slope of the track in the \(r-z\) plane.
See also
Returns NaN if the particle is not related to any MCParticle.
- mcZ0#
Returns the MC value of \(z_0\), the z-coordinate of the point-of-closest-approach (POCA).
See also
Returns NaN if the particle is not related to any MCParticle.
- Unit:
cm
- nCDCHits#
The number of CDC hits associated to the track. Returns NaN if called for something other than a track-based particle.
- nExtraCDCHits#
[Eventbased] The number of CDC hits in the event not assigned to any track.
Returns NaN if there is no event-level tracking information available.
- nExtraCDCHitsPostCleaning#
[Eventbased] Returns a count of the number of CDC hits in the event not assigned to any track nor very likely beam background (i.e. hits that survive a cleanup selection).
Returns NaN if there is no event-level tracking information available.
- nExtraCDCSegments#
[Eventbased] Returns the number of CDC segments not assigned to any track.
Returns NaN if there is no event-level tracking information available.
- nPXDHits#
The number of PXD hits associated to the track. Returns NaN if called for something other than a track-based particle.
- nSVDHits#
The number of SVD hits associated to the track. Returns NaN if called for something other than a track-based particle.
- nVXDHits#
The number of PXD and SVD hits associated to the track. Returns NaN if called for something other than a track-based particle.
- ndf#
Returns the number of degrees of freedom of the track fit.
Note
Note that this is not simply the number of hits -5 due to outlier hit rejection.
Returns NaN if called for something other than a track-based particle, or for mdst files processed with basf2 versions older than
release-05-01
.
- omega#
Returns the tracking parameter \(\omega\), the curvature of the track.
Returns NaN if called for something other than a track-based particle.
- Unit:
\(\text{cm}^{-1}\)
- omegaErr#
Returns the uncertainty on \(\omega\), the curvature of the track.
Returns NaN if called for something other than a track-based particle.
- Unit:
\(\text{cm}^{-1}\)
- omegaPull#
The pull of the tracking parameter \(\omega\) for the reconstructed pattern-recognition track, with respect to the MC track. That is:
\[\frac{\omega^\textrm{MC} - \omega^\textrm{PR}}{\sigma_{\omega; \textrm{PR}}}\]Returns NaN if no MC particle is related or if called on something other than a track-based particle.
- pValue#
The \(\chi^2\) probability of the track fit.
Note
This is the p-value of the track-fit. It does not get updated after vertex fitting or kinematic fitting, and is meaningless for composite particles.
See
chiProb
(you probably want this for high-level analysis).Returns NaN if called for something other than a track-based particle.
- phi0#
Returns the tracking parameter \(\phi_0\), the angle of the transverse momentum in the \(r-\phi\) plane.
Note
Tracking parameters are with respect to the origin (0,0,0). For the POCA with respect to the measured beam interaction point, see
phi0FromIP
.Returns NaN if called for something other than a track-based particle.
- Unit:
rad
- phi0Err#
Returns the uncertainty on \(\phi_0\), the angle of the transverse momentum in the \(r-\phi\) plane.
Returns NaN if called for something other than a track-based particle.
- Unit:
rad
- phi0FromIP#
Returns the tracking parameter \(\phi_0\), the angle of the transverse momentum in the \(r-\phi\) plane, with respect to the measured beam interaction point.
Returns NaN if called for something other than a track-based particle.
- Unit:
rad
- phi0Pull#
The pull of the tracking parameter \(\phi_0\) for the reconstructed pattern-recognition track, with respect to the MC track. That is:
\[\frac{\phi_0^\textrm{MC} - \phi_0^\textrm{PR}}{\sigma_{\phi_0; \textrm{PR}}}\]Returns NaN if no MC particle is related or if called on something other than a track-based particle.
- tanLambda#
Returns \(\tan\lambda\), the slope of the track in the \(r-z\) plane.
Returns NaN if called for something other than a track-based particle.
- tanLambdaErr#
Returns the uncertainty on \(\tan\lambda\), the slope of the track in the \(r-z\) plane.
See also
Returns NaN if called for something other than a track-based particle.
- tanLambdaPull#
The pull of the tracking parameter \(\tan\lambda\) for the reconstructed pattern-recognition track, with respect to the MC track. That is:
\[\frac{(\tan\lambda)^\textrm{MC} - (\tan\lambda)^\textrm{PR}}{\sigma_{\tan\lambda; \textrm{PR}}}\]See also
Returns NaN if no MC particle is related or if called on something other than a track-based particle.
- trackFindingFailureFlag#
[Eventbased] Returns a flag set by the tracking if there is reason to assume there was a track in the event missed by the tracking, or the track finding was (partly) aborted for this event.
Returns NaN if there is no event-level tracking information available.
- trackFitCovariance(i, j)#
The track fit covariance matrix element corresponding to the two indices is returned. This is the association between integers and parameters:
0: \(d_0\)
1: \(\phi_0\)
2: \(\omega\)
3: \(z_0\)
4: \(\tan\lambda\)
Note
The covariance is returned. This means that the return value can be negative. Furthermore, it’s the squared value of the track fit error variables
d0Err
, etc. when selecting the diagonal entries.
- trackFitHypothesisPDG#
Returns the PDG code of the track hypothesis actually used for the fit. Returns NaN if called for something other than a track-based particle.
- trackLength#
Returns the arc length of the helix for the TrackFitResult associated with the particle. The arc length is measured from the track origin to the radius of the CDC layer in which the Track has a hit. Returns NaN if the particle has no CDC Hits.
- Unit:
cm
- trackNECLClusters#
Returns a count of the number of ECLClusters matched to the track. This is always 0 or 1 with newer versions of ECL reconstruction.
Note
For high-level analysis it is recommended to require the presence of a matched ECL cluster along with a minimum energy requirement. A track-based particle will have a clusterE if it is matched (NaN if there is no cluster match for the track.
import modularAnalysis as ma # minimum energy of 200 MeV ma.fillParticleList("e+:clusters", "clusterE > 0.2", path) # these two are equivalent ma.fillParticleList("e+:unmatched", "isNAN(clusterE) == 1", path) ma.fillParticleList("e+:unmatched2", "trackNECLClusters == 0", path)
Returns NaN if called for something other than a track-based particle.
- trackTime#
Returns the time at which the track is produced relative to the time of the collision (given by SVD EventT0). Both the time of the collision and the track time are computed using only SVD hits. Returns NaN if SVD EventT0 is NaN, or if no SVD Hits are attached to the track. For more details, see Time Extraction page.
- Unit:
ns
- z0#
Returns the tracking parameter \(z_0\), the z-coordinate of the point-of-closest-approach (POCA).
Note
Tracking parameters are with respect to the origin (0,0,0). For the POCA with respect to the measured beam interaction point, see
dz
(you probably want this unless you’re doing a tracking study or some debugging) andz0FromIP
.Returns NaN if called for something other than a track-based particle.
- Unit:
cm
- z0Err#
Returns the uncertainty on \(z_0\), the z-coordinate of the point-of-closest-approach (POCA).
Returns NaN if called for something other than a track-based particle.”
- Unit:
cm
- z0FromIP#
Returns the tracking parameter \(z_0\), the z-coordinate of the point-of-closest-approach (POCA), with respect to the measured beam interaction point.
Returns NaN if called for something other than a track-based particle.
- Unit:
cm
- z0Pull#
The pull of the tracking parameter \(z_0\) for the reconstructed pattern-recognition track, with respect to the MC track. That is:
\[\frac{z_0^\textrm{MC} - z_0^\textrm{PR}}{\sigma_{z_0; \textrm{PR}}}\]Returns NaN if no MC particle is related or if called on something other than a track-based particle.
V0 Tracking#
Here is a list of track variables for V0 daughters:
- V0Deltad0#
Return the difference between d0 impact parameters of V0’s daughters with the V0 vertex point as a pivot for the track.
- Unit:
cm
- V0Deltaz0#
Return the difference between z0 impact parameters of V0’s daughters with the V0 vertex point as a pivot for the track.
- Unit:
cm
- V0d0(id)#
Return the d0 impact parameter of a V0’s daughter with daughterID index with the V0 vertex point as a pivot for the track.
- Unit:
cm
- V0z0(id)#
Return the z0 impact parameter of a V0’s daughter with daughterID index with the V0 vertex point as a pivot for the track.
- Unit:
cm
- convertedPhotonDelR(i, j)#
Discriminating variable Delta-R calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- convertedPhotonDelTanLambda(i, j)#
Discriminating variable Delta-TanLambda calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- convertedPhotonDelZ(i, j)#
Discriminating variable Delta-Z calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- convertedPhotonInvariantMass(i, j)#
Invariant mass of the i-j daughter system as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
GeV/\(\text{c}^2\)
- convertedPhotonPx(i, j)#
Estimate of x-component of photon momentum calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
GeV/c
- convertedPhotonPy(i, j)#
Estimate of y-component of photon momentum calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
GeV/c
- convertedPhotonPz(i, j)#
Estimate of z-component of photon momentum calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
GeV/c
- convertedPhotonRho(i, j)#
Estimate of vertex Rho calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- convertedPhotonX(i, j)#
Estimate of vertex X coordinate calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- convertedPhotonY(i, j)#
Estimate of vertex Y coordinate calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- convertedPhotonZ(i, j)#
Estimate of vertex Z coordinate calculated for daughters (i,j) as defined in https://indico.belle2.org/event/3644/contributions/18622/attachments/9401/14443/Photon_vertexin_B2GM.pdf, assuming it’s a converted photon
- Unit:
cm
- v0DaughterCov(i, j)#
j-th element of the 15 covariance matrix elements (at IP perigee) of the i-th daughter track. (0,0), (0,1) … (1,1), (1,2) … (2,2) …index order is: 0:d0, 1:phi0, 2:omega, 3:z0, 4:tanLambda
- Unit:
cm, rad, \(\text{cm}^{-1}\), cm, unitless
- v0DaughterD0(i)#
d0 of the i-th daughter track fit
- Unit:
cm
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
d0
, so replace the current call withdaughter(i, d0)
.
- v0DaughterD0Error(i)#
d0 error of the i-th daughter track fit
- Unit:
cm
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
d0Err
, so replace the current call withdaughter(i, d0Err)
.
- v0DaughterD0PullWithOriginAsPivot(i)#
d0 pull of the i-th daughter track with the origin as the track pivot
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
d0Pull
, so replace the current call withdaughter(i, d0Pull)
.
- v0DaughterD0PullWithTrueVertexAsPivot(i)#
d0 pull of the i-th daughter track with the true V0 vertex as the track pivot
- v0DaughterFirstCDCLayer(i)#
First activated CDC layer associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
firstCDCLayer
, so replace the current call withdaughter(i, firstCDCLayer)
.
- v0DaughterFirstPXDLayer(i)#
First activated PXD layer associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
firstPXDLayer
, so replace the current call withdaughter(i, firstPXDLayer)
.
- v0DaughterFirstSVDLayer(i)#
First activated SVD layer associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
firstSVDLayer
, so replace the current call withdaughter(i, firstSVDLayer)
.
- v0DaughterLastCDCLayer(i)#
Last CDC layer associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
lastCDCLayer
, so replace the current call withdaughter(i, lastCDCLayer)
.
- v0DaughterNCDCHits(i)#
Number of CDC hits associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
nCDCHits
, so replace the current call withdaughter(i, nCDCHits)
.
- v0DaughterNPXDHits(i)#
Number of PXD hits associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
nPXDHits
, so replace the current call withdaughter(i, nPXDHits)
.
- v0DaughterNRemovedHits(i)#
The number of the i-th daughter track hits removed in V0Finder. Returns 0 if called for something other than V0 daughters.
- v0DaughterNSVDHits(i)#
Number of SVD hits associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
nSVDHits
, so replace the current call withdaughter(i, nSVDHits)
.
- v0DaughterNVXDHits(i)#
Number of PXD+SVD hits associated to the i-th daughter track
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
nVXDHits
, so replace the current call withdaughter(i, nVXDHits)
.
- v0DaughterOmega(i)#
omega of the i-th daughter track fit
- Unit:
\(\text{cm}^{-1}\)
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
omega
, so replace the current call withdaughter(i, omega)
.
- v0DaughterOmegaError(i)#
omega error of the i-th daughter track fit
- Unit:
\(\text{cm}^{-1}\)
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
omegaErr
, so replace the current call withdaughter(i, omegaErr)
.
- v0DaughterOmegaPullWithOriginAsPivot(i)#
omega pull of the i-th daughter track with the origin as the track pivot
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
omegaPull
, so replace the current call withdaughter(i, omegaPull)
.
- v0DaughterOmegaPullWithTrueVertexAsPivot(i)#
omega pull of the i-th daughter track with the true V0 vertex as the track pivot
- v0DaughterPValue(i)#
chi2 probalility of the i-th daughter track fit
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
pValue
, so replace the current call withdaughter(i, pValue)
.
- v0DaughterPhi0(i)#
phi0 of the i-th daughter track fit
- Unit:
rad
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
phi0
, so replace the current call withdaughter(i, phi0)
.
- v0DaughterPhi0Error(i)#
phi0 error of the i-th daughter track fit
- Unit:
rad
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
phi0Err
, so replace the current call withdaughter(i, phi0Err)
.
- v0DaughterPhi0PullWithOriginAsPivot(i)#
phi0 pull of the i-th daughter track with the origin as the track pivot
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
phi0Pull
, so replace the current call withdaughter(i, phi0Pull)
.
- v0DaughterPhi0PullWithTrueVertexAsPivot(i)#
phi0 pull of the i-th daughter track with the true V0 vertex as the track pivot
- v0DaughterTanLambda(i)#
tan(lambda) of the i-th daughter track fit
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
tanLambda
, so replace the current call withdaughter(i, tanLambda)
.
- v0DaughterTanLambdaError(i)#
tan(lambda) error of the i-th daughter track fit
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
tanLambdaErr
, so replace the current call withdaughter(i, tanLambdaErr)
.
- v0DaughterTanLambdaPullWithOriginAsPivot(i)#
tan(lambda) pull of the i-th daughter track with the origin as the track pivot
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
tanLambdaPull
, so replace the current call withdaughter(i, tanLambdaPull)
.
- v0DaughterTanLambdaPullWithTrueVertexAsPivot(i)#
tan(lambda) pull of the i-th daughter track with the true V0 vertex as the track pivot
- v0DaughterTau(i, j)#
j-th track parameter (at IP perigee) of the i-th daughter track. j: 0:d0, 1:phi0, 2:omega, 3:z0, 4:tanLambda
- Unit:
cm, rad, \(\text{cm}^{-1}\), cm, unitless
- v0DaughterZ0(i)#
z0 of the i-th daughter track fit
- Unit:
cm
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
z0
, so replace the current call withdaughter(i, z0)
.
- v0DaughterZ0Error(i)#
z0 error of the i-th daughter track fit
- Unit:
cm
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
z0Err
, so replace the current call withdaughter(i, z0Err)
.
- v0DaughterZ0PullWithOriginAsPivot(i)#
z0 pull of the i-th daughter track with the origin as the track pivot
Note
Deprecated since version light-2104-poseidon.
The same value can be calculated with the more generic variable
z0Pull
, so replace the current call withdaughter(i, z0Pull)
.
- v0DaughterZ0PullWithTrueVertexAsPivot(i)#
z0 pull of the i-th daughter track with the true V0 vertex as the track pivot
flag for V0 daughters sharing the first(innermost) VXD hit. 0x1(0x2) bit represents V/z(U/r-phi)-hit share.
flag for V0 daughters sharing the first(innermost) VXD U-side hit.
flag for V0 daughters sharing the first(innermost) VXD V-side hit.
PID#
Here is a list of particle identification variables:
Warning
The definitions of the default PID variables have changed between release-01 and release-02.
Prior to release-02-00-00 (i.e. in release-01-XX-YY) each ID was calculated against the pion likelihood alone, or the kaon in the case of the pion itself. Namely the pair probability (also known as the binary probability) was returned:
for all particles: \(\text{<Part>ID}=\mathcal{L}_{\text{<Part>}}/\mathcal{L}_\pi\), where \(\text{<Part>}\in[e,\mu,K,p,d]\).
for pions: \(\text{PionID}=\mathcal{L}_\pi/\mathcal{L}_K\).
In other words, pionID was sensitive only to the pion-kaon mis-id, and not to the pion-proton or pion-muon mis-identification.
- binaryElectronID_noSVD_noTOP(pdgCodeTest)#
(SPECIAL (TEMP) variable) Returns the binary probability for the electron mass hypothesis with respect to another mass hypothesis using all detector components, excluding the SVD and the TOP. NB: this variable must be used in place of
binaryPID
(pdgCode1=11
) when analysing data (MC) processed (simulated) in release 5
- binaryElectronID_noTOP(pdgCodeTest)#
(SPECIAL (TEMP) variable) Returns the binary probability for the electron mass hypothesis with respect to another mass hypothesis using all detector components, excluding the TOP. NB: this variable must be used in place of
binaryPID
(pdgCode1=11
) when analysing data (MC) processed (simulated) in release 6
- binaryPID(pdgCode1, pdgCode2)#
Returns the binary probability for the first provided mass hypothesis with respect to the second mass hypothesis using all detector components
- binaryPID_noARICHwoECL(pdgCode1, pdgCode2)#
Returns the binary probability for the first provided mass hypothesis with respect to the second mass hypothesis using all detector components, but ARICH info excluded for tracks without associated ECL cluster
- binaryPID_noSVD(pdgCode1, pdgCode2)#
Returns the binary probability for the first provided mass hypothesis with respect to the second mass hypothesis using all detector components, excluding the SVD.
- deuteronID#
deuteron identification probability defined as \(\mathcal{L}_d/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- deuteronID_noSVD#
(SPECIAL (TEMP) variable) deuteron identification probability defined as \(\mathcal{L}_d/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- electronID#
electron identification probability defined as \(\mathcal{L}_e/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- electronID_noSVD#
(SPECIAL (TEMP) variable) electron identification probability defined as \(\mathcal{L}_e/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- electronID_noSVD_noTOP#
(SPECIAL (TEMP) variable) electron identification probability defined as \(\mathcal{L}_e/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD and the TOP. NB: this variable must be used in place of
electronID
when analysing data (MC) processed (simulated) in release 5
- electronID_noTOP#
(SPECIAL (TEMP) variable) electron identification probability defined as \(\mathcal{L}_e/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the TOP. NB: this variable must be used in place of
electronID
when analysing data (MC) processed (simulated) in release 6
- isPIDAvailable#
True if PID is available (for at least one of the PID detectors
- isPIDAvailableFrom(detectorList)#
True if PID is available for at least one of the detectors in the list)
- kaonID#
kaon identification probability defined as \(\mathcal{L}_K/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- kaonIDNN#
kaon identification probability as calculated from the PID neural network.
- kaonID_noARICHwoECL#
(SPECIAL (TEMP) variable) kaon identification probability defined as \(\mathcal{L}_K/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors but ARICH info excluded for tracks without associated ECL cluster
- kaonID_noSVD#
(SPECIAL (TEMP) variable) kaon identification probability defined as \(\mathcal{L}_K/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- muonID#
muon identification probability defined as \(\mathcal{L}_\mu/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- muonID_noSVD#
(SPECIAL (TEMP) variable) muon identification probability defined as \(\mathcal{L}_\mu/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- nbarID#
Returns MVA classifier for antineutron PID.
1 signal(antineutron) like
0 background like
-1 invalid using this PID due to some ECL variables used unavailable
This PID is only for antineutron. Neutron is also considered as background. The variables used are
clusterPulseShapeDiscriminationMVA
,clusterE
,clusterLAT
,clusterE1E9
,clusterE9E21
,clusterAbsZernikeMoment40
,clusterAbsZernikeMoment51
,clusterZernikeMVA
.
- particleID#
the particle identification probability under the particle’s own hypothesis, using info from all available detectors
- pidChargedBDTScore(pdgCodeHyp, detector)#
Returns the charged Pid BDT score for a certain mass hypothesis with respect to all other charged stable particle hypotheses. The second argument specifies which BDT training to use: based on ‘ALL’ PID detectors (NB: ‘SVD’ is currently excluded), or ‘ECL’ only. The choice depends on the ChargedPidMVAMulticlassModule’s configuration.
- pidPairChargedBDTScore(pdgCodeHyp, pdgCodeTest, detector)#
Returns the charged Pid BDT score for a certain mass hypothesis with respect to an alternative hypothesis. The second argument specifies which BDT training to use: based on ‘ALL’ PID detectors (NB: ‘SVD’ is currently excluded), or ‘ECL’ only. The choice depends on the ChargedPidMVAModule’s configuration.
- pionID#
pion identification probability defined as \(\mathcal{L}_\pi/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- pionIDNN#
pion identification probability as calculated from the PID neural network.
- pionID_noARICHwoECL#
(SPECIAL (TEMP) variable) pion identification probability defined as \(\mathcal{L}_\pi/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors but ARICH info excluded for tracks without associated ECL cluster
- pionID_noSVD#
(SPECIAL (TEMP) variable) pion identification probability defined as \(\mathcal{L}_\pi/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- protonID#
proton identification probability defined as \(\mathcal{L}_p/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors
- protonID_noSVD#
(SPECIAL (TEMP) variable) proton identification probability defined as \(\mathcal{L}_p/(\mathcal{L}_e+\mathcal{L}_\mu+\mathcal{L}_\pi+\mathcal{L}_K+\mathcal{L}_p+\mathcal{L}_d)\), using info from all available detectors excluding the SVD
- weightedDeuteronID(weightMatrixName)#
weighted deuteron identification probability defined as \(\frac{\mathcal{\tilde{L}}_d}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
- weightedElectronID(weightMatrixName)#
weighted electron identification probability defined as \(\frac{\mathcal{\tilde{L}}_e}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
- weightedKaonID(weightMatrixName)#
weighted kaon identification probability defined as \(\frac{\mathcal{\tilde{L}}_K}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
- weightedMuonID(weightMatrixName)#
weighted muon identification probability defined as \(\frac{\mathcal{\tilde{L}}_\mu}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
- weightedPionID(weightMatrixName)#
weighted pion identification probability defined as \(\frac{\mathcal{\tilde{L}}_\pi}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
- weightedProtonID(weightMatrixName)#
weighted proton identification probability defined as \(\frac{\mathcal{\tilde{L}}_p}{\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i}\), where \(\mathcal{\tilde{L}}_i\) is defined as \(\log\mathcal{\tilde{L}}_i = \sum_{j={\mathrm{SVD, CDC, TOP, ARICH, ECL, KLM}}} \mathcal{w}_{ij}\log\mathcal{L}_{ij}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector. One can provide the name of the weight matrix as the argument.
Basic particle information#
- charge#
Returns electric charge of particle in units of \(e\).
- chiProb#
A context-dependent \(\chi^2\) probability for ‘the fit’ related to this particle.
If this particle is track-based, then this is the pvalue of the track fit (identical to
pValue
).If this particle is cluster-based then this variable is currently unused.
If this particle is composite and a vertex fit has been performed, then this is the \(\chi^2\) probability of the vertex fit result.
Tip
If multiple vertex fits are performed then the last one sets the
chiProb
overwriting all previous.See also
pValue
for tracks
- flavor#
Returns 1 if particle is flavored type, 0 if it is unflavored.
- isFromECL#
Returns true if this particle was created from an ECLCluster, false otherwise.
- isFromKLM#
Returns true if this particle was created from a KLMCluster, false otherwise.
- isFromTrack#
Returns true if this particle was created from a track, false otherwise.
- isFromV0#
Returns true if this particle was created from a V0, false otherwise.
- isUnspecified#
Returns true if the particle is marked as an unspecified object (like B0 -> @Xsd e+ e-), false otherwise
- mdstIndex#
Store array index (0 - based) of the MDST object from which the Particle was created. It’s 0 for composite particles.
Tip
It is not a unique identifier of particle. For example, a pion and a gamma can have the same
mdstIndex
: pions are created from tracks whereas gammas are created from ECL clusters; tracks and ECL clusters are stored in different arrays. A photon may be created from ECL cluster with index 0 and a pion may be created from track with index 0 will both havemdstIndex
equal to 0, but they will be different particles.Tip
Two particles of the same type can also have the same
mdstIndex
. This would mean that they are created from the same object. For example, if pion and kaon have the samemdstIndex
it means that they are created from the same track.Tip
If you are looking for unique identifier of the particle, please use
uniqueParticleIdentifier
.
- nDaughters#
Returns number of daughter particles
- particleSource#
Returns mdst source used to create the particle. The meaning of the values are
0: undefined
1: created from track
2: created from ECL cluster
3: created from KLM cluster
4: created from V0
5: MC particle
6: composite particle
- uniqueParticleIdentifier#
Returns unique identifier of final state particle. Particles created from the same object (e.g. from the same track) have different
uniqueParticleIdentifier
value.
PID for expert#
These expert-level variables are metavariable that allow the used to access the LogLikelihood values, the binary likelihood ratios and the global likelihood ratios for any arbitrary detector combination of mass hypothesis. The accepted detector codes are SVD, TOP, CDC, ARICH, ECL, KLM and ALL.
If a likelihood is not available from the selected detector list, NaN is returned.
Warning
These variables are not to be used in physics analyses, but only by experts doing performance studies.
- klmMuonIDDNN#
Muon probability calculated from Neural Network with KLM information (expert use only)
- pidDeltaLogLikelihoodValueExpert(pdgCode1, pdgCode2, detectorList)#
returns LogL(hyp1) - LogL(hyp2) (aka DLL) for two mass hypotheses and a set of detectors.
- pidIsMostLikely(ePrior=1/6, muPrior=1/6, piPrior=1/6, KPrior=1/6, pPrior=1/6, dPrior=1/6)#
Returns True if the largest PID likelihood of a given particle corresponds to its particle hypothesis. This function accepts either no arguments, or 6 floats as priors for the charged particle hypotheses following the order shown in the metavariable’s declaration. Flat priors are assumed as default.
- pidLogLikelihoodValueExpert(pdgCode, detectorList)#
returns the log likelihood value of for a specific mass hypothesis and set of detectors.
- pidLogarithmicProbabilityExpert(pdgCodeHyp, detectorList)#
logarithmic equivalent of pidProbability (p) defined as log(p/(1-p)), which gives a smooth peak-like distribution
- pidMissingProbabilityExpert(detectorList)#
returns 1 if PID is missing for at least one of the detectors in the list, otherwise 0.
- pidMostLikelyPDG(ePrior=1/6, muPrior=1/6, piPrior=1/6, KPrior=1/6, pPrior=1/6, dPrior=1/6)#
Returns PDG code of the largest PID likelihood, or NaN if PID information is not available. This function accepts either no arguments, or 6 floats as priors for the charged particle hypotheses following the order shown in the metavariable’s declaration. Flat priors are assumed as default.
- pidNeuralNetworkValueExpert(pdgCodeHyp, PIDNeuralNetworkName)#
Probability for the particle hypothesis pdgCodeHype calculated from a neural network, which uses high-level information as inputs, such as the likelihood from the 6 subdetectors for PID for all 6 hypotheses, \(\mathcal{\tilde{L}}_{hyp}^{det}\), or the track momentum and charge
- pidPairProbabilityExpert(pdgCodeHyp, pdgCodeTest, detectorList)#
Pair (or binary) probability for the pdgCodeHyp mass hypothesis respect to the pdgCodeTest one, using an arbitrary set of detectors. \(\mathcal{L}_{hyp}/(\mathcal{L}_{test}+\mathcal{L}_{hyp})\)
- pidProbabilityExpert(pdgCodeHyp, detectorList)#
probability for the pdgCodeHyp mass hypothesis respect to all the other ones, using an arbitrary set of detectors \(\mathcal{L}_{hyp}/(\Sigma_{\text{all~hyp}}\mathcal{L}_{i})\).
- pidWeightedLogLikelihoodValueExpert(weightMatrixName, pdgCode, detectorList)#
returns the weighted log likelihood value of for a specific mass hypothesis and set of detectors, \(\log\mathcal{\tilde{L}}_{hyp} = \sum_{j\in\mathrm{detectorList}} \mathcal{w}_{hyp,j}\log\mathcal{L}_{hyp,j}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector.
- pidWeightedPairProbabilityExpert(weightMatrixName, pdgCodeHyp, pdgCodeTest, detectorList)#
Weighted pair (or binary) probability for the pdgCodeHyp mass hypothesis with respect to the pdgCodeTest one, using an arbitrary set of detectors, \(\mathcal{\tilde{L}}_{hyp}/(\mathcal{\tilde{L}}_{test}+\mathcal{\tilde{L}}_{hyp})\) where \(\mathcal{\tilde{L}}_{i}\) is defined as \(\log\mathcal{\tilde{L}}_{i} = \sum_{j\in\mathrm{detectorList}} \mathcal{w}_{i,j}\log\mathcal{L}_{i,j}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector.
- pidWeightedProbabilityExpert(weightMatrixName, pdgCodeHyp, detectorList)#
Weighted probability for the pdgCodeHyp mass hypothesis with respect to all the other ones, using an arbitrary set of detectors, \(\mathcal{\tilde{L}}_{hyp}/\sum_{i=e,\mu,\pi,K,p,d} \mathcal{\tilde{L}}_i\) where \(\mathcal{\tilde{L}}_{i}\) is defined as \(\log\mathcal{\tilde{L}}_{i} = \sum_{j\in\mathrm{detectorList}} \mathcal{w}_{i,j}\log\mathcal{L}_{i,j}\). The \(\mathcal{L}_{ij}\) is the original likelihood and \(\mathcal{w}_{ij}\) is the PID calibration weight of i-th particle type and j-th detector.
ECL Cluster#
Here is a list of variables related to ECL cluster. All ECLCluster-based variables return NaN if no ECLCluster is found.
Note
All floating type variables in the mdst dataobject ECLCluster use ROOT Double32_t types with specific range declaration to save disk storage. This has two important consequences for a user:
All ECL cluster variables have a limited precision. This precision is always better than the intrinsic ECL data acquisition precision. However, if these variables are histogrammed, binning effects are likely.
All ECL cluster variables are clipped at the lower and upper boundaries: Values below (above) these boundaries will be set to the lower (upper) bound.
Lower and upper limits, and precision of these variables are mentioned inside the note box below them. One should note this in the context of binning effects.
- beamBackgroundSuppression#
Returns the output of an MVA classifier that uses shower-related variables to distinguish true photon clusters from beam background clusters. Class 1 is for true photon clusters while class 0 is for beam background clusters.
The MVA has been trained using MC and the features used are:
Both run-dependent and run-independent weights are available. For more information on this, and for usage recommendations, please see the Neutrals Performance XWiki Page.
- clusterAbsZernikeMoment40#
Returns absolute value of Zernike moment 40 (\(|Z_{40}|\)). (shower shape variable).
Note
- clusterAbsZernikeMoment51#
Returns absolute value of Zernike moment 51 (\(|Z_{51}|\)). (shower shape variable).
Note
- clusterCellID#
Returns cellId of the crystal with highest energy in the ECLCluster.
- clusterClusterID#
Returns ECL cluster ID of this ECL cluster within the connected region (CR) to which it belongs to.
- clusterConnectedRegionID#
Returns ECL cluster’s connected region ID.
- clusterDeltaLTemp#
- Returns DeltaL for the shower shape.A cluster comprises the energy depositions of several crystals. All these crystals have slightly different orientations in space. A shower direction can be constructed by calculating the weighted average of these orientations using the corresponding energy depositions as weights. The intersection (more precisely the point of closest approach) of the vector with this direction originating from the cluster center and an extrapolated track can be used as reference for the calculation of the shower depth. It is defined as the distance between this intersection and the cluster center.
Warning
This distance is calculated on the reconstructed level and is temporarily included to the ECL cluster MDST data format for studying purposes. If it is found that it is not crucial for physics analysis then this variable will be removed in future releases. Therefore, keep in mind that this variable might be removed in the future!
Note
- Unit:
cm
- clusterE#
Returns ECL cluster’s energy corrected for leakage and background.
The raw photon energy is given by the weighted sum of all ECL crystal energies within the ECL cluster. The weights per crystals are \(\leq 1\) after cluster energy splitting in the case of overlapping clusters. The number of crystals that are included in the sum depends on a initial energy estimation and local beam background levels at the highest energy crystal position. It is optimized to minimize the core width (resolution) of true photons. Photon energy distributions always show a low energy tail due to unavoidable longitudinal and transverse leakage that can be further modified by the clustering algorithm and beam backgrounds.The peak position of the photon energy distributions are corrected to match the true photon energy in MC:
Leakage correction: Using large MC samples of mono-energetic single photons, a correction factor \(f\) as function of reconstructed detector position, reconstructed photon energy and beam backgrounds is determined via \(f = \frac{\text{peak_reconstructed}}{\text{energy_true}}\).
Cluster energy calibration (data only): To reach the target precision of \(< 1.8\%\) energy resolution for high energetic photons, the remaining difference between MC and data must be calibrated using kinematically fit muon pairs. This calibration is only applied to data and not to MC and will take time to develop.
Energy Bias Correction module, sub-percent correction, is NOT applied on clusterE, but on photon energy and momentum. Only applied to data.
It is important to note that after perfect leakage correction and cluster energy calibration, the \(\pi^{0}\) mass peak will be shifted slightly to smaller values than the PDG average due to the low energy tails of photons. The \(\pi^{0}\) mass peak must not be corrected to the PDG value by adjusting the reconstructed photon energies. Selection criteria based on the mass for \(\pi^{0}\) candidates must be based on the biased value. Most analysis will used mass constrained \(\pi^{0}\) s anyhow.
Warning
We only store clusters with \(E > 20\,\) MeV.
Note
Please read this first.Lower limit: \(-5\) (\(e^{-5} = 0.00674\,\) GeV)Upper limit: \(3.0\) (\(e^3 = 20.08553\,\) GeV)Precision: \(18\) bitThis value can be changed to a different reference frame withuseCMSFrame
.- Unit:
GeV
- clusterE1E9#
Returns ratio of energies of the central crystal, E1, and 3x3 crystals, E9, around the central crystal. Since \(E1 \leq E9\), this ratio is \(\leq 1\) and tends towards larger values for photons and smaller values for hadrons.
Note
- clusterE9E21#
Returns ratio of energies in inner 3x3 crystals, E9, and 5x5 crystals around the central crystal without corners. Since \(E9 \leq E21\), this ratio is \(\leq 1\) and tends towards larger values for photons and smaller values for hadrons.
Note
- clusterE9E25#
Deprecated - kept for backwards compatibility - returns clusterE9E21.
- clusterEoP#
Returns ratio of uncorrelated energy E over momentum p, a convenience alias for (clusterE / p).
- clusterErrorE#
Returns ECL cluster’s uncertainty on energy (from background level and energy dependent tabulation).
- Unit:
GeV
- clusterErrorPhi#
Returns ECL cluster’s uncertainty on \(\phi\) (from background level and energy dependent tabulation).
- Unit:
rad
- clusterErrorTheta#
Returns ECL cluster’s uncertainty on \(\theta\) (from background level and energy dependent tabulation).
- Unit:
rad
- clusterErrorTiming#
Returns ECL cluster’s timing uncertainty that contains \(99\%\) of true photons (dt99).
The photon timing uncertainty is currently determined using MC. The resulting parametrization depends on the true energy deposition in the highest energetic crystal and the local beam background level in that crystal. The resulting timing distribution is non-Gaussian and for each photon the value dt99 is stored, where \(|\text{timing}| / \text{dt99} < 1\) is designed to give a \(99\%\) timing efficiency for true photons from the IP. The resulting efficiency is approximately flat in energy and independent of beam background levels.
Very large values of dt99 are an indication of failed waveform fits in the ECL. We remove such clusters in most physics photon lists.
Note
Warning
In real data there will be a sizeable number of high energetic Bhabha events (from previous or later bunch collisions) that can easily be rejected by timing cuts. However, these events create large ECL clusters that can overlap with other ECL clusters and it is not clear that a simple rejection is the correction strategy.
- Unit:
ns
- clusterHasFailedErrorTiming#
Status bit for if the ECL cluster’s timing uncertainty calculation failed. Photon timing is given by the fitted time of the recorded waveform of the highest energetic crystal in a cluster; however, that fit can fail and so this variable tells the user if that has happened.
- clusterHasFailedTiming#
Status bit for if the ECL cluster’s timing fit failed. Photon timing is given by the fitted time of the recorded waveform of the highest energetic crystal in a cluster; however, that fit can fail and so this variable tells the user if that has happened.
- clusterHasNPhotons#
Returns 1.0 if cluster has the ‘N photons’ hypothesis (historically called ‘N1’), 0.0 if not, and NaN if no cluster is associated to the particle.
- clusterHasNeutralHadron#
Returns 1.0 if the cluster has the ‘neutral hadrons’ hypothesis (historically called ‘N2’), 0.0 if not, and NaN if no cluster is associated to the particle.
- clusterHasPulseShapeDiscrimination#
Status bit to indicate if cluster has digits with waveforms that passed energy and \(\chi^2\) thresholds for computing PSD variables.
- clusterHighestE#
Returns energy of the highest energetic crystal in the ECL cluster after reweighting.
Warning
This variable must be used carefully since it can bias shower selection towards photons that hit crystals in the center and hence have a large energy deposition in the highest energy crystal.
Note
Please read this first.Lower limit: \(-5\) (\(e^{-5} = 0.00674\,\) GeV)Upper limit: \(3.0\) (\(e^3 = 20.08553\,\) GeV)Precision: \(18\) bit- Unit:
GeV
- clusterKlId#
Returns MVA classifier that uses ECL clusters variables to discriminate Klong clusters from em background.
1 for Kl
0 for background
- clusterLAT#
Returns lateral energy distribution (shower variable). It is defined as following:
\[S = \frac{\sum_{i=2}^{n} w_{i} E_{i} r^2_{i}}{(w_{0} E_{0} + w_{1} E_{1}) r^2_{0} + \sum_{i=2}^{n} w_{i} E_{i} r^2_{i}}\]where \(E_{i} = (E_{0}, E_{1}, ...)\) are the single crystal energies sorted by energy (\(E_{0}\) is the highest energy and \(E_{1}\) the second highest), \(w_{i}\) is the crystal weight, \(r_{i}\) is the distance of the \(i\)-th digit to the shower center projected to a plane perpendicular to the shower axis, and \(r_{0} \approx 5\,cm\) is the distance between two crystals.
clusterLAT peaks around 0.3 for radially symmetrical electromagnetic showers and is larger for hadronic events, and electrons with a close-by radiative or Bremsstrahlung photon.
Note
- clusterMdstIndex#
StoreArray index(0 - based) of the MDST ECLCluster (useful for track-based particles matched to a cluster).
- clusterNHits#
Returns sum of weights \(w_{i}\) (\(w_{i} \leq 1\)) of all crystals in an ECL cluster. For non-overlapping clusters this is equal to the number of crystals in the cluster. In case of energy splitting among nearby clusters, this can be a non-integer value.
Note
Please read this first.Lower limit: \(0.0\)Upper limit: \(200.0\)Precision: \(10\) bitIf fractional weights are not of interest, this value should be cast to the nearest integer.
- clusterNumberOfHadronDigits#
Returns ECL cluster’s number of hadron digits in cluster (pulse shape discrimination variable). Weighted sum of digits in cluster with significant scintillation emission (\(> 3\,\) MeV) in the hadronic scintillation component. Computed only using cluster digits with energy \(> 50\,\) MeV and good offline waveform fit \(\chi^2\).
Note
- clusterPhi#
Returns ECL cluster’s azimuthal angle \(\phi\) (this is not generally equal to a photon azimuthal angle).
The direction of a cluster is given by the connecting line of \(\,(0,0,0)\,\) and cluster centroid position in the ECL.The cluster centroid position is calculated using up to 21 crystals (5x5 excluding corners) after cluster energy splitting in the case of overlapping clusters.The centroid position is the logarithmically weighted average of all crystals evaluated at the crystal centers. Cluster centroids are generally biased towards the centers of the highest energetic crystal. This effect is larger for low energetic photons.Beam backgrounds slightly decrease the position resolution, mainly for low energetic photons.Note
Radius of a cluster is almost constant in the barrel and should not be used directly in any selection.
Unlike for charged tracks, the uncertainty (covariance) of the photon directions is not determined based on individual cluster properties but taken from on MC-based parametrizations of the resolution as function of true photon energy, true photon direction and beam background level.
Warning
Users must use the actual particle direction (done automatically in the modularAnalysis using the average IP position (can be changed if needed)) and not the ECL Cluster direction (position in the ECL measured from \((0,0,0)\)) for particle kinematics.
Note
- Unit:
rad
- clusterPhiID#
Returns phiId of the crystal with highest energy in the ECLCluster.
- clusterPulseShapeDiscriminationMVA#
Returns MVA classifier that uses pulse shape discrimination to identify electromagnetic vs hadronic showers.
1 for electromagnetic showers
0 for hadronic showers
- clusterR#
Returns ECL cluster’s centroid distance from \((0,0,0)\).
- Unit:
cm
- clusterReg#
Returns an integer code for the ECL region of a cluster.
1: forward, 2: barrel, 3: backward,
11: between FWD and barrel, 13: between BWD and barrel,
0: otherwise
- clusterSecondMoment#
Returns second moment \(S\). It is defined as:
\[S = \frac{1}{S_{0}(\theta)}\frac{\sum_{i=0}^{n} w_{i} E_{i} r^2_{i}}{\sum_{i=0}^{n} w_{i} E_{i}}\]where \(E_{i} = (E_0, E_1, ...)\) are the single crystal energies sorted by energy, \(w_{i}\) is the crystal weight, and \(r_{i}\) is the distance of the \(i\)-th digit to the shower center projected to a plane perpendicular to the shower axis. \(S_{0}(\theta)\) normalizes \(S\) to 1 for isolated photons.
Note
- Unit:
dimensionless
- clusterTheta#
Returns ECL cluster’s polar angle \(\theta\) (this is not generally equal to a photon polar angle).
The direction of a cluster is given by the connecting line of \(\,(0,0,0)\,\) and cluster centroid position in the ECL.The cluster centroid position is calculated using up to 21 crystals (5x5 excluding corners) after cluster energy splitting in the case of overlapping clusters.The centroid position is the logarithmically weighted average of all crystals evaluated at the crystal centers. Cluster centroids are generally biased towards the centers of the highest energetic crystal. This effect is larger for low energetic photons.Beam backgrounds slightly decrease the position resolution, mainly for low energetic photons.Note
Radius of a cluster is almost constant in the barrel and should not be used directly in any selection.
Unlike for charged tracks, the uncertainty (covariance) of the photon directions is not determined based on individual cluster properties but taken from on MC-based parametrizations of the resolution as function of true photon energy, true photon direction and beam background level.
Warning
Users must use the actual particle direction (done automatically in the modularAnalysis using the average IP position (can be changed if needed)) and not the ECL Cluster direction (position in the ECL measured from \((0,0,0)\)) for particle kinematics.
Note
- Unit:
rad
- clusterThetaID#
Returns thetaId of the crystal with highest energy in the ECLCluster.
- clusterTiming#
In Belle II: Returns the time of the ECL cluster. It is calculated as the Photon timing minus the Event t0. Photon timing is given by the fitted time of the recorded waveform of the highest energy crystal in the cluster. After all calibrations and corrections (including Time-Of-Flight), photons from the interaction point (IP) should have a Photon timing that corresponds to the Event t0, \(t_{0}\). The Event t0 is the time of the event and may be measured by a different sub-detector (see Event t0 documentation). For an ECL cluster produced at the interaction point in time with the event, the cluster time should be consistent with zero within the uncertainties. Special values are returned if the fit for the Photon timing fails (see documentation for
clusterHasFailedTiming
). (For MC, the calibrations and corrections are not fully simulated).Note
In Belle: Returns the trigger cell (TC) time of the ECL cluster (photon). This information is available only in Belle data since experiment 31, and not available in Belle MC. Clusters produced at the interaction point in time with the event, have TC time in the range of 9000-11000 Calculated based on the Appendix of Belle note 831.
Note
In case this variable is obtained from Belle data that is stored in Belle II mdst/udst format, it will be truncated to:Lower limit: \(-1000.0\)Upper limit: \(1000.0\)Precision: \(12\) bit- Unit:
ns
- clusterTrackMatch#
Returns 1.0 if at least one reconstructed charged track is matched to the ECL cluster.
Every reconstructed charged track is extrapolated into the ECL. Every ECL crystal that is crossed by the track extrapolation is marked. Each ECL cluster that contains any marked crystal is matched to the track. Multiple tracks can be matched to one cluster and multiple clusters can be matched to one track. It is conceptually correct to have two tracks matched to the same cluster.
- clusterUncorrE#
[Expert] [Calibration] Returns ECL cluster’s uncorrected energy. That is, before leakage corrections. This variable should only be used for study of the ECL. Please see
clusterE
.- Unit:
GeV
- clusterZernikeMVA#
Returns output of a MVA using eleven Zernike moments of the cluster. Zernike moments are calculated per shower in a plane perpendicular to the shower direction via
\[|Z_{nm}| = \frac{n+1}{\pi} \frac{1}{\sum_{i} w_{i} E_{i}} \left|\sum_{i} R_{nm}(\rho_{i}) e^{-im\alpha_{i}} w_{i} E_{i} \right|\]where n, m are the integers, \(i\) runs over the crystals in the shower, \(E_{i}\) is the energy of the i-th crystal in the shower, \(R_{nm}\) is a polynomial of degree \(n\), \(\rho_{i}\) is the radial distance of the \(i\)-th crystal in the perpendicular plane, and \(\alpha_{i}\) is the polar angle of the \(i\)-th crystal in the perpendicular plane. As a crystal can be related to more than one shower, \(w_{i}\) is the fraction of the energy of the \(i\)-th crystal associated with the shower.
More details about the implementation can be found in BELLE2-NOTE-TE-2017-001 .
More details about Zernike polynomials can be found in Wikipedia .
For cluster with hypothesisId==N1: raw MVA output.For cluster with hypothesisId==N2: 1 - prod{clusterZernikeMVA}, where the product is on all N1 showers belonging to the same connected region (shower shape variable).Note
- distanceToMcKl#
Returns the distance to the nearest truth KL particle, extrapolated to the cluster radius. To use this variable, it is required to run getNeutralHadronGeomMatches function. Optionally, it can return negative values to indicate that the ECL cluster should be removed from the analysis to correct for data to MC difference in KL efficiency.
- Unit:
cm
- distanceToMcNeutron#
Returns the distance to the nearest truth (anti)neutron, extrapolated to the cluster radius. To use this variable, it is required to run getNeutralHadronGeomMatches function. Optionally, it can return negative values to indicate that the ECL cluster should be removed from the analysis to correct for data to MC difference in KL efficiency.
- Unit:
cm
- eclClusterOnlyInvariantMass#
[Expert] The invariant mass calculated from all ECLCluster daughters (i.e. photons) and cluster-matched tracks using the cluster 4-momenta.
Used for ECL-based dark sector physics and debugging track-cluster matching.
- Unit:
GeV/\(\text{c}^2\)
- eclExtPhi#
Returns extrapolated \(\phi\) of particle track associated to the cluster (if any). Requires module ECLTrackCalDigitMatch to be executed..
- Unit:
rad
- eclExtPhiId#
Returns extrapolated \(\phi\) ID of particle track associated to the cluster (if any). Requires module ECLTrackCalDigitMatch to be executed..
- eclExtTheta#
Returns extrapolated \(\theta\) of particle track associated to the cluster (if any). Requires module ECLTrackCalDigitMatch to be executed.
- Unit:
rad
- fakePhotonSuppression#
Returns the output of an MVA classifier that uses shower-related variables to distinguish true photon clusters from fake photon clusters (e.g. split-offs, track-cluster matching failures etc.). Class 1 is for true photon clusters while class 0 is for fake photon clusters.
The MVA has been trained using MC and the features are:
This MVA is the same as the one used for
hadronicSplitOffSuppression
but that variable should not be used as it is deprecated and does not use the new weights.Both run-dependent and run-independent weights are available. For more information on this, and for usage recommendations, please see the Neutrals Performance XWiki Page.
- hadronicSplitOffSuppression#
Returns the output of an MVA classifier that uses shower-related variables to distinguish true photon clusters from hadronic splitoff clusters. The classes are:
1 for true photon clusters
0 for hadronic splitoff clusters
The MVA has been trained using samples of signal photons and hadronic splitoff photons coming from MC. The features used are (in decreasing order of significance):
Note
Deprecated since version light-2302-genetta.
Use the variable
fakePhotonSuppression
instead which is maintained and uses the latest weight files.
- maxWeightedDistanceFromAverageECLTime#
Returns maximum weighted distance between time of the cluster of a photon and the ECL average time, amongst the clusters (neutrals) and matched clusters (charged) of daughters (of all generations) of the provided particle.
- Unit:
ns
- mdstIndexMcKl#
Returns the mdst index of the nearest truth KL, extrapolated to the cluster radius, if it is within the matching cone. To use this variable, it is required to run getNeutralHadronGeomMatches function.
- mdstIndexMcNeutron#
Returns the mdst index of the nearest truth (anti)neutron, extrapolated to the cluster radius, if it is within the matching cone. To use this variable, it is required to run getNeutralHadronGeomMatches function.
- minC2TDist#
Returns the distance between the ECL cluster and its nearest track.
For all tracks in the event, the distance between each of their extrapolated hits in the ECL and the ECL shower position is calculated, and the overall smallest distance is returned. The track array index of the track that is closest to the ECL cluster can be retrieved using
minC2TDistID
.If the calculated distance is greater than \(250.0\), the returned distance will be capped at \(250.0\). If there are no extrapolated hits found in the ECL for the event, NaN will be returned.
Note
This distance is calculated on the reconstructed level.
Note
- Unit:
cm
- minC2TDistID#
Returns the track array index of the nearest track to the ECL cluster. The nearest track is calculated using the
minC2TDist
variable.
- minC2TDistVar(variable, particleList=pi-:all)#
Returns the variable value of the nearest track to the given ECL cluster as calculated by
minC2TDist
. The first argument is the variable name, e.g.nCDCHits
, while the second (optional) argument is the particle list name which will be used to pick up the nearest track in the calculation ofminC2TDist
. The default particle list used ispi-:all
.
- nECLClusterTrackMatches#
Returns number of charged tracks matched to this cluster.
Note
Sometimes (perfectly correctly) two tracks are extrapolated into the same cluster.
For charged particles, this should return at least 1 (but sometimes 2 or more).
For neutrals, this should always return 0.
Returns NaN if there is no cluster.
- nECLLocalMaximums#
[Eventbased] Returns the number of LocalMaximums in the ECL.
- nECLLocalMaximumsBWDEndcap#
[Eventbased] Returns the number of LocalMaximums in the ECL backward endcap.
- nECLLocalMaximumsBarrel#
[Eventbased] Returns the number of LocalMaximums in the ECL barrel.
- nECLLocalMaximumsFWDEndcap#
[Eventbased] Returns the number of LocalMaximums in the ECL forward endcap.
- nECLOutOfTimeCrystals#
[Eventbased] Returns the number of crystals (ECLCalDigits) that are out of time.
- nECLOutOfTimeCrystalsBWDEndcap#
[Eventbased] Returns the number of crystals (ECLCalDigits) that are out of time in the backward endcap.
- nECLOutOfTimeCrystalsBarrel#
[Eventbased] Returns the number of crystals (ECLCalDigits) that are out of time in the barrel.
- nECLOutOfTimeCrystalsFWDEndcap#
[Eventbased] Returns the number of crystals (ECLCalDigits) that are out of time in the forward endcap.
- nECLShowers#
[Eventbased] Returns the number of ECLShowers.
- nECLShowersBWDEndcap#
[Eventbased] Returns the number of ECLShowers in the backward endcap.
- nECLShowersBarrel#
[Eventbased] Returns the number of ECLShowers in the barrel.
- nECLShowersFWDEndcap#
[Eventbased] Returns the number of ECLShowers in the forward endcap.
- nECLTriggerCells#
[Eventbased] Returns the number of ECL trigger cells above 100 MeV.
- nECLTriggerCellsBWDEndcap#
[Eventbased] Returns the number of ECL trigger cells above 100 MeV in the backward endcap.
- nECLTriggerCellsBarrel#
[Eventbased] Returns the number of ECL trigger cells above 100 MeV in the barrel.
- nECLTriggerCellsFWDEndcap#
[Eventbased] Returns the number of ECL trigger cells above 100 MeV in the forward endcap.
- nKLMMultistripHits#
[Eventbased] Returns the number of multi-strip hits in the KLM.
- nKLMMultistripHitsBWDEndcap#
[Eventbased] Returns the number of multi-strip hits in the KLM backward endcap.
- nKLMMultistripHitsBarrel#
[Eventbased] Returns the number of multi-strip hits in the KLM barrel.
- nKLMMultistripHitsFWDEndcap#
[Eventbased] Returns the number of multi-strip hits in the KLM forward endcap.
- nRejectedECLShowers#
[Eventbased] Returns the number of showers in the ECL that do not become clusters.
- nRejectedECLShowersBWDEndcap#
[Eventbased] Returns the number of showers in the ECL that do not become clusters, from the backward endcap. If the number exceeds 255 (uint8_t maximum value) the variable is set to 255.
- nRejectedECLShowersBarrel#
[Eventbased] Returns the number of showers in the ECL that do not become clusters, from the barrel. If the number exceeds 255 (uint8_t maximum value) the variable is set to 255.
- nRejectedECLShowersFWDEndcap#
[Eventbased] Returns the number of showers in the ECL that do not become clusters, from the forward endcap. If the number exceeds 255 (uint8_t maximum value) the variable is set to 255.
- photonHasOverlap(cutString, photonlistname, tracklistname)#
Returns true if the connected ECL region of the particle’s cluster is shared by another particle’s cluster. Neutral and charged cluster are considered. A cut string can be provided to ignore cluster that do not satisfy the given criteria. By default, the ParticleList
gamma:all
is used for the check of neutral ECL cluster, and the ParticleListe-:all
for the check of charged ECL cluster. However, one can customize the name of the ParticleLists via additional arguments. If no argument or only a cut string is provided andgamma:all
ore-:all
does not exist or if the variable is requested for a particle that is not a photon, NaN is returned.
- weightedAverageECLTime#
Returns ECL weighted average time of all clusters (neutrals) and matched clusters (charged) of daughters (of any generation) of the provided particle.
- Unit:
ns
There are also some special variables related to the MC matching of ECL clusters (specifically).
- clusterBestMCMatchWeight#
Returns the weight of the ECLCluster -> MCParticle relation for the relation with the largest weight.
- clusterBestMCPDG#
Returns the PDG code of the MCParticle for the ECLCluster -> MCParticle relation with the largest weight.
- clusterMCMatchWeight#
Returns the weight of the ECLCluster -> MCParticle relation for the MCParticle matched to the particle. Returns NaN if: no cluster is related to the particle, the particle is not MC matched, or if there are no mcmatches for the cluster. Returns -1 if the cluster was matched to particles, but not the match of the particle provided.
- clusterTotalMCMatchWeight#
Returns the sum of all weights of the ECLCluster -> MCParticles relations.
- clusterTotalMCMatchWeightForBestKlong#
Returns the sum of all weights of the ECLCluster -> MCParticles relations when MCParticle is the same Klong or daughter of the Klong. If multiple MC Klongs are related to the ECLCluster, returns the sum of weights for the best matched Klong.
- clusterTotalMCMatchWeightForKlong#
Returns the sum of all weights of the ECLCluster -> MCParticles relations when MCParticle is a Klong or daughter of a Klong
Acceptance#
Here is a list of variables for acceptance cuts:
- inARICHAcceptance#
Returns true if particle is within ARICH geometrical and kinematical acceptance, false otherwise. This variable is an alias for
thetaInARICHAcceptance
.
- inCDCAcceptance#
Returns true if particle is within CDC geometrical and kinematical acceptance, false otherwise. This variable is an alias for
thetaInCDCAcceptance
.
- inECLAcceptance#
Returns true if particle is within ECL geometrical and kinematical acceptance, false otherwise.”); This variable is a combination of
thetaInEECLAcceptance
,thetaInBECLAcceptance
andptInBECLAcceptance
.
- inKLMAcceptance#
Returns true if particle is within KLM geometrical and kinematical acceptance, false otherwise. This variable is a combination of
thetaInEKLMAcceptance
,thetaInBKLMAcceptance
andptInBKLMAcceptance
.
- inTOPAcceptance#
Returns true if particle is within TOP geometrical and kinematical acceptance, false otherwise. This variable is a combination of
thetaInTOPAcceptance
andptInTOPAcceptance
.
- ptInBECLAcceptance#
Returns true if particle transverse momentum \(p_t\) is within Barrel ECL acceptance, \(p_t > 0.28\) GeV, false otherwise.
- ptInBKLMAcceptance#
Returns true if particle transverse momentum \(p_t\) is within Barrel KLM acceptance, \(p_t > 0.6\) GeV, false otherwise.
- ptInTOPAcceptance#
Returns true if particle transverse momentum \(p_t\) is within TOP acceptance, \(p_t > 0.27\) GeV, false otherwise.
- thetaInARICHAcceptance#
Returns true if particle is within ARICH angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(14^\circ < \theta < 30^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInBECLAcceptance#
Returns true if particle is within Barrel ECL angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(32.2^\circ < \theta < 128.7^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInBKLMAcceptance#
Returns true if particle is within Barrel KLM angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(37^\circ < \theta < 130^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInCDCAcceptance#
Returns true if particle is within CDC angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(17^\circ < \theta < 150^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInECLAcceptance#
Checks if particle is within ECL angular acceptance. This variable checks if the particle polar angle \(\theta\) is within certain ranges. Return values and the corresponding \(\theta\) ranges are the following:
0: Outside of ECL acceptance, \(\theta < 12.4^\circ\) or \(\theta > 155.1^\circ\), or in one of the acceptance gaps at \(31.4^{\circ} < \theta < 32.2^{\circ}\) or \(128.7^{\circ} < \theta < 130.7^{\circ}\);
1: Forward ECL, \(12.4^\circ < \theta < 31.4^\circ\);
2: Barrel ECL, \(32.2^\circ < \theta < 128.7^\circ\);
3: Backward ECL, \(130.7^\circ < \theta < 155.1^\circ\).
The polar angle is computed using only the initial particle momentum.
- thetaInEECLAcceptance#
Returns true if particle is within Endcap ECL angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(12.4^\circ < \theta < 31.4^\circ\) or \(130.7^\circ < \theta < 155.1^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInEKLMAcceptance#
Returns true if particle is within Endcap KLM angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(18^\circ < \theta < 47^\circ\) or \(122^\circ < \theta < 155^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInKLMAcceptance#
Checks if particle is within KLM angular acceptance. This variable checks if the particle polar angle \(\theta\) is within certain ranges. Return values and the corresponding \(\theta\) ranges are the following:
0: Outside of KLM acceptance, \(\theta < 18^\circ\) or \(\theta < 155^\circ\).
1: Forward endcap, \(18^\circ < \theta < 37^\circ\);
2: Forward overlap, \(37^\circ < \theta < 47^\circ\);
3: Barrel, \(47^\circ < \theta < 122^\circ\);
4: Backward overlap, \(122^\circ < \theta < 130^\circ\);
5: Backward endcap, \(130^\circ < \theta < 155^\circ\).
The polar angle is computed using only the initial particle momentum.
- thetaInKLMOverlapAcceptance#
Returns true if particle is within the angular region where KLM barrel and endcaps overlap, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(37^\circ < \theta < 47^\circ\) or \(122^\circ < \theta < 130^\circ\). The polar angle is computed using only the initial particle momentum.
- thetaInTOPAcceptance#
Returns true if particle is within TOP angular acceptance, false otherwise. This variable checks if the particle polar angle \(\theta\) is within the range \(31^\circ < \theta < 128^\circ\). The polar angle is computed using only the initial particle momentum.
Trigger#
Here is a list of trigger variables:
- L1FTDL(name)#
[Eventbased] Returns the FTDL (Final Trigger Decision Logic, before prescale) status (1 or 0) of the output trigger bit with the given name. Output bits are the outputs of GDL, combining different input trigger bits for final decision. For example,
ty_0/1/2/3
is one of the input trigger bits meaning the number of neuro 3D tracks is one/two/three/more than three. Whileyyy
is one of the output trigger bits meaning(ty_2 or ty_3) and !veto
. Please check on the dedicated XWiki page or the dedicated Belle II notes to find out the definition of trigger bits.
- L1FTDLBit(i)#
[Eventbased] Returns the FTDL (Final Trigger Decision Logic, before prescale) status (1 or 0) of i-th trigger bit.
Warning
It is recommended to use this variable only for debugging and to use
L1FTDL
with the explicit trigger bit name for physics analyses or performance studies.
- L1Input(name)#
[Eventbased] Returns the input bit status (1 or 0) of the trigger bit with the given name. Input trigger bits are predefined selections from each sub-detector, with adjustment of the delay and width, in order to fix latency on GDL. For example,
ty_0/1/2/3
is one of the input trigger bits meaning the number of neuro 3D tracks is one/two/three/more than three. Please check on the dedicated XWiki page or the dedicated Belle II notes to find out the definition of trigger bits.
- L1InputBit(i)#
[Eventbased] Returns the input bit status (1 or 0) of the i-th input trigger bit.
Warning
It is recommended to use this variable only for debugging and to use
L1Input
with the explicit trigger bit name for physics analyses or performance studies.
- L1PSNM(name)#
[Eventbased] Returns the PSNM (Prescale And Mask, after prescale) status (1 or 0) of the output trigger bit with the given name. For some output trigger bits, we assign a prescale factor to reduce the number of triggered events. For example, we want to keep only 1% of Bhabha events. A prescale factor of 100 is then assigned to
bha_3D
(Bhabha selected in 3D criteria). Prescale factor of a given output trigger bit could be different in different datasets. It is recommended to use prescaled trigger bits (L1PSNM) or un-prescaled trigger bits (L1FTDL) for your analysis. In run-independent MC, configuration of the prescales in TSIM (trigger simulation) can be different from data, so L1 FTDL is recommended. In run-dependent MC, configuration of the prescales in TSIM is consistent with data, so L1PSNM is recommended. Please check on the dedicated XWiki page or the dedicated Belle II notes to find out the definition of trigger bits.
- L1PSNMBit(i)#
[Eventbased] Returns the PSNM (Prescale And Mask, after prescale) status (1 or 0) of i-th trigger bit.
Warning
It is recommended to use this variable only for debugging and to use
L1PSNM
with the explicit trigger bit name for physics analyses or performance studies.
- L1PSNMBitPrescale(i)#
[Eventbased] Returns the PSNM (prescale and mask) prescale of i-th trigger bit.
Warning
It is recommended to use this variable only for debugging and to use
L1Prescale
with the explicit trigger bit name for physics analyses or performance studies.
- L1Prescale(name)#
[Eventbased] Returns the PSNM (prescale and mask) prescale factor of the trigger bit with the given name. Definition of prescale factor is shown in a few lines before in
L1PSNM
. Prescale factors are usually dependent on different datasets.
- L1TimeQuality#
[Eventbased] Returns expected Level1 timing resolution. This flag will be used for SVD 3-point sampling in future. 0:None; 1:Coarse (sigma > x ns); 2:FINE (sigma < x ns); x has been set to about 5ns before LS1 but can be changed in future
- L1TimeType#
[Eventbased] Returns kind of detector which determines the Level1 trigger timing. 0:ECL, 1:TOP, 2:SELF(timing of PSNM bit), 3:CDC, 5:delayed bhabha, 7: random, 13:poisson.
- L1Trigger#
[Eventbased] Returns 1 if at least one PSNM L1 trigger bit is true.
- isPoissonTriggerInInjectionVeto#
[Eventbased] Returns 1 if the poisson random trigger is within the injection veto window.
Tip
Please see the Trigger Bits section for further details.
- HighLevelTrigger#
[Eventbased] True if event passes the HLT trigger, false if not
- SoftwareTriggerPrescaling(triggerIdentifier)#
[Eventbased] return the prescaling for the specific software trigger identifier. Please note, this prescaling is taken from the currently setup database. It only corresponds to the correct HLT prescale if you are using the online database!If the trigger identifier is not found, returns NaN.
- SoftwareTriggerResult(triggerIdentifier)#
[Eventbased] [Expert] returns the SoftwareTriggerCutResult, defined as reject (-1), accept (1), or noResult (0). If the trigger identifier is not found, returns NaN.
For example:
SoftwareTriggerResult(filter 1_Estargt1_GeV_cluster_no_other_cluster_Estargt0.3_GeV)
which is equivalent to
SoftwareTriggerResult(software_trigger_cut&filter&1_Estargt1_GeV_cluster_no_other_cluster_Estargt0.3_GeV)
Warning
the meanings of these change depending if using trigger or the skim stage, hence expert.
See also
b2hlt_triggers
for possible triggerIdentifiers.
- SoftwareTriggerResultNonPrescaled(triggerIdentifier)#
[Eventbased] [Expert] returns the SoftwareTriggerCutResult, if this trigger would not be prescaled.Please note, this is not the final HLT decision! It is defined as reject (-1), accept (1), or noResult (0). Note that the meanings of these change depending if using trigger or the skim stage, hence expert.If the trigger identifier is not found, returns NaN.
Event#
Here is a list of event variables:
- Ecms#
[Eventbased] Returns center-of-mass energy.
- Unit:
GeV
- EcmsMC#
[Eventbased] Truth value of sqrt(s)
- Unit:
GeV
- IPCov(i, j)#
[Eventbased] Returns (i,j)-th element of the covariance matrix of the measured interaction point.
- Unit:
\(\text{cm}^2\)
- IPX#
[Eventbased] Returns x coordinate of the measured interaction point.
Note
For old data and uncalibrated MC files this will return 0.0.
Note
You might hear tracking and calibration people refer to this as the
BeamSpot
.- Unit:
cm
- IPY#
[Eventbased] Returns y coordinate of the measured interaction point.
- Unit:
cm
- IPZ#
[Eventbased] Returns z coordinate of the measured interaction point.
- Unit:
cm
- PxHER#
[Eventbased] Returns x component of the electron beam momentum in the laboratory frame.
- Unit:
GeV/c
- PxLER#
[Eventbased] Returns x component of the positron beam momentum in the laboratory frame.
- Unit:
GeV/c
- PyHER#
[Eventbased] Returns y component of the electron beam momentum in the laboratory frame.
- Unit:
GeV/c
- PyLER#
[Eventbased] Returns y component of the positron beam momentum in the laboratory frame.
- Unit:
GeV/c
- PzHER#
[Eventbased] Returns z component of the electron beam momentum in the laboratory frame.
- Unit:
GeV/c
- PzLER#
[Eventbased] Returns z component of the positron beam momentum in the laboratory frame.
- Unit:
GeV/c
- beamE#
[Eventbased] Returns total beam energy in the laboratory frame.
- Unit:
GeV
- beamPx#
[Eventbased] Returns x component of total beam momentum in the laboratory frame.
- Unit:
GeV/c
- beamPy#
[Eventbased] Returns y component of total beam momentum in the laboratory frame.
- Unit:
GeV/c
- beamPz#
[Eventbased] Returns z component of total beam momentum in the laboratory frame.
- Unit:
GeV/c
- belleECLEnergy#
[Eventbased][Legacy] Returns total energy in ECL in the event as used in Belle 1 analyses.
Warning
For Belle II use cases use either
totalEnergyOfParticlesInList(gamma:all)
, or (probably better) fill a photon list with some minimal cleanup cuts and use that instead:from variables import variables as vm fillParticleList("gamma:cleaned", "E > 0.05 and isFromECL==1", path=path) fillParticleList("e+:cleaned", "clusterE > 0.05", path=path) vm.addAlias("myNeutralECLEnergy", "totalEnergyOfParticlesInList(gamma:cleaned)") vm.addAlias("myChargedECLEnergy", "totalEnergyOfParticlesInList(e+:cleaned)") vm.addAlias("myECLEnergy", "formula(myNeutralECLEnergy+myChargedECLEnergy)")
- Unit:
GeV
- date#
[Eventbased] Returns the date when the event was recorded, a number of the form YYYYMMDD (in UTC).
See also
year
,eventTimeSeconds
,eventTimeSecondsFractionRemainder
, provided for convenience.
- eventTimeSeconds#
[Eventbased] Time of the event (truncated down) since 1970/1/1 (Unix epoch).
- Unit:
s
- eventTimeSecondsFractionRemainder#
[Eventbased] Remainder of the event time.
Tip
Use eventTimeSeconds + eventTimeSecondsFractionRemainder to get the total event time in seconds.
- Unit:
s
- evtNum#
[Eventbased] Returns the event number.
- expNum#
[Eventbased] Returns the experiment number.
- genIPX#
[Eventbased] Returns x coordinate of the interaction point used for the underlying MC generation. Returns NaN for data.
Note
This is normally smeared from 0.0
- Unit:
cm
- genIPY#
[Eventbased] Returns y coordinate of the interaction point used for the underlying MC generation. Returns NaN for data.
- Unit:
cm
- genIPZ#
[Eventbased] Returns z coordinate of the interaction point used for the underlying MC generation. Returns NaN for data.
- Unit:
cm
- hasRecentInjection#
[Eventbased] Returns 1 if an injection happened recently, 0 otherwise.
- injectionInHER#
[Eventbased] Returns 1 if injection was in HER, 0 otherwise.
- isChargedBEvent#
[Eventbased] Returns 1.0 if event contains a charged B-meson on generator level.
- isContinuumEvent#
[Eventbased] Returns 1.0 if event doesn’t contain a \(\Upsilon(4S)\) particle on generator level, 0.0 otherwise.
- isMC#
[Eventbased] Returns 1 if current basf2 process is running over simulated (Monte-Carlo) dataset and 0 in case of real experimental data.
- isNotContinuumEvent#
[Eventbased] Returns 1.0 if event does contain an \(\Upsilon(4S)\) particle on generator level and therefore is not a continuum event, 0.0 otherwise.
- isUnmixedBEvent#
[Eventbased] Returns 1.0 if the event contains opposite flavor neutral B-mesons on generator level, 0.0 in case of same flavor B-mesons and NaN if the event has no generated neutral B.
- mcPxHER#
[Eventbased] Returns truth value of the x component of the incoming electron momentum in the laboratory frame.
- Unit:
GeV/c
- mcPxLER#
[Eventbased] Returns truth value of the x component of the incoming positron momentum in the laboratory frame.
- Unit:
GeV/c
- mcPyHER#
[Eventbased] Returns truth value of the y component of the incoming electron momentum in the laboratory frame.
- Unit:
GeV/c
- mcPyLER#
[Eventbased] Returns truth value of the y component of the incoming positron momentum in the laboratory frame.
- Unit:
GeV/c
- mcPzHER#
[Eventbased] Returns truth value of the z component of the incoming electron momentum in the laboratory frame.
- Unit:
GeV/c
- mcPzLER#
[Eventbased] Returns truth value of the z component of the incoming positron momentum in the laboratory frame.
- Unit:
GeV/c
- nChargeZeroTrackFits#
[Eventbased] Returns number of track fits with zero charge.
Note
Sometimes, track fits can have zero charge, if background or non IP originating tracks, for example, are fit from the IP. These tracks are excluded from particle lists, but a large amount of charge zero fits may indicate problems with whole event constraints or abnominally high beam backgrounds and/or noisy events.
- nInitialPrimaryMCParticles#
[Eventbased] Returns number of initial primary MCParticles in the event.
- nKLMClusters#
[Eventbased] Returns number of KLM clusters in the event.
- nMCParticles#
[Eventbased] Returns number of MCParticles in the event.
- nNeutralECLClusters(hypothesis)#
[Eventbased] Returns number of neutral ECL clusters with a given hypothesis, 1:nPhotons, 2:NeutralHadron.
- nPrimaryMCParticles#
[Eventbased] Returns number of primary MCParticles in the event.
- nTracks#
[Eventbased] Returns the total number of tracks (unfiltered) in the event.
Warning
This variable is exceedingly background-dependent and should not really be used in any selections (other than perhaps for monitoring purposes).
See also
nCleanedTracks
for a more useful variable for use in selections.
- nV0s#
[Eventbased] Returns number of V0s in the event.
- nValidV0s#
[Eventbased] Returns number of V0s consisting of pair of tracks with opposite charges.
- nVirtualPrimaryMCParticles#
[Eventbased] Returns number of virtual primary MCParticles in the event.
- productionIdentifier#
[Eventbased] Production identifier. Uniquely identifies an MC sample by the (grid-jargon) production ID. This is useful when analysing large MC samples split between more than one production or combining different MC samples (e.g. combining all continuum samples). In such cases the event numbers are sequential only within a production, so experiment/run/event will restart with every new sample analysed.
Tip
Experiment/run/event/production is unique for all MC samples. Experiment/run/event is unique for data.
- revolutionCounter2#
[Eventbased] The lowest bit of revolution counter, i.e. return 0 or 1
Note
related to PXD data acquisition; PXD needs ~2 revolutions to read out one frame
- runNum#
[Eventbased] Returns the run number.
- timeSinceLastInjectionClockTicks#
[Eventbased] Time since the last injected bunch passed by the detector.
- Unit:
clock ticks
- timeSinceLastInjectionMicroSeconds#
[Eventbased] Time since the last injected bunch passed by the detector.
- Unit:
\(\mathrm{\mu s}\)
- timeSinceLastInjectionSignalClockTicks#
[Eventbased] Time since the last injection pre-kick signal (127MHz=RF/4 clock)
Warning
this returns the time without the delay until the injected bunch reaches the detector (which differs for HER/LER)
- Unit:
clock ticks
- timeSinceLastInjectionSignalMicroSeconds#
[Eventbased] Time since the last injection pre-kick signal
Warning
this returns the time without the delay until the injected bunch reaches the detector (which differs for HER/LER)
- Unit:
\(\mathrm{\mu s}\)
- timeSincePrevTriggerClockTicks#
[Eventbased] Time since the previous trigger (127MHz=RF/4 clock).
- Unit:
clock ticks
- timeSincePrevTriggerMicroSeconds#
[Eventbased] Time since the previous trigger.
- Unit:
\(\mathrm{\mu s}\)
- totalEnergyMC#
[Eventbased] Truth value of sum of energies of all the generated particles
- Unit:
GeV
- triggeredBunchNumber#
[Eventbased] Number of triggered bunch ranging from 0-1279.
Note
There are a maximum of 5120 buckets, which could each carry one bunch of e+/e-, but we only have 1280 clock ticks (=5120/4) to identify the bunches
- triggeredBunchNumberTTD#
[Eventbased] Number of triggered bunch ranging from 0-1279.
Warning
This is the bunch number as provided by the TTD, which does not necessarily correspond to the ‘global’ SKB bunch number.
Note
There are a maximum of 5120 buckets, which could each carry one bunch of e+/e-, but we only have 1280 clock ticks (=5120/4) to identify the bunches.
- year#
[Eventbased] Returns the year when the event was recorded (in UTC).
Tip
For more precise event time, see
eventTimeSeconds
andeventTimeSecondsFractionRemainder
.
Parameter Functions#
Here is a list of variables that require a parameter:
- NumberOfMCParticlesInEvent(pdgcode)#
[Eventbased] Returns number of MC Particles (including anti-particles) with the given pdgcode in the event.
Used in the FEI to determine to calculate reconstruction efficiencies.
The variable is event-based and does not need a valid particle pointer as input.
- azimuthalAngleInDecayPlane(i, j)#
Azimuthal angle of i-th daughter in decay plane towards projection of particle momentum into decay plane.
First we define the following symbols:
P: four-momentum vector of decaying particle in whose decay plane the azimuthal angle is measured
M: “mother” of p, however not necessarily the direct mother but any higher state, here the CMS itself is chosen
D1: daughter for which the azimuthal angle is supposed to be calculated
D2: another daughter needed to span the decay plane
L: normal to the decay plane (four-component vector)
L can be defined via the following relation:
\[L^{\sigma} = \delta^{\sigma\nu} \epsilon_{\mu\nu\alpha\beta} P^{\mu}D1^{\alpha}D2^{\beta}\]The azimuthal angle is given by
\[\phi \equiv \cos^{-1} \left(\frac{-\vec{M_{\parallel}} \cdot \vec{D1}}{|\vec{M_{\parallel}}| \cdot |\vec{D1}|}\right)\]For a frame independent formulation the three component vectors need to be written via invariant four-momentum vectors.
\[\begin{split}-\vec{M_{\parallel}} \cdot \vec{D1} &= \biggl[M - \frac{(M \cdot L)L}{L^2}\biggr] \cdot D1 - \frac{(M \cdot P)(D1 \cdot P)}{m^2_P}\\ |\vec{M_{\parallel}}| &= |\vec{M}| \sqrt{1 - \cos^2 \psi}\\ |\vec{M}| &= \sqrt{\frac{(M \cdot P)^2}{m^2_P} - m^2_M}\\ \cos \psi &= \frac{\vec{M} \cdot \vec{L}}{|\vec{M}| \cdot |\vec{L}|} = \frac{-M \cdot L}{|\vec{M}| \cdot \sqrt{-L^2}}\\ |\vec{D1}| &= \sqrt{\frac{(D1 \cdot P)^2}{m^2_P} - m^2_{D1}}\end{split}\]- Unit:
rad
- constant(float i)#
Returns i.
Useful for debugging purposes and in conjunction with the formula meta-variable.
- daughterInvariantMass(i, j, ...)#
Returns invariant mass of the given daughter particles. E.g.:
daughterInvariantMass(0, 1) returns the invariant mass of the first and second daughter.
daughterInvariantMass(0, 1, 2) returns the invariant mass of the first, second and third daughter.
Useful to identify intermediate resonances in a decay, which weren’t reconstructed explicitly.
Returns NaN if particle is nullptr or if the given daughter-index is out of bound (>= number of daughters).
- Unit:
GeV/\(\text{c}^2\)
Note
Deprecated since version light-2203-zeus.
The variable
daughterInvM
provides exactly the same functionality.
- daughterMCInvariantMass(i, j, ...)#
Returns true invariant mass of the given daughter particles, same behaviour as daughterInvariantMass variable.
- Unit:
GeV/\(\text{c}^2\)
- decayAngle(i)#
Angle in the mother’s rest frame between the reverted CMS momentum vector and the direction of the i-th daughter
- Unit:
rad
- hasAncestor(PDG, abs)#
Returns a positive integer if an ancestor with the given PDG code is found, 0 otherwise.
The integer is the level where the ancestor was found, 1: first mother, 2: grandmother, etc.
Second argument is optional, 1 means that the sign of the PDG code is taken into account, default is 0.
If there is no MC relations found, -1 is returned. In case of nullptr particle, NaN is returned.
- isAncestorOf(i, j, ...)#
Returns a positive integer if daughter at position particle->daughter(i)->daughter(j)… is an ancestor of the related MC particle, 0 otherwise.
Positive integer represents the number of steps needed to get from final MC daughter to ancestor. If any particle or MCparticle is a nullptr, NaN is returned. If MC relations of any particle doesn’t exist, -1.0 is returned.
- massDifference(i)#
Difference in invariant masses of this particle and its i-th daughter
- Unit:
GeV/\(\text{c}^2\)
- massDifferenceError(i)#
Estimated uncertainty on difference in invariant masses of this particle and its i-th daughter
- Unit:
GeV/\(\text{c}^2\)
- massDifferenceSignificance(i)#
Signed significance of the deviation from the nominal mass difference of this particle and its i-th daughter [(massDiff - NOMINAL_MASS_DIFF)/ErrMassDiff]
- pointingAngle(i)#
Angle between i-th daughter’s momentum vector and vector connecting production and decay vertex of i-th daughter. This makes only sense if the i-th daughter has itself daughter particles and therefore a properly defined vertex.
- Unit:
rad
- randomChoice(i, j, ...)#
Returns random element of given numbers.
Useful for testing purposes.
Meta Functions#
Here is a list of variables that returns extra info of a given particle:
- abs(variable)#
Returns absolute value of the given variable. E.g. abs(mcPDG) returns the absolute value of the mcPDG, which is often useful for cuts.
- acos(variable)#
Returns arccosine value of the given variable. The unit of the acos() is
rad
- angleToClosestInList(particleListName)#
Returns the angle between this particle and the closest particle (smallest opening angle) in the list provided. The unit of the angle is
rad
- angleToMostB2BInList(particleListName)#
Returns the angle between this particle and the most back-to-back particle (closest opening angle to 180) in the list provided. The unit of the angle is
rad
- asin(variable)#
Returns arcsine of the given variable. The unit of the asin() is
rad
- atan(variable)#
Returns arctangent value of the given variable. The unit of the atan() is
rad
- averageValueInList(particleListName, variable)#
[Eventbased] Returns the arithmetic mean of the given variable of the particles in the given particle list.
- closestInList(particleListName, variable)#
Returns
variable
for the closest particle (smallest opening angle) in the list provided.
- clusterBestMatchedMCParticle(variable)#
Returns variable output for the MCParticle that is best-matched with the ECLCluster of the given Particle. E.g. To get the energy of the MCParticle that matches best with an ECLCluster, one could use
clusterBestMatchedMCParticle(E)
When the variable is called forgamma
and if thegamma
is matched with MCParticle, it works same asmatchedMC
. If the variable is called forgamma
that fails to match with an MCParticle, it provides the mdst-level MCMatching information abouth the ECLCluster. Returns NaN if the particle is not matched to an ECLCluster, or if the ECLCluster has no matching MCParticles
- conditionalVariableSelector(cut, variableIfTrue, variableIfFalse)#
Returns one of the two supplied variables, depending on whether the particle passes the supplied cut. The first variable is returned if the particle passes the cut, and the second variable is returned otherwise.
- cos(variable)#
Returns cosine value of the given variable.
- countDaughters(cut)#
Returns number of direct daughters which satisfy the cut. Used by the skimming package (for what exactly?)
- countDescendants(cut)#
Returns number of descendants for all generations which satisfy the cut.
- countFSPDaughters(cut)#
Returns number of final-state daughters which satisfy the cut.
- countInList(particleList, cut='')#
[Eventbased] Returns number of particle which pass given in cut in the specified particle list. Useful for creating statistics about the number of particles in a list. E.g.
countInList(e+, isSignal == 1)
returns the number of correctly reconstructed electrons in the event. The variable is event-based and does not need a valid particle pointer as input.
- daughter(i, variable)#
Returns value of variable for the i-th daughter. E.g.
daughter(0, p)
returns the total momentum of the first daughter.daughter(0, daughter(1, p)
returns the total momentum of the second daughter of the first daughter.
Returns NaN if particle is nullptr or if the given daughter-index is out of bound (>= amount of daughters).
- daughterAngle(daughterIndex_1, daughterIndex_2[, daughterIndex_3])#
Returns the angle in between any pair of particles belonging to the same decay tree. The unit of the angle is
rad
.The particles are identified via generalized daughter indexes, which are simply colon-separated lists of daughter indexes, ordered starting from the root particle. For example,
0:1:3
identifies the fourth daughter (3) of the second daughter (1) of the first daughter (0) of the mother particle.1
simply identifies the second daughter of the root particle.Both two and three generalized indexes can be given to
daughterAngle
. If two indices are given, the variable returns the angle between the momenta of the two given particles. If three indices are given, the variable returns the angle between the momentum of the third particle and a vector which is the sum of the first two daughter momenta.Tip
daughterAngle(0, 3)
will return the angle between the first and fourth daughter.daughterAngle(0, 1, 3)
will return the angle between the fourth daughter and the sum of the first and second daughter.daughterAngle(0:0, 3:0)
will return the angle between the first daughter of the first daughter, and the first daughter of the fourth daughter.
- daughterClusterAngleInBetween(i, j)#
Returns the angle between clusters associated to the two daughters.If two indices given: returns the angle between the momenta of the clusters associated to the two given daughters.If three indices given: returns the angle between the momentum of the third particle’s cluster and a vector which is the sum of the first two daughter’s cluster momenta.Returns nan if any of the daughters specified don’t have an associated cluster.The arguments in the argument vector must be integers corresponding to the ith and jth (and kth) daughters.
- Unit:
rad
- daughterCombination(variable, daughterIndex_1, daughterIndex_2 ... daughterIndex_n)#
Returns a
variable
function only of the 4-momentum calculated on an arbitrary set of (grand)daughters.Warning
variable
can only be a function of the daughters’ 4-momenta.Daughters from different generations of the decay tree can be combined using generalized daughter indexes, which are simply colon-separated the list of daughter indexes, starting from the root particle: for example,
0:1:3
identifies the fourth daughter (3) of the second daughter (1) of the first daughter (0) of the mother particle.Tip
daughterCombination(M, 0, 3, 4)
will return the invariant mass of the system made of the first, fourth and fifth daughter of particle.daughterCombination(M, 0:0, 3:0)
will return the invariant mass of the system made of the first daughter of the first daughter and the first daughter of the fourth daughter.
- daughterDiffOf(daughterIndex_i, daughterIndex_j, variable)#
Returns the difference of a variable between the two given daughters. E.g.
useRestFrame(daughterDiffOf(0, 1, p))
returns the momentum difference between first and second daughter in the rest frame of the given particle. (That means that it returns \(p_j - p_i\))The daughters can be provided as generalized daughter indexes, which are simply colon-separated lists of daughter indexes, ordered starting from the root particle. For example,
0:1
identifies the second daughter (1) of the first daughter (0) of the mother particle.
- daughterDiffOfClusterPhi(i, j)#
Returns the difference in \(\phi\) between the ECLClusters of two given daughters. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i\). The function returns NaN if at least one of the daughters is not matched to or not based on an ECLCluster.Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of the related ECL clusters of two daughters can be calculated with the generic variable
daughterDiffOf
.
- daughterDiffOfClusterPhiCMS(i, j)#
Returns the difference in \(\phi\) between the ECLClusters of two given daughters in the CMS frame. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i`\). The function returns NaN if at least one of the daughters is not matched to or not based on an ECLCluster.Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of the related ECL clusters of two daughters in the CMS frame can be calculated with the generic variable
daughterDiffOf
.
- daughterDiffOfPhi(i, j)#
Returns the difference in \(\phi\) between the two given daughters. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i\).Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of two daughters can be calculated with the generic variable
daughterDiffOf
.
- daughterDiffOfPhiCMS(i, j)#
Returns the difference in \(\phi\) between the two given daughters in the CMS frame. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i\).Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of two daughters in the CMS frame can be calculated with the generic variable
daughterDiffOf
.
- daughterHighest(variable)#
Returns the highest value of the given variable among all daughters. E.g.
useCMSFrame(daughterHighest(p))
returns the highest momentum in CMS frame.
- daughterInvM(i[, j, ...])#
Returns the invariant mass adding the Lorentz vectors of the given daughters. The unit of the invariant mass is GeV/\(\text{c}^2\) E.g.
daughterInvM(0, 1, 2)
returns the invariant Mass \(m = \sqrt{(p_0 + p_1 + p_2)^2}\) of the first, second and third daughter.Daughters from different generations of the decay tree can be combined using generalized daughter indexes, which are simply colon-separated daughter indexes for each generation, starting from the root particle. For example,
0:1:3
identifies the fourth daughter (3) of the second daughter (1) of the first daughter(0) of the mother particle.Returns NaN if the given daughter-index is out of bound (>= number of daughters)
- daughterLowest(variable)#
Returns the lowest value of the given variable among all daughters. E.g.
useCMSFrame(daughterLowest(p))
returns the lowest momentum in CMS frame.
- daughterMotherDiffOf(i, variable)#
Returns the difference of a variable between the given daughter and the mother particle itself. E.g.
useRestFrame(daughterMotherDiffOf(0, p))
returns the momentum difference between the given particle and its first daughter in the rest frame of the mother.
- daughterMotherNormDiffOf(i, variable)#
Returns the normalized difference of a variable between the given daughter and the mother particle itself. E.g.
daughterMotherNormDiffOf(1, p)
returns the normalized momentum difference between the given particle and its second daughter in the lab frame.
- daughterNormDiffOf(i, j, variable)#
Returns the normalized difference of a variable between the two given daughters. E.g.
daughterNormDiffOf(0, 1, p)
returns the normalized momentum difference between first and second daughter in the lab frame.
- daughterProductOf(variable)#
Returns product of a variable over all daughters. E.g.
daughterProductOf(extraInfo(SignalProbability))
returns the product of the SignalProbabilitys of all daughters.
- daughterSumOf(variable)#
Returns sum of a variable over all daughters. E.g.
daughterSumOf(nDaughters)
returns the number of grand-daughters.
- deltaPhiToMostB2BPhiInList(particleListName)#
Returns the abs(delta phi) between this particle and the most back-to-back particle in phi (closest opening angle to 180) in the list provided. The unit of the angle is
rad
- eclClusterSpecialTrackMatched(cut)#
Returns if at least one Track that satisfies the given condition is related to the ECLCluster of the Particle.
- eventCached(variable)#
[Eventbased] Returns value of event-based variable and caches this value in the EventExtraInfo. The result of second call to this variable in the same event will be provided from the cache. It is recommended to use this variable in order to declare custom aliases as event-based. This is necessary if using the eventwise mode of variablesToNtuple).
- eventExtraInfo(name)#
[Eventbased] Returns extra info stored under the given name in the event extra info. The extraInfo has to be set first by another module like MVAExpert in event mode. If nothing is set under this name, NaN is returned.
- exp(variable)#
Returns exponential evaluated for the given variable.
- extraInfo(name)#
Returns extra info stored under the given name. The extraInfo has to be set by a module first. E.g.
extraInfo(SignalProbability)
returns the SignalProbability calculated by theMVAExpert
module. If nothing is set under the given name or if the particle is a nullptr, NaN is returned. In the latter case please useeventExtraInfo
if you want to access an EventExtraInfo variable.
- formula(v1 + v2 * [v3 - v4] / v5^v6)#
Returns the result of the given formula, where v1 to vN are variables or floating point numbers. Currently the only supported operations are addition (
+
), subtraction (-
), multiplication (*
), division (/
) and power (^
or**
). Parenthesis can be in the form of square brackets[v1 * v2]
or normal brackets(v1 * v2)
. It will work also with variables taking arguments. Operator precedence is taken into account. For example(daughter(0, E) + daughter(1, E))**2 - p**2 + 0.138
Changed in version release-03-00-00: now both,
[]
and()
can be used for grouping operations,**
can be used for exponent and float literals are possible directly in the formula.
- genParticle(index, variable)#
[Eventbased] Returns the
variable
for the ith generator particle. The arguments of the function must be theindex
of the particle in the MCParticle Array, andvariable
, the name of the function or variable for that generator particle. Ifindex
goes beyond the length of the MCParticles array, NaN will be returned.E.g.
genParticle(0, p)
returns the total momentum of the first MCParticle, which in a generic decay up to MC15 is the Upsilon(4S) and for MC16 and beyond the initial electron.
- genUpsilon4S(variable)#
[Eventbased] Returns the
variable
evaluated for the generator-level \(\Upsilon(4S)\). If no generator level \(\Upsilon(4S)\) exists for the event, NaN will be returned.E.g.
genUpsilon4S(p)
returns the total momentum of the \(\Upsilon(4S)\) in a generic decay.genUpsilon4S(mcDaughter(1, p))
returns the total momentum of the second daughter of the generator-level \(\Upsilon(4S)\) (i.e. the momentum of the second B meson in a generic decay).
- getVariableByRank(particleList, rankedVariableName, variableName, rank)#
[Eventbased] Returns the value of
variableName
for the candidate in theparticleList
with the requestedrank
.Note
The
BestCandidateSelection
module available viarankByHighest
/rankByLowest
has to be used before.Warning
The first candidate matching the given rank is used. Thus, it is not recommended to use this variable in conjunction with
allowMultiRank
in theBestCandidateSelection
module.The suffix
_rank
is automatically added to the argumentrankedVariableName
, which either has to be the name of the variable used to order the candidates or the selected outputVariable name without the ending_rank
. This means that your selected name for the rank variable has to end with_rank
.An example of this variable’s usage is given in the tutorial B2A602-BestCandidateSelection
- grandDaughterDecayAngle(i, j)#
Returns the decay angle of the granddaughter in the daughter particle’s rest frame. It is calculated with respect to the reverted momentum vector of the particle. Two arguments representing the daughter and granddaughter indices have to be provided as arguments.
- Unit:
rad
- grandDaughterDiffOf(i, j, variable)#
Returns the difference of a variable between the first daughters of the two given daughters. E.g.
useRestFrame(grandDaughterDiffOf(0, 1, p))
returns the momentum difference between the first daughters of the first and second daughter in the rest frame of the given particle. (That means that it returns \(p_j - p_i\))Note
Deprecated since version light-2402-ocicat.
The difference between any combination of (grand-)daughters can be calculated with the more general variable
daughterDiffOf
by using generalized daughter indexes.
- grandDaughterDiffOfClusterPhi(i, j)#
Returns the difference in \(\phi\) between the ECLClusters of the daughters of the two given daughters. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i\). The function returns NaN if at least one of the daughters is not matched to or not based on an ECLCluster.Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of the related ECL clusters of two granddaughters can be calculated with the generic variable
grandDaughterDiffOf
.
- grandDaughterDiffOfPhi(i, j)#
Returns the difference in \(\phi\) between the first daughters of the two given daughters. The unit of the angle is
rad
. The difference is signed and takes account of the ordering of the given daughters. The function returns \(\phi_j - \phi_i\).Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of two granddaughters can be calculated with the generic variable
grandDaughterDiffOf
.
- ifNANgiveX(variable, x)#
Returns x (has to be a number) if variable value is nan (determined via std::isnan(double)). Useful for technical purposes while training MVAs.
- int(variable, nan_replacement)#
Casts the output of the variable to an integer value.
Note
Overflow and underflow are clipped at maximum and minimum values, respectively. NaN values are replaced with the value of the 2nd argument.
- invMassInLists(pList1, pList2, ...)#
[Eventbased] Returns the invariant mass of the combination of particles in the given particle lists. The unit of the invariant mass is GeV/\(\text{c}^2\)
- isDaughterOfList(particleListNames)#
Returns 1 if the given particle is a daughter of at least one of the particles in the given particle Lists.
- isDescendantOfList(particleListName[, anotherParticleListName][, generationFlag = -1])#
Returns 1 if the given particle appears in the decay chain of the particles in the given ParticleLists.
Passing an integer as the last argument, allows to check if the particle belongs to the specific generation:
isDescendantOfList(<particle_list>,1)
returns 1 if particle is a daughter of the list,isDescendantOfList(<particle_list>,2)
returns 1 if particle is a granddaughter of the list,isDescendantOfList(<particle_list>,3)
returns 1 if particle is a great-granddaughter of the list, etc.Default value is
-1
that is inclusive for all generations.
- isGrandDaughterOfList(particleListNames)#
Returns 1 if the given particle is a grand daughter of at least one of the particles in the given particle Lists.
- isInList(particleListName)#
Returns 1 if the particle is in the list provided, 0 if not. Note that this only checks the particle given. For daughters of composite particles, please see
isDaughterOfList
.
- isInfinity(variable)#
Returns true if variable value evaluates to infinity (determined via std::isinf(double)). Useful for debugging.
- isMCDescendantOfList(particleListName[, anotherParticleListName][, generationFlag = -1])#
Returns 1 if the given particle is linked to the same MC particle as any reconstructed daughter of the decay lists.
Passing an integer as the last argument, allows to check if the particle belongs to the specific generation:
isMCDescendantOfList(<particle_list>,1)
returns 1 if particle is matched to the same particle as any daughter of the list,isMCDescendantOfList(<particle_list>,2)
returns 1 if particle is matched to the same particle as any granddaughter of the list,isMCDescendantOfList(<particle_list>,3)
returns 1 if particle is matched to the same particle as any great-granddaughter of the list, etc.Default value is
-1
that is inclusive for all generations.
It makes only sense for lists created with
fillParticleListFromMC
function withaddDaughters=True
argument.
- isNAN(variable)#
Returns true if variable value evaluates to nan (determined via std::isnan(double)). Useful for debugging.
- log(variable)#
Returns natural logarithm evaluated for the given variable.
- log10(variable)#
Returns base-10 logarithm evaluated for the given variable.
- matchedMC(variable)#
Returns variable output for the matched MCParticle by constructing a temporary Particle from it. This may not work too well if your variable requires accessing daughters of the particle. E.g.
matchedMC(p)
returns the total momentum of the related MCParticle. Returns NaN if no matched MCParticle exists.
- matchedMCHasPDG(PDGCode)#
Returns if the absolute value of the PDGCode of the MCParticle related to the Particle matches a given PDGCode.Returns 0/NAN/1 if PDGCode does not match/is not available/ matches
- max(var1, var2)#
Returns max value of two variables.
- maxOpeningAngleInList(particleListName)#
[Eventbased] Returns maximum opening angle in the given particle List. The unit of the angle is
rad
- maxPtInList(particleListName)#
[Eventbased] Returns maximum transverse momentum Pt in the given particle List. The unit of the transverse momentum is
GeV/c
- mcDaughter(i, variable)#
Returns the value of the requested variable for the i-th Monte Carlo daughter of the particle.
Returns NaN if the particle is nullptr, if the particle is not matched to an MC particle, or if the i-th MC daughter does not exist.
E.g.
mcDaughter(0, PDG)
will return the PDG code of the first MC daughter of the matched MC particle of the reconstructed particle the function is applied to.The meta variable can also be nested:
mcDaughter(0, mcDaughter(1, PDG))
.
- mcDaughterAngle(daughterIndex_1, daughterIndex_2, [daughterIndex_3])#
MC matched version of the
daughterAngle
function. Also works if applied directly to MC particles. The unit of the angle israd
- mcDaughterDiffOf(i, j, variable)#
MC matched version of the
daughterDiffOf
function.
- mcDaughterDiffOfPhi(i, j)#
MC matched version of the
daughterDiffOfPhi
function. The unit of the angle israd
Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of the MC partners of two daughters can be calculated with the generic variable
mcDaughterDiffOf
.
- mcDaughterDiffOfPhiCMS(i, j)#
MC matched version of the
daughterDiffOfPhiCMS
function. The unit of the angle israd
Note
Deprecated since version release-06-00-00.
The difference of the azimuthal angle \(\\phi\) of the MC partners of two daughters in the CMS frame can be calculated with the generic variable
mcDaughterDiffOf
.
- mcMother(variable)#
Returns the value of the requested variable for the Monte Carlo mother of the particle.
Returns NaN if the particle is nullptr, if the particle is not matched to an MC particle, or if the MC mother does not exist.
E.g.
mcMother(PDG)
will return the PDG code of the MC mother of the matched MC particle of the reconstructed particle the function is applied to.The meta variable can also be nested:
mcMother(mcMother(PDG))
.
- mcParticleIsInMCList(particleListName)#
Returns 1 if the particle’s matched MC particle is also matched to a particle in
particleListName
(or if either of the lists were filled from generator levelmodularAnalysis.fillParticleListFromMC
.)See also
isMCDescendantOfList
to check daughters.
- medianValueInList(particleListName, variable)#
[Eventbased] Returns the median value of the given variable of the particles in the given particle list.
- min(var1, var2)#
Returns min value of two variables.
- modulo(variable, n)#
Returns rest of division of variable by n.
- mostB2BInList(particleListName, variable)#
Returns
variable
for the most back-to-back particle (closest opening angle to 180) in the list provided.
- nCleanedECLClusters(cut)#
[Eventbased] Returns the number of clean Clusters in the event Clean clusters are defined by the clusters which pass the given cut assuming a photon hypothesis.
- nCleanedTracks(cut)#
[Eventbased] Returns the number of clean Tracks in the event Clean tracks are defined by the tracks which pass the given cut assuming a pion hypothesis.
- nParticlesInList(particleListName)#
[Eventbased] Returns number of particles in the given particle List.
- nTrackFitResults(particleType)#
[Eventbased] Returns the total number of TrackFitResults for a given particleType. The argument can be the name of particle (e.g. pi+) or PDG code (e.g. 211).
- numberOfNonOverlappingParticles(pList1, pList2, ...)#
Returns the number of non-overlapping particles in the given particle listsUseful to check if there is additional physics going on in the detector if one reconstructed the Y4S
- originalDaughter(i, variable)#
Returns value of variable for the original particle from which the i-th daughter is copied.
The copy of particle is created, for example, when the vertex fit updates the daughters and
modularAnalysis.copyParticles
is called. Returns NaN if the daughter is not copied and so there is no original daughter.Returns NaN if particle is nullptr or if the given daughter-index is out of bound (>= amount of daughters).
- originalParticle(variable)#
Returns value of variable for the original particle from which the given particle is copied.
The copy of particle is created, for example, when the vertex fit updates the daughters and
modularAnalysis.copyParticles
is called. Returns NaN if the given particle is not copied and so there is no original particle.
- pValueCombination(p1, p2, ...)#
Returns the combined p-value of the provided p-values according to the formula given in Nucl. Instr. and Meth. A 411 (1998) 449 . If any of the p-values is invalid, i.e. smaller than zero, -1 is returned.
- particleCached(variable)#
Returns value of given variable and caches this value in the ParticleExtraInfo of the provided particle. The result of second call to this variable on the same particle will be provided from the cache.
- passesCut(cut)#
Returns 1 if particle passes the cut otherwise 0. Useful if you want to write out if a particle would have passed a cut or not.
- passesEventCut(cut)#
[Eventbased] Returns 1 if event passes the cut otherwise 0. Useful if you want to select events passing a cut without looping into particles, such as for skimming.
- productValueInList(particleListName, variable)#
[Eventbased] Returns the product of the given variable of the particles in the given particle list.
- sin(variable)#
Returns sine value of the given variable.
- sourceObjectIsInList(particleListName)#
Returns 1 if the underlying mdst object (e.g. track, or cluster) was used to create a particle in
particleListName
, 0 if not.Note
This only makes sense for particles that are not composite. Returns -1 for composite particles.
- sumValueInList(particleListName, variable)#
[Eventbased] Returns the sum of the given variable of the particles in the given particle list.
- tan(variable)#
Returns tangent value of the given variable.
- totalECLEnergyOfParticlesInList(particleListName)#
[Eventbased] Returns the total ECL energy of particles in the given particle List. The unit of the energy is
GeV
- totalEnergyOfParticlesInList(particleListName)#
[Eventbased] Returns the total energy of particles in the given particle List. The unit of the energy is
GeV
- totalPxOfParticlesInList(particleListName)#
[Eventbased] Returns the total momentum Px of particles in the given particle List. The unit of the momentum is
GeV/c
- totalPyOfParticlesInList(particleListName)#
[Eventbased] Returns the total momentum Py of particles in the given particle List. The unit of the momentum is
GeV/c
- totalPzOfParticlesInList(particleListName)#
[Eventbased] Returns the total momentum Pz of particles in the given particle List. The unit of the momentum is
GeV/c
- unmask(variable, flag1, flag2, ...)#
unmask(variable, flag1, flag2, …) or unmask(variable, mask) sets certain bits in the variable to zero. For example, if you want to set the second, fourth and fifth bits to zero, you could call
unmask(variable, 2, 8, 16)
orunmask(variable, 26)
.
- useAlternativeDaughterHypothesis(variable, daughterIndex_1:newMassHyp_1, ..., daughterIndex_n:newMassHyp_n)#
Returns a
variable
calculated using new mass hypotheses for (some of) the particle’s daughters.Warning
variable
can only be a function of the particle 4-momentum, which is re-calculated as the sum of the daughters’ 4-momenta, and the daughters’ 4-momentum. This means that if you made a kinematic fit without updating the daughters’ momenta, the result of this variable will not reflect the effect of the kinematic fit. Also, the track fit is not performed again: the variable only re-calculates the 4-vectors using different mass assumptions. In the variable, a copy of the given particle is created with daughters’ alternative mass assumption (i.e. the original particle and daughters are not changed).Warning
Generalized daughter indexes are not supported (yet!): this variable can be used only on first-generation daughters.
Tip
useAlternativeDaughterHypothesis(M, 0:K+, 2:pi-)
will return the invariant mass of the particle assuming that the first daughter is a kaon and the third is a pion, instead of whatever was used in reconstructing the decay.useAlternativeDaughterHypothesis(mRecoil, 1:p+)
will return the recoil mass of the particle assuming that the second daughter is a proton instead of whatever was used in reconstructing the decay.
- useCMSFrame(variable)#
Returns the value of the variable using the CMS frame as current reference frame. E.g.
useCMSFrame(E)
returns the energy of a particle in the CMS frame.
- useDaughterRestFrame(variable, daughterIndex_1, [daughterIndex_2, ... daughterIndex_3])#
Returns the value of the variable in the rest frame of the selected daughter particle. The daughter is identified via generalized daughter index, e.g.
0:1
identifies the second daughter (1) of the first daughter (0). If the daughter index is invalid, it returns NaN. If two or more indices are given, the rest frame of the sum of the daughters is used.
- useLabFrame(variable)#
Returns the value of
variable
in the lab frame.Tip
The lab frame is the default reference frame, usually you don’t need to use this meta-variable. E.g.
useLabFrame(E)
returns the energy of a particle in the Lab frame, same as justE
.Specifying the lab frame is useful in some corner-cases. For example:
useRestFrame(daughter(0, formula(E - useLabFrame(E))))
which is the difference of the first daughter’s energy in the rest frame of the mother (current particle) with the same daughter’s lab-frame energy.
- useMCancestorBRestFrame(variable)#
Returns the value of the variable in the rest frame of the ancestor B MC particle. If no B or no MC-matching is found, it returns NaN.
- useParticleRestFrame(variable, particleList)#
Returns the value of the variable in the rest frame of the first Particle contained in the given ParticleList. It is strongly recommended to pass a ParticleList that contains at most only one Particle in each event. When more than one Particle is present in the ParticleList, only the first Particle in the list is used for computing the rest frame and a warning is thrown. If the given ParticleList is empty in an event, it returns NaN.
- useRecoilParticleRestFrame(variable, particleList)#
Returns the value of the variable in the rest frame of recoil system against the first Particle contained in the given ParticleList. It is strongly recommended to pass a ParticleList that contains at most only one Particle in each event. When more than one Particle is present in the ParticleList, only the first Particle in the list is used for computing the rest frame and a warning is thrown. If the given ParticleList is empty in an event, it returns NaN.
- useRestFrame(variable)#
Returns the value of the variable using the rest frame of the given particle as current reference frame. E.g.
useRestFrame(daughter(0, p))
returns the total momentum of the first daughter in its mother’s rest-frame
- useTagSideRecoilRestFrame(variable, daughterIndexTagB)#
Returns the value of the variable in the rest frame of the recoiling particle to the tag side B meson. The variable should only be applied to an Upsilon(4S) list. E.g.
useTagSideRecoilRestFrame(daughter(1, daughter(1, p)), 0)
applied on a Upsilon(4S) list (Upsilon(4S)->B+:tag B-:sig
) returns the momentum of the second daughter of the signal B meson in the signal B meson rest frame.
- varFor(pdgCode, variable)#
Returns the value of the variable for the given particle if its abs(pdgCode) agrees with the given one. E.g.
varFor(11, p)
returns the momentum if the particle is an electron or a positron.
- varForBestMatchedMCKlong(variable)#
Returns variable output for the Klong MCParticle which has the best match with the ECLCluster of the given Particle. Returns NaN if the particle is not matched to an ECLCluster, or if the ECLCluster has no matching Klong MCParticle
- varForFirstMCAncestorOfType(type, variable)#
Returns requested variable of the first ancestor of the given type. Ancestor type can be set up by PDG code or by particle name (check evt.pdl for valid particle names)
- varForMCGen(variable)#
Returns the value of the variable for the given particle if the MC particle related to it is primary, not virtual, and not initial. If no MC particle is related to the given particle, or the MC particle is not primary, virtual, or initial, NaN will be returned. E.g.
varForMCGen(PDG)
returns the PDG code of the MC particle related to the given particle if it is primary, not virtual, and not initial.
- veto(particleList, cut, pdgCode = 11)#
Combines current particle with particles from the given particle list and returns 1 if the combination passes the provided cut. For instance one can apply this function on a signal Photon and provide a list of all photons in the rest of event and a cut around the neutral Pion mass (e.g.
0.130 < M < 0.140
). If a combination of the signal Photon with a ROE photon fits this criteria, hence looks like a neutral pion, the veto-Metavariable will return 1
MC matching and MC truth#
Here is a list of MC truth-related variables. For some variables, you will need to run truth matching in order to get sensible results.
from modularAnalysis import matchMCTruth
matchMCTruth("B0:myCandidates") # for example
Variables will also work on generator-level particles:
from modularAnalysis import fillParticleListFromMC
fillParticleListFromMC("B0:generator", "") # the generator-level B particles
- Eher#
[Eventbased] The nominal HER energy used by the generator.
Warning
This variable does not make sense for data.
- Unit:
GeV
- Eler#
[Eventbased] The nominal LER energy used by the generator.
Warning
This variable does not make sense for data.
- Unit:
GeV
- XAngle#
[Eventbased] The nominal beam crossing angle in the x-z plane from generator level beam kinematics.
Warning
This variable does not make sense for data.
- Unit:
rad
- YAngle#
[Eventbased] The nominal beam crossing angle in the y-z plane from generator level beam kinematics.
Warning
This variable does not make sense for data.
- Unit:
rad
- ancestorBIndex#
Returns array index of B ancestor, or -1 if no B or no MC-matching is found.
- genMotherID#
Check the array index of a particles generated mother
- genMotherID(i)
Check the array index of a particle n-th MC mother particle by providing an argument. 0 is first mother, 1 is grandmother etc.
- genMotherP#
Generated momentum of a particles MC mother particle
- Unit:
GeV/c
- genMotherPDG#
Check the PDG code of a particles MC mother particle
- genMotherPDG(i)
Check the PDG code of a particles n-th MC mother particle by providing an argument. 0 is first mother, 1 is grandmother etc.
- genNMissingDaughter(PDG)#
Returns the number of missing daughters having assigned PDG codes. NaN if no MCParticle is associated to the particle.
- genNStepsToDaughter(i)#
Returns number of steps to i-th daughter from the particle at generator level. NaN if no MCParticle is associated to the particle or i-th daughter. NaN if i-th daughter does not exist.
- genParticleID#
Check the array index of a particle’s related MCParticle
- genQ2PmPd(i, j, ...)#
Returns the generated four momentum transfer squared \(q^2\) calculated as \(q^2 = (p_m - p_{d_i} - p_{d_j} - ...)^2\).
Here \(p_m\) is the four momentum of the given (mother) particle, and \(p_{d_{i,j,...}}\) are the daughter particles with indices given as arguments . The ordering of daughters is as defined in the DECAY.DEC file used in the generation, with the numbering starting at \(N=0\).
Returns NaN if no related MCParticle could be found. Returns NaN if any of the given indices is larger than the number of daughters of the given particle.
- Unit:
\([\text{GeV}/\text{c}]^2\)
- generatorEventWeight#
[Eventbased] Returns the event weight produced by the event generator
- isBBCrossfeed#
Returns 1 for crossfeed in reconstruction of given B meson, 0 for no crossfeed and NaN for no true B meson or failed truthmatching.
- isCloneTrack#
Return 1 if the charged final state particle comes from a cloned track, 0 if not a clone. Returns NAN if neutral, composite, or MCParticle not found (like for data or if not MCMatched)
- isMisidentified#
Return 1 if the particle is misidentified: at least one of the final state particles has the wrong PDG code assignment (including wrong charge), 0 if PDG code is fine, and NaN if no related MCParticle could be found.
- isOrHasCloneTrack#
Return 1 if the particle is a clone track or has a clone track as a daughter, 0 otherwise.
- isPrimarySignal#
1.0 if Particle is correctly reconstructed (SIGNAL) and primary, 0.0 if not, and NaN if no related MCParticle could be found
- isSignal#
1.0 if Particle is correctly reconstructed (SIGNAL), 0.0 if not, and NaN if no related MCParticle could be found. It behaves according to DecayStringGrammar.
- isSignalAcceptBremsPhotons#
1.0 if Particle is correctly reconstructed (SIGNAL), 0.0 if not, and NaN if no related MCParticle could be found. Particles with gamma daughters attached through the bremsstrahlung recovery modules are allowed.
- isSignalAcceptMissing#
Same as isSignal, but also accept missing particle
- isSignalAcceptMissingGamma#
Same as isSignal, but also accept missing gamma, such as B -> K* gamma, pi0 -> gamma gamma
- isSignalAcceptMissingMassive#
Same as isSignal, but also accept missing massive particle
- isSignalAcceptMissingNeutrino#
Same as isSignal, but also accept missing neutrino
- isSignalAcceptWrongFSPs#
1.0 if Particle is almost correctly reconstructed (SIGNAL), 0.0 if not, and NaN if no related MCParticle could be found. Misidentification of charged FSP is allowed.
- isWrongCharge#
Return 1 if the charge of the particle is wrongly assigned, 0 if it’s the correct charge, and NaN if no related MCParticle could be found.
- mcCosThetaBetweenParticleAndNominalB#
Cosine of the angle in CMS between momentum the particle and a nominal B particle. In this calculation, the momenta of all descendant neutrinos are subtracted from the B momentum.
- mcDecayTime#
The decay time of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
ns
- mcE#
The energy of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV
- mcErrors#
The bit pattern indicating the quality of MC match (see MCMatching::MCErrorFlags)
- mcFSR#
Returns 1 if Particle is related to FSR MCParticle, 0 if Particle is related to non - FSR MCParticle ,NaN if Particle is not related to MCParticle.
- mcISR#
Returns 1 if Particle is related to ISR MCParticle, 0 if Particle is related to non - ISR MCParticle, NaN if Particle is not related to MCParticle.
- mcInitial#
Returns 1 if Particle is related to initial MCParticle, 0 if Particle is related to non - initial MCParticle, NaN if Particle is not related to MCParticle.
- mcLifeTime#
The life time of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
ns
- mcMatchWeight#
The weight of the Particle -> MCParticle relation (only for the first Relation = largest weight).
- mcP#
The total momentum of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV/c
- mcPDG#
The PDG code of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- mcPT#
The pt of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV/c
- mcPX#
The px of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV/c
- mcPY#
The py of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV/c
- mcPZ#
The pz of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
GeV/c
- mcParticleStatus#
Returns status bits of related MCParticle or NaN if MCParticle relation is not set.
- mcPhi#
The phi of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
rad
- mcPhotos#
Returns 1 if Particle is related to Photos MCParticle, 0 if Particle is related to non - Photos MCParticle, NaN if Particle is not related to MCParticle.
- mcPrimary#
Returns 1 if Particle is related to primary MCParticle, 0 if Particle is related to non - primary MCParticle, NaN if Particle is not related to MCParticle.
- mcRecoilMass#
The mass recoiling against the particles attached as particle’s daughters calculated using MC truth values.
- Unit:
GeV/\(\text{c}^2\)
- mcSecPhysProc#
Returns the secondary physics process flag, which is set by Geant4 on secondary particles. It indicates the type of process that produced the particle.
Returns NaN if the particle is not matched to a MCParticle.
Returns -1 in case of unknown process.
Returns 0 if the particle is primary, i.e. produced by the event generator and not Geant4. Particles produced by Geant4 (i.e. secondary particles) include those produced in interaction with detector material, Bremsstrahlung, and the decay products of long-lived particles (e.g. muons, pions, K_S0, K_L0, Lambdas, …).
List of possible values (taken from the Geant4 source of G4DecayProcessType, G4HadronicProcessType, G4TransportationProcessType and G4EmProcessSubType)
1 Coulomb scattering
2 Ionisation
3 Bremsstrahlung
4 Pair production by charged
5 Annihilation
6 Annihilation to mu mu
7 Annihilation to hadrons
8 Nuclear stopping
9 Electron general process
10 Multiple scattering
11 Rayleigh
12 Photo-electric effect
13 Compton scattering
14 Gamma conversion
15 Gamma conversion to mu mu
16 Gamma general process
21 Cerenkov
22 Scintillation
23 Synchrotron radiation
24 Transition radiation
91 Transportation
92 Coupled transportation
111 Hadron elastic
121 Hadron inelastic
131 Capture
132 Mu atomic capture
141 Fission
151 Hadron at rest
152 Lepton at rest
161 Charge exchange
201 Decay
202 Decay with spin
203 Decay (pion make spin)
210 Radioactive decay
211 Unknown decay
221 Mu atom decay
231 External decay
Note
This is what
modularAnalysis.printMCParticles
shows ascreation process
whenshowStatus
is set toTrue
.
- mcTheta#
The theta of matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- Unit:
rad
- mcVirtual#
Returns 1 if Particle is related to virtual MCParticle, 0 if Particle is related to non - virtual MCParticle, NaN if Particle is not related to MCParticle.
- nMCDaughters#
The number of daughters of the matched MCParticle, NaN if no match. Requires running matchMCTruth() on the reconstructed particles, or a particle list filled with generator particles (MCParticle objects).
- nMCMatches#
The number of relations of this Particle to MCParticle.
- isReconstructible#
Checks charged particles were seen in the SVD and neutrals in the ECL, returns 1.0 if so, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- isTrackFound#
works on charged stable particle list created from MCParticles, returns NaN if not ; returns 1.0 if there is a reconstructed track related to the charged stable MCParticle with the correct charge, return -1.0 if the reconstructed track has the wrong charge, return 0.0 when no reconstructed track is found.
- seenInARICH#
Returns 1.0 if the MC particle was seen in the ARICH, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInCDC#
Returns 1.0 if the MC particle was seen in the CDC, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInECL#
Returns 1.0 if the MC particle was seen in the ECL, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInKLM#
Returns 1.0 if the MC particle was seen in the KLM, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInPXD#
Returns 1.0 if the MC particle was seen in the PXD, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInSVD#
Returns 1.0 if the MC particle was seen in the SVD, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- seenInTOP#
Returns 1.0 if the MC particle was seen in the TOP, 0.0 if not, NaN for composite particles or if no related MCParticle could be found. Useful for generator studies, not for reconstructed particles.
- B0Mode
[Eventbased] It will return the decays mode of B0 particles
- Bbar0Mode
[Eventbased] It will return the decays mode of anti-B0 particles
- BminusMode
[Eventbased] It will return the decays mode of B- particles
- BplusMode
[Eventbased] It will return the decays mode of B+ particles
- Bs0Mode
[Eventbased] It will return the decays mode of B_s0 particles
- Bsbar0Mode
[Eventbased] It will return the decays mode of anti-B_s0 particles
- D0Mode
[Eventbased] It will return the decays mode of D0 particles
- Dbar0Mode
[Eventbased] It will return the decays mode of anti-D0 particles
- DminusMode
[Eventbased] It will return the decays mode of D- particles
- DplusMode
[Eventbased] It will return the decays mode of D+ particles
- DsminusMode
[Eventbased] It will return the decays mode of D_s- particles
- DsplusMode
[Eventbased] It will return the decays mode of D_s+ particles
- DstminusMode
[Eventbased] It will return the decays mode of D*- particles
- DstplusMode
[Eventbased] It will return the decays mode of D*+ particles
- TauminusMode
[Eventbased] It will return the decays mode of tau- particles
- TauplusMode
[Eventbased] It will return the decays mode of tau+ particles
- tauMinusEgstar
[Eventbased] Energy of radiated gamma from negative tau lepton in a tau pair generated event.
- tauMinusMCMode
[Eventbased] Decay ID for the negative tau lepton in a tau pair generated event.
- tauMinusMCProng
[Eventbased] Prong for the negative tau lepton in a tau pair generated event.
- tauPlusEgstar
[Eventbased] Energy of radiated gamma from positive tau lepton in a tau pair generated event.
- tauPlusMCMode
[Eventbased] Decay ID for the positive tau lepton in a tau pair generated event.
- tauPlusMCProng
[Eventbased] Prong for the positive tau lepton in a tau pair generated event.
Daughter info#
Here is a list of variables getting info from particle’s daughters:
- hasCharmedDaughter(i)#
If i = 1 is provided it checks for b -> anti-c / anti-b -> c transition and for i = -1 it checks for b -> c / anti-b -> anti-c transitions. Returns 1 if at least one of the daughters on MC truth level is a charm meson. The particle’s MC partner must be a B-meson. Returns 0 if no charmed daughter found on MC truth level and NaN if no MC partner was found or the related MC particle isn’t a B-meson.
- hasCharmoniumDaughter#
Returns 1 if at least one of the daughters on MC truth level is a ccbar resonance. The particle’s MC partner must be a B-meson. Returns 0 if no ccbar resonance found on MC truth level and NaN if no MC partner was found or the related MC particle isn’t a B-meson.
- hasRealPhotonDaughter#
Returns 1 if on MC truth level there is at least one real photon daughter, a photon that was not created by photos. Returns 0 if no real photon daughter found and NaN if the particle has no daughters.
KLM Cluster and \(K_{L}^0\) Identification#
Here is a list of KLM Cluster and \(K_{L}^0\) identification variables:
Warning
Please note that these variables refer to KLMClusters, which are designed to reconstruct \(K_{L}^0\) and other neutral particles with the KLM subdetector. These variables must not be used to do particle identification of charged tracks (for example, they must not be used to identify muons), otherwise there is a serious risk to spoil a physics analysis.
For particle identification of charged tracks, please use the canonical PID variables.
- klmClusterBelleECLFlag#
Returns the Belle-style ECL flag.
- klmClusterBelleTrackFlag#
Returns the Belle-style Track flag.
- klmClusterEnergy#
Returns the energy of the associated KLMCluster.
Warning
This variable returns an approximation of the energy: it uses
klmClusterMomentum
as momentum and the hypothesis that the KLMCluster is originated by a \(K_{L}^0\) (\(E_{\text{KLM}} = \sqrt{M_{K^0_L}^2 + p_{\text{KLM}}^2}\), where \(E_{\text{KLM}}\) is this variable, \(M_{K^0_L}\) is the \(K^0_L\) mass and \(p_{\text{KLM}}\) isklmClusterMomentum
). It should be used with caution, and may not be physically meaningful, especially for \(n\) candidates.- Unit:
GeV
- klmClusterInnermostLayer#
Returns the number of the innermost KLM layer with a 2-dimensional hit of the associated KLMCluster.
- klmClusterIsBKLM#
Returns 1 if the associated KLMCluster is in barrel KLM.
- klmClusterIsBackwardEKLM#
Returns 1 if the associated KLMCluster is in backward endcap KLM.
- klmClusterIsEKLM#
Returns 1 if the associated KLMCluster is in endcap KLM.
- klmClusterIsForwardEKLM#
Returns 1 if the associated KLMCluster is in forward endcap KLM.
- klmClusterKlId#
Returns the KlId classifier output associated to the KLMCluster.
- klmClusterLayers#
Returns the number of KLM layers with 2-dimensional hits of the associated KLMCluster.
- klmClusterMomentum#
Returns the momentum magnitude of the associated KLMCluster.
Warning
This variable returns an approximation of the momentum, since it is proportional to
klmClusterLayers
(\(p_{\text{KLM}} = 0.215 \cdot N_{\text{layers}}\), where \(p_{\text{KLM}}\) is this variable and \(N_{\text{layers}}\) isklmClusterLayers
). It should be used with caution, and may not be physically meaningful.- Unit:
GeV/c
- klmClusterPhi#
Returns the azimuthal (\(\phi\)) angle of the associated KLMCluster.
- Unit:
rad
- klmClusterPositionX#
Returns the \(x\) position of the associated KLMCluster.
- Unit:
cm
- klmClusterPositionY#
Returns the \(y\) position of the associated KLMCluster.
- Unit:
cm
- klmClusterPositionZ#
Returns the \(z\) position of the associated KLMCluster.
- Unit:
cm
- klmClusterTheta#
Returns the polar (\(\theta\)) angle of the associated KLMCluster.
- Unit:
rad
- klmClusterTiming#
Returns the timing information of the associated KLMCluster.
- Unit:
ns
- klmClusterTrackDistance#
Returns the distance between the Track and the KLMCluster associated to this Particle. This variable returns NaN if there is no Track-to-KLMCluster relationship.
- Unit:
cm
- maximumKLMAngleCMS#
Returns the maximum angle in the CMS frame between the Particle and all KLMClusters in the event.
- Unit:
rad
- nKLMClusterECLClusterMatches#
Returns the number of ECLClusters matched to the KLMCluster associated to this Particle.
- nKLMClusterTrackMatches#
Returns the number of Tracks matched to the KLMCluster associated to this Particle. This variable can return a number greater than 0 for \(K_{L}^0\) or \(n\) candidates originating from KLMClusters and returns NaN for Particles with no KLMClusters associated.
- nMatchedKLMClusters#
Returns the number of KLMClusters matched to the particle. It only works for Particles created either from Tracks or from ECLCluster, while it returns NaN for \(K_{L}^0\) or \(n\) candidates originating from KLMClusters.
Time Dependent CPV Analysis Variables#
To use most of the variables in this section on need to run vertex.TagV
method:
- DeltaBoost#
\(\Delta z\) in the boost direction
- Unit:
cm
- DeltaBoostErr#
Uncertainty of \(\Delta z\) in the boost direction
- Unit:
cm
- DeltaT#
Proper decay time difference \(\Delta t\) between signal B-meson \((B_{rec})\) and tag B-meson \((B_{tag})\).
- Unit:
ps
- DeltaT3D#
Returns the \(\Delta t\) variable calculated as a difference of
tSigB
andtTagB
, i.e. not from the projection along boost vector axis.The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
ps
- DeltaTBelle#
[Legacy] \(\Delta t\), as it was used in Belle
- Unit:
ps
- DeltaTErr#
Proper decay time difference \(\Delta t\) uncertainty
- Unit:
ps
- DeltaTRes#
\(\Delta t\) residual, to be used for resolution function studies
- Unit:
ps
- DeltaZ#
Difference of decay vertex longitudinal components between signal B-meson \((B_{rec})\) and tag B-meson \((B_{tag})\): \(\Delta z = z(B_{rec}) - z(B_{tag})\)
- Unit:
cm
- DeltaZErr#
Uncertainty of the difference \(z(B_{rec}) - z(B_{tag})\)
- Unit:
cm
- LBoost#
Returns the vertex component in the boost direction
- Unit:
cm
- LBoostErr#
Returns the error of the vertex in the boost direction
- Unit:
cm
- OBoost#
Returns the vertex component in the direction orthogonal to the boost
- Unit:
cm
- OBoostErr#
Returns the error of the vertex in the direction orthogonal to the boost
- Unit:
cm
- TagTrackAverage(var)#
return the average over the tag tracks of the variable
var
.var
must be one of the TagTrackXXX variables, for example:TagTrackAverage(TagTrackDistanceToConstraint)
. The tracks that are assigned a zero weight are ignored.
- TagTrackAverageSquares(var)#
return the average over the tag tracks of the square of the variable
var
.var
must be one of the TagTrackXXX variables, for example:TagTrackAverageSquares(TagTrackDistanceToConstraint)
. The tracks that are assigned a zero weight are ignored.
- TagTrackD0(i)#
Returns the d0 parameter of the ith track used in the tag vtx fit
- Unit:
cm
- TagTrackDistanceToConstraint(i)#
Returns the measured distance between the ith tag track and the centre of the constraint.
- Unit:
cm
- TagTrackDistanceToConstraintErr(i)#
Returns the estimated error on the distance between the ith tag track and the centre of the constraint.
- Unit:
cm
- TagTrackDistanceToConstraintSignificance(i)#
Returns the significance of the distance between the centre of the constraint and the tag track indexed by track index (computed as distance / uncertainty)
- TagTrackDistanceToTagV(i)#
Returns the measured distance between the ith tag track and the tag vtx.
- Unit:
cm
- TagTrackDistanceToTagVErr(i)#
Returns the estimated error on the distance between the ith tag track and the tag vtx.
Warning
Only the uncertainties on the track position parameters are taken into account.
- Unit:
cm
- TagTrackDistanceToTagVSignificance(i)#
Returns the significance of the distance between the tag vtx and the tag track indexed by trackIndex (computed as distance / uncertainty)
- TagTrackMax(var)#
return the maximum value of the variable
var
evaluated for each tag track.var
must be one of the TagTrackXXX variables, for example:TagTrackMax(TagTrackDistanceToConstraint)
. The tracks that are assigned a zero weight are ignored.
- TagTrackMin(var)#
return the minimum value of the variable
var
evaluated for each tag track.var
must be one of the TagTrackXXX variables, for example:TagTrackMin(TagTrackDistanceToConstraint)
. The tracks that are assigned a zero weight are ignored.
- TagTrackMomentum(i)#
Returns the magnitude of the momentum of the ith track used in the tag vtx fit.
- Unit:
GeV/c
- TagTrackMomentumX(i)#
Returns the X component of the momentum of the ith track used in the tag vtx fit.
- Unit:
GeV/c
- TagTrackMomentumY(i)#
Returns the Y component of the momentum of the ith track used in the tag vtx fit.
- Unit:
GeV/c
- TagTrackMomentumZ(i)#
Returns the Z component of the momentum of the ith track used in the tag vtx fit.
- Unit:
GeV/c
- TagTrackRaveWeight(i)#
Returns the weight assigned by Rave to track i
- TagTrackSum(var)#
Returns the sum of the provided variable for all tag tracks. The variable must be one of the TagTrackXXX variables, for example
TagTrackSum(TagTrackD0)
. The tracks that are assigned a zero weight are ignored.
- TagTrackTrueDistanceToTagV(i)#
Returns the true distance between the true B Tag decay vertex and the particle corresponding to the ith tag vtx track.
- Unit:
cm
- TagTrackTrueMomentumX(i)#
Returns the X component of the true momentum of the MC particle corresponding to the ith tag vtx track.
- Unit:
GeV/c
- TagTrackTrueMomentumY(i)#
Returns the Y component of the true momentum of the MC particle corresponding to the ith tag vtx track.
- Unit:
GeV/c
- TagTrackTrueMomentumZ(i)#
Returns the Z component of the true momentum of the MC particle corresponding to the ith tag vtx track.
- Unit:
GeV/c
- TagTrackTrueOriginX(i)#
Returns the X component of the true origin of the MC particle corresponding to the ith tag vtx track.
- Unit:
cm
- TagTrackTrueOriginY(i)#
Returns the Y component of the true origin of the MC particle corresponding to the ith tag vtx track.
- Unit:
cm
- TagTrackTrueOriginZ(i)#
Returns the Z component of the true origin of the MC particle corresponding to the ith tag vtx track.
- Unit:
cm
- TagTrackTrueVecToTagVX(i)#
Returns the X coordinate of the vector between the mc particle corresponding to the ith tag vtx track and the true tag B decay vertex.
- Unit:
cm
- TagTrackTrueVecToTagVY(i)#
Returns the Y coordinate of the vector between the mc particle corresponding to the ith tag vtx track and the true tag B decay vertex.
- Unit:
cm
- TagTrackTrueVecToTagVZ(i)#
Returns the Z coordinate of the vector between the mc particle corresponding to the ith tag vtx track and the true tag B decay vertex.
- Unit:
cm
- TagTrackWeightedAverage(var)#
return the average over the tag tracks of the variable
var
, weighted by weights of the tag vertex fitter.var
must be one of the TagTrackXXX variables, for example:TagTrackWeightedAverage(TagTrackDistanceToConstraint)
.
- TagTrackWeightedAverageSquares(var)#
return the average over the tag tracks of the variable
var
, weighted by weights of the tag vertex fitter.var
must be one of the TagTrackXXX variables, for example:TagTrackWeightedAverageSquares(TagTrackDistanceToConstraint)
.
- TagTrackZ0(i)#
Returns the z0 parameter of the ith track used in the tag vtx fit
- Unit:
cm
- TagVChi2#
chi2 value of the tag vertex fit
- TagVChi2IP#
IP component of chi2 value of the tag vertex fit
- TagVCov(i, j)#
returns the (i,j)-th element of the Tag vertex covariance matrix (3x3). Order of elements in the covariance matrix is: x, y, z.
- Unit:
cm, cm, cm
- TagVDistanceToConstraint#
returns the measured distance between the tag vtx and the centre of the constraint.
- Unit:
cm
- TagVDistanceToConstraintErr#
returns the estimated error on the distance between the tag vtx and the centre of the constraint.
- Unit:
cm
- TagVDistanceToConstraintSignificance#
returns the significance of the distance between the tag vtx and the centre of the constraint (computed as distance / uncertainty)
- TagVFitTruthStatus#
Returns the status of the fit performed with the truth info. Possible values are:
-1: no related TagVertex found
0: fit performed with measured parameters
1: fit performed with true parameters
2: unable to recover truth parameters
- TagVLBoost#
Returns the TagV component in the boost direction
- Unit:
cm
- TagVLBoostErr#
Returns the error of TagV in the boost direction
- Unit:
cm
- TagVNDF#
Number of degrees of freedom in the tag vertex fit
- TagVNFitTracks#
returns the number of tracks used by rave to fit the vertex (not counting the ones coming from Kshorts)
- TagVNTracks#
Number of tracks in the tag vertex
- TagVOBoost#
Returns the TagV component in the direction orthogonal to the boost
- Unit:
cm
- TagVOBoostErr#
Returns the error of TagV in the direction orthogonal to the boost
- Unit:
cm
- TagVRollBackStatus#
Returns the status of the fit performed with rolled back tracks. Possible values are:
-1: no related TagVertex found
0: fit performed with measured parameters
1: fit performed with true parameters
2: unable to recover truth parameters
- TagVType#
Type of algorithm for the tag vertex. -1: failed (1,2: single track, deprecated), 3: standard, 4: standard_PXD, 5: no constraint
- TagVpVal#
Tag vertex p-Value
- TagVx#
Tag vertex X component
- Unit:
cm
- TagVxErr#
Tag vertex X component uncertainty
- Unit:
cm
- TagVy#
Tag vertex Y component
- Unit:
cm
- TagVyErr#
Tag vertex Y component uncertainty
- Unit:
cm
- TagVz#
Tag vertex Z component
- Unit:
cm
- TagVzErr#
Tag vertex Z component uncertainty
- Unit:
cm
- Y4SvtxX#
Returns X component of Y4S vertex. The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
cm
- Y4SvtxY#
Returns Y component of Y4S vertex. The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
cm
- Y4SvtxZ#
Returns Z component of Y4S vertex. The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
cm
- cosAngleBetweenMomentumAndBoostVector#
cosine of the angle between momentum and boost vector
- internalTagVMCFlavor#
[Expert] [Debugging] This variable is only for internal checks of the TagV module by developers. It returns the internal mc flavor information of the tag-side B provided by the TagV module.
- mcDeltaBoost#
True difference of decay vertex boost-direction components between signal B-meson \((B_{rec})\) and tag B-meson \((B_{tag})\): \(\Delta l = l(B_{rec}) - l(B_{tag})\)
- Unit:
cm
- mcDeltaT#
- Generated proper decay time difference (in z-difference approximation) \(\Delta t\):
\((l(B_{\rm rec}) - l(B_{\rm tag}))/\beta_{\Upsilon(4S)}\gamma_{\Upsilon(4S)}\)
- Unit:
ps
- mcDeltaTau#
Generated proper decay time difference \(\Delta t\): \(\tau(B_{\rm rec})-\tau(B_{\rm tag})\)
- Unit:
ps
- mcLBoost#
Returns the MC vertex component in the boost direction
- Unit:
cm
- mcOBoost#
Returns the MC vertex component in the direction orthogonal to the boost
- Unit:
cm
- mcTagVLBoost#
Returns the MC TagV component in the boost direction
- Unit:
cm
- mcTagVOBoost#
Returns the MC TagV component in the direction orthogonal to the boost
- Unit:
cm
- mcTagVx#
Generated Tag vertex X component
- Unit:
cm
- mcTagVy#
Generated Tag vertex Y component
- Unit:
cm
- mcTagVz#
Generated Tag vertex Z component
- Unit:
cm
- tSigB#
Returns the proper decay time of the fully reconstructed signal B meson. The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
ps
- tTagB#
Returns the proper decay time of the tagged B meson. The result is meaningful and nontrivial when the signal B vertex is determined by
vertex.treeFit
withipConstraint=True
and thevertex.TagV
is called with thetube
constraint.- Unit:
ps
Flavor Tagger#
Analysis variables#
- isRelatedRestOfEventB0Flavor#
Returns -1 (1) if the RestOfEvent related to the given particle is related to a
anti-B0
(B0
). TheMCError
bit of Breco has to be 0, 1, 2, 16 or 1024. The output of the variable is 0 otherwise. If one particle in the RestOfEvent is found to belong to the reconstructedB0
, the output is -2(2) for aanti-B0
(B0
) on the reconstructed side.
- qOutput(combinerMethod)#
Returns the flavor tag \(q\) output of the flavorTagger for the given combinerMethod. The available methods are ‘FBDT’ and ‘FANN’ (category-based combiners), and ‘DNN’ (DNN tagger output).
Warning
You have to run the Flavor Tagger for this variable to be meaningful.
See also
- qrOutput(combinerMethod)#
Returns the output of the flavorTagger, flavor tag \(q\) times the dilution factor \(r\), for the given combiner method. The available methods are ‘FBDT’ and ‘FANN’ (category-based combiners), and ‘DNN’ (DNN tagger output).
Warning
You have to run the Flavor Tagger for this variable to be meaningful.
See also
- rBinBelle(combinerMethod)#
Returns the corresponding \(r\) (dilution) bin according to the Belle binning for the given
combinerMethod
. The available methods are ‘FBDT’ and ‘FANN’ (category-based combiners), and ‘DNN’ (DNN tagger output). The return values and the corresponding dilution ranges are the following:0: \(0.000 < r < 0.100\);
1: \(0.100 < r < 0.250\);
2: \(0.250 < r < 0.500\);
3: \(0.500 < r < 0.625\);
4: \(0.625 < r < 0.750\);
5: \(0.750 < r < 0.875\);
6: \(0.875 < r < 1.000\).
Warning
You have to run the Flavor Tagger for this variable to be meaningful.
See also
Training and expert variables#
- BtagToWBosonVariables(requestedVariable[, maskName])#
[Eventbased][Expert] Returns values of FlavorTagging-specific kinematical variables assuming a semileptonic decay with the given particle as target. The input values of
requestedVariable
can be the following: recoilMass in GeV/c^2 , pMissCMS inGeV/c
, cosThetaMissCMS and EW90.
- FSCVariables(requestedVariable)#
[Eventbased][Expert] Returns values of FlavorTagging-specific kinematical variables for
FastSlowCorrelated
category. The input values ofrequestedVariable
can be the following: pFastCMS inGeV/c
, cosSlowFast, SlowFastHaveOpositeCharges, or cosTPTOFast.
- HighestProbInCat(particleListName, extraInfoName)#
[Expert] Returns the highest target track probability value for the given category, for allowed input values for
extraInfoName
seehasHighestProbInCat
.
- KaonPionVariables(requestedVariable)#
[Expert] Returns values of FlavorTagging-specific kinematical variables for
KaonPion
category. The input values ofrequestedVariable
can be the following: cosKaonPion, HaveOpositeCharges.
- NumberOfKShortsInRoe#
[Expert] Returns the number of
K_S0
in the rest of event. The particle listK_S0:inRoe
has to be filled beforehand.
- QpOf(particleListName, outputExtraInfo, rankingExtraInfo)#
[Eventbased][Expert] Returns the \(q*p\) value for a given particle list provided as the 1st argument, where \(p\) is the probability of a category stored as extraInfo, provided as the 2nd argument, allowed values are same as in
hasHighestProbInCat
. The particle is selected after ranking according to a flavor tagging extraInfo, provided as the 3rd argument, allowed values are same as inhasHighestProbInCat
.
- QpTrack(particleListName, outputExtraInfo, rankingExtraInfo)#
[Expert] Returns the \(q*p\) value of the particle in a given particle list provided as the 1st argument that is originated from the same Track of given particle. where \(p\) is the probability of a category stored as extraInfo, provided as the 2nd argument, allowed values are same as in
hasHighestProbInCat
. The particle is selected after ranking according to a flavor tagging extraInfo, provided as the 3rd argument, allowed values are same as inhasHighestProbInCat
.
- ancestorHasWhichFlavor#
[Expert] Checks the decay chain of the given particle upwards up to the
Upsilon(4S)
resonance and outputs 0 (1) if an ancestor is found to be aanti-B0
(B0
), if not -2.
- chargeTimesKaonLiklihood#
[Expert] Returns
q*(highest PID_Likelihood for Kaons)
, 0. otherwise.
- cosTPTO
[Expert] Returns cosine of angle between thrust axis of given particle and thrust axis of ROE.
- cosTPTO(maskName)#
[Expert] Returns cosine of angle between thrust axis of given particle and thrust axis of ROE.
- hasHighestProbInCat(particleListName, extraInfoName)#
[Expert] Returns 1.0 if the given Particle is classified as target track, i.e. if it has the highest target track probability in particleListName. The probability is accessed via
extraInfoName
, which can have the following input values:isRightTrack(Electron),
isRightTrack(IntermediateElectron),
isRightTrack(Muon),
isRightTrack(IntermediateMuon),
isRightTrack(KinLepton),
isRightTrack(IntermediateKinLepton),
isRightTrack(Kaon),
isRightTrack(SlowPion),
isRightTrack(FastHadron),
isRightTrack(MaximumPstar),
isRightTrack(Lambda),
isRightCategory(Electron),
isRightCategory(IntermediateElectron),
isRightCategory(Muon),
isRightCategory(IntermediateMuon),
isRightCategory(KinLepton),
isRightCategory(IntermediateKinLepton),
isRightCategory(Kaon),
isRightCategory(SlowPion),
isRightCategory(FastHadron),
isRightCategory(MaximumPstar),
isRightCategory(Lambda),
isRightCategory(KaonPion),
isRightCategory(FSC).
- hasRestOfEventTracks
[Expert] Returns the number of tracks in the RestOfEvent related to the given Particle. -2 if the RestOfEvent is empty.
- hasRestOfEventTracks(maskName)#
[Expert] Returns the number of tracks in the RestOfEvent related to the given Particle. -2 if the RestOfEvent is empty.
- hasTrueTarget(categoryName)#
[Expert] Returns 1 if the given category has a target, 0 otherwise.
- hasTrueTargets(categoryName)#
[Expert] Returns 1 if target particles (checking only the decay chain) of the category with the given name is found in the MC particles. The allowed categories are the official Flavor Tagger Category Names.
- isInElectronOrMuonCat#
[Expert] Returns 1.0 if the particle has been selected as target in the Muon or Electron Category, 0.0 otherwise.
- isLambda#
[Expert] Returns 1.0 if particle is truth-matched to
Lambda0
, 0.0 otherwise.
- isMajorityInRestOfEventFromB0
[Eventbased][Expert] Checks if the majority of the tracks in the current RestOfEvent are from a
B0
.
- isMajorityInRestOfEventFromB0(maskName)#
[Eventbased][Expert] Checks if the majority of the tracks in the current RestOfEvent are from a
B0
.
- isMajorityInRestOfEventFromB0bar
[Eventbased][Expert] Check if the majority of the tracks in the current RestOfEvent are from a
anti-B0
.
- isMajorityInRestOfEventFromB0bar(maskName)#
[Eventbased][Expert] Check if the majority of the tracks in the current RestOfEvent are from a
anti-B0
.
- isRelatedRestOfEventMajorityB0Flavor
[Expert] Returns 0 (1) if the majority of tracks and clusters of the RestOfEvent related to the given Particle are related to a
anti-B0
(B0
).
- isRelatedRestOfEventMajorityB0Flavor(maskName)#
[Expert] Returns 0 (1) if the majority of tracks and clusters of the RestOfEvent related to the given Particle are related to a
anti-B0
(B0
).
- isRestOfEventMajorityB0Flavor#
[Expert] Returns 0 (1) if the majority of tracks and clusters of the current RestOfEvent are related to a
anti-B0
(B0
).
- isRightCategory(particleName)#
[Expert] Returns 1.0 if the class track by
particleName
category has the same flavor as the MC target track, 0.0 otherwise. Allowed input values forparticleName
argument in this variable are the following:Electron,
IntermediateElectron,
Muon,
IntermediateMuon,
KinLepton,
IntermediateKinLepton
Kaon,
SlowPion,
FastHadron,
KaonPion,
MaximumPstar,
FSC,
Lambda,
mcAssociated.
- isRightTrack(particleName)#
[Expert] Returns 1.0 if the given particle was really from a B-meson depending on category provided in
particleName
argument, 0.0 otherwise. Allowed input values forparticleName
argument in this variable are the following:Electron,
IntermediateElectron,
Muon,
IntermediateMuon,
KinLepton,
IntermediateKinLepton,
Kaon,
SlowPion,
FastHadron,
Lambda,
mcAssociated.
- isTrueCategory(categoryName)#
[Expert] Returns 1 if the given category tags the B0 MC flavor correctly, 0 otherwise.
- isTrueFTCategory(categoryName)#
[Expert] Returns 1 if the target particle (checking the decay chain) of the category with the given name is found in the MC particles, and if it provides the right flavor. The allowed categories are the official Flavor Tagger Category Names.
- lambdaFlavor#
[Expert] Returns 1.0 if particle is
Lambda0
, -1.0 in case ofanti-Lambda0
, 0.0 otherwise.
- lambdaZError#
[Expert] Returns the variance of the z-component of the decay vertex.
- Unit:
\(\text{cm}^2\)
- mcFlavorOfOtherB#
[Expert] Returns the MC flavor (+1 or -1) of the accompanying tag-side B meson if the given particle is a correctly truth-matched B candidate, 0 otherwise. In other words, this variable checks the generated flavor of the other generated
Upsilon(4S)
daughter.
- momentumOfSecondDaughter#
[Expert] Returns the momentum of second daughter if exists, 0. otherwise.
- Unit:
GeV/c
- momentumOfSecondDaughterCMS#
[Expert] Returns the momentum of the second daughter in the centre-of-mass system, 0. if this daughter doesn’t exist.
- Unit:
GeV/c
- pMissTag
[Expert] Calculates the missing momentum for a given particle on the tag side.
- Unit:
GeV/c
- pMissTag(maskName)#
[Expert] Calculates the missing momentum for a given particle on the tag side. The unit of the missing momentum is
GeV/c
- pt2TracksRoe(maskName)#
[Expert] Returns the transverse momentum squared of all charged tracks of the ROE related to the given particle, 0.0 if particle has no related ROE. The unit of the momentum squared is \([\text{GeV}/\text{c}]^2\)
- ptTracksRoe
[Expert] Returns the transverse momentum of all charged tracks of the ROE related to the given particle, 0.0 if particle has no related ROE.
- Unit:
GeV/c
- ptTracksRoe(maskName)#
[Expert] Returns the transverse momentum of all charged tracks of the ROE related to the given particle, 0.0 if particle has no related ROE. The unit of the momentum is
GeV/c
- qpCategory(categoryName)#
[Expert] Returns the output \(q\) (charge of target track) times \(p\) (probability that this is the right category) of the category with the given name. The allowed categories are the official Flavor Tagger Category Names.
- qrCombined#
[Eventbased][Expert] Returns -1 (1) if current RestOfEvent is related to a
anti-B0
(B0
). TheMCError
bit of Breco has to be 0, 1, 2, 16 or 1024. The output of the variable is 0 otherwise. If one particle in the RestOfEvent is found to belong to the reconstructedB0
, the output is -2(2) for aanti-B0
(B0
) on the reconstructed side.
- variableOfTarget(particleListName, inputVariable, rankingExtraInfo)#
[Eventbased][Expert] Returns the value of an input variable provided as the 2nd argument for a particle selected from the given list provided as the 1st argument. The particles are ranked according to a flavor tagging extraInfo, provided as the 2nd argument, allowed values are same as in
hasHighestProbInCat
.
- weightedQpOf(particleListName, outputExtraInfo, rankingExtraInfo)#
[Eventbased][Expert] Returns the weighted \(q*p\) value for a given particle list, provided as the 1st argument, where \(p\) is the probability of a category stored as extraInfo, provided in the 2nd argument, allowed values are same as in
hasHighestProbInCat
. The particles in the list are ranked according to a flavor tagging extraInfo, provided as the 3rd argument, allowed values are same as inhasHighestProbInCat
. The values for the three top particles is combined into an effective (weighted) output.
Rest of Event#
- bssMassDifference(maskName)#
Bs* - Bs mass difference. The unit of the mass is \(\text{GeV/c}^2\).
- currentROEIsInList(particleList)#
[Eventbased] Returns 1 the associated particle of the current ROE is contained in the given list or its charge-conjugated.Useful to restrict the for_each loop over ROEs to ROEs of a certain ParticleList.
- etaProb(mode)#
Returns eta probability, where mode is used to specify the selection criteria for soft photon. The following strings are available.
standard
: loose energy cut and no clusterNHits cut are applied to soft photontight
: tight energy cut and no clusterNHits cut are applied to soft photoncluster
: loose energy cut and clusterNHits cut are applied to soft photonboth
: tight energy cut and clusterNHits cut are applied to soft photon
You can find more details in
writePi0EtaVeto
function in modularAnalysis.py.
- hasAncestorFromSignalSide#
Returns 1 if a particle has ancestor from signal side, 0 otherwise. Requires generator information and truth-matching. One can use this variable only in a
for_each
loop over the RestOfEvent StoreArray.
- hasCorrectROECombination#
Returns 1 if there is correct combination of daughter particles between the particle that is the basis of the ROE and the particle loaded from the ROE. Returns 0 if there is not correct combination. If there is no daughter particle loaded from the ROE, returns quiet NaN.
- isCloneOfSignalSide#
Returns 1 if a particle is a clone of signal side final state particles, 0 otherwise. Requires generator information and truth-matching. One can use this variable only in a
for_each
loop over the RestOfEvent StoreArray.
- isInRestOfEvent#
Returns 1 if a track, ecl or klmCluster associated to particle is in the current RestOfEvent object, 0 otherwise.One can use this variable only in a for_each loop over the RestOfEvent StoreArray.
- nROE_Charged(maskName, PDGcode = 0)#
Returns number of all charged particles in the related RestOfEvent object. First optional argument is ROE mask name. Second argument is a PDG code to count only one charged particle species, independently of charge. For example:
nROE_Charged(cleanMask, 321)
will output number of kaons in Rest Of Event withcleanMask
. PDG code 0 is used to count all charged particles
- nROE_Composites(maskName)#
Returns number of composite particles or V0s in the related RestOfEvent object that pass the selection criteria.
- nROE_ECLClusters(maskName)#
Returns number of ECL clusters in the related RestOfEvent object that pass the selection criteria.
- nROE_KLMClusters#
Returns number of all remaining KLM clusters in the related RestOfEvent object.
- nROE_NeutralECLClusters(maskName)#
Returns number of neutral ECL clusters in the related RestOfEvent object that pass the selection criteria.
- nROE_NeutralHadrons(maskName)#
Returns number of all neutral hadrons in the related RestOfEvent object, accepts 1 optional argument of ROE mask name.
- nROE_ParticlesInList(pListName[, maskName])#
Returns the number of particles in ROE from the given particle list. If a mask name is provided the selection criteria are applied. Use of variable aliases is advised.
- nROE_Photons(maskName)#
Returns number of all photons in the related RestOfEvent object, accepts 1 optional argument of ROE mask name.
- nROE_RemainingTracks#
Returns number of tracks in ROE - number of tracks of given particleOne can use this variable only in a for_each loop over the RestOfEvent StoreArray.
- nROE_RemainingTracks(maskName)
Returns number of remaining tracks between the ROE (specified via a mask) and the given particle. For the given particle only tracks are counted which are in the RoE.One can use this variable only in a for_each loop over the RestOfEvent StoreArray.Is required for the specific FEI.
- nROE_Tracks(maskName)#
Returns number of tracks in the related RestOfEvent object that pass the selection criteria.
- particleRelatedToCurrentROE(var)#
[Eventbased] Returns variable applied to the particle which is related to the current RestOfEvent objectOne can use this variable only in a for_each loop over the RestOfEvent StoreArray.
- passesROEMask(maskName)#
Returns boolean value if a particle passes a certain mask or not. Only to be used in for_each path, otherwise returns quiet NaN.
- pi0Prob(mode)#
Returns pi0 probability, where mode is used to specify the selection criteria for soft photon. The following strings are available.
standard
: loose energy cut and no clusterNHits cut are applied to soft photontight
: tight energy cut and no clusterNHits cut are applied to soft photoncluster
: loose energy cut and clusterNHits cut are applied to soft photonboth
: tight energy cut and clusterNHits cut are applied to soft photon
You can find more details in
writePi0EtaVeto
function in modularAnalysis.py.
- printROE#
For debugging, prints indices of all particles in the ROE and all masks. Returns 0.
- roeCharge(maskName)#
Returns total charge of the related RestOfEvent object. The unit of the charge is
e
- roeDeltae(maskName)#
Returns energy difference of the related RestOfEvent object with respect to \(E_\mathrm{cms}/2\). The unit of the energy is
GeV
- roeE(maskName)#
Returns energy of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the energy isGeV
- roeEextra(maskName)#
Returns extra energy from ECLClusters in the calorimeter that is not associated to the given Particle. The unit of the energy is
GeV
- roeM(maskName)#
Returns invariant mass of unused tracks and clusters in ROE. The unit of the invariant mass is \(\text{GeV/c}^2\)
- roeMC_E#
Returns true energy of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV
- roeMC_M#
Returns true invariant mass of unused tracks and clusters in ROE
- Unit:
GeV/\(\text{c}^2\)
- roeMC_MissFlags(maskName)#
Returns flags corresponding to missing particles on ROE side.
- roeMC_P#
Returns true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV/c
- roeMC_PTheta#
Returns polar angle of true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
rad
- roeMC_Pt#
Returns transverse component of true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV/c
- roeMC_Px#
Returns x component of true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV/c
- roeMC_Py#
Returns y component of true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV/c
- roeMC_Pz#
Returns z component of true momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function.- Unit:
GeV/c
- roeMbc(maskName)#
Returns beam constrained mass of the related RestOfEvent object with respect to \(E_\mathrm{cms}/2\). The unit of the beam constrained mass is \(\text{GeV/c}^2\).
- roeNeextra(maskName)#
Returns extra energy from neutral ECLClusters in the calorimeter that is not associated to the given Particle, can be used with
use***Frame()
function. The unit of the energy isGeV
- roeP(maskName)#
Returns momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the momentum isGeV/c
- roePTheta(maskName)#
Returns theta angle of momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the angle israd
- roePt(maskName)#
Returns transverse component of momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the momentum isGeV/c
- roePx(maskName)#
Returns x component of momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the momentum isGeV/c
- roePy(maskName)#
Returns y component of momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the momentum isGeV/c
- roePz(maskName)#
Returns z component of momentum of unused tracks and clusters in ROE, can be used with
use***Frame()
function. The unit of the momentum isGeV/c
- useROERecoilFrame(variable)#
Returns the value of the variable using the rest frame of the ROE recoil as current reference frame. Can be used inside for_each loop or outside of it if the particle has associated Rest of Event. E.g.
useROERecoilFrame(E)
returns the energy of a particle in the ROE recoil frame.
- weCosThetaEll(maskName)#
Returns the cosine of the angle between \(M\) and lepton in \(W\) rest frame in the decays of the type: \(M \to h_1 ... h_n \ell\), where W 4-momentum is given as
\[p_W = p_\ell + p_\nu.\]The neutrino momentum is calculated from ROE taking into account the specified mask, and setting
\[E_{\nu} = |p_{miss}|.\]
- weDeltae(maskName, opt)#
Returns the energy difference of the B meson, corrected with the missing neutrino momentum (reconstructed side + neutrino) with respect to \(E_\mathrm{cms}/2\). The unit of the energy is
GeV
- weMbc(maskName, opt)#
Returns beam constrained mass of B meson, corrected with the missing neutrino momentum (reconstructed side + neutrino) with respect to \(E_\mathrm{cms}/2\). The unit of the beam constrained mass is \(\text{GeV/c}^2\).
- weMissE(maskName, opt)#
Returns the energy of the missing momentum. The unit of the Energy is
GeV
. Possible optionsopt
are the following:0
: CMS, use energy and momentum of charged particles and photons1
: CMS, same as0
, fix \(E_\mathrm{miss} = p_\mathrm{miss}\)2
: CMS, same as0
, fix \(E_\mathrm{roe} = E_\mathrm{cms}/2\)3
: CMS, use only energy and momentum of signal side4
: CMS, same as3
, update with direction of ROE momentum5
: LAB, use energy and momentum of charged particles and photons from whole event6
: LAB, same as5
, fix \(E_\mathrm{miss} = p_\mathrm{miss}`\)7
: CMS, correct pmiss 3-momentum vector with factor alpha so that \(d_E = 0`\) (used for \(M_\mathrm{bc}\) calculation).
- weMissM2(maskName, opt)#
Returns the invariant mass squared of the missing momentum (see
weMissE
possible options). The unit of the invariant mass squared is \([\text{GeV}/\text{c}^2]^2\).
- weMissM2OverMissE(maskName)#
Returns missing mass squared over missing energy. The unit of the missing mass squared is \(\text{GeV/c}^4\).
- weMissP(maskName, opt)#
Returns the magnitude of the missing momentum (see possible
weMissE
options). The unit of the magnitude of missing momentum isGeV/c
- weMissPTheta(maskName, opt)#
Returns the polar angle of the missing momentum (see possible
weMissE
options). The unit of the polar angle israd
- weMissPx(maskName, opt)#
Returns the x component of the missing momentum (see
weMissE
possible options). The unit of the missing momentum isGeV/c
- weMissPy(maskName, opt)#
Returns the y component of the missing momentum (see
weMissE
possible options). The unit of the missing momentum isGeV/c
- weMissPz(maskName, opt)#
Returns the z component of the missing momentum (see
weMissE
possible options). The unit of the missing momentum isGeV/c
- weQ2lnu(maskName, option)#
Returns the momentum transfer squared, \(q^2\), calculated in CMS as \(q^2 = (p_l + p_\nu)^2\), where \(B \to H_1\dots H_n \ell \nu_\ell\). Lepton is assumed to be the last reconstructed daughter. This calculation uses constraints from dE = 0 and Mbc = Mb to correct the neutrino direction. By default, option is set to
7
(seeweMissE
). Unless you know what you are doing, keep this default value. The unit of the momentum transfer squared is \([\text{GeV}/\text{c}]^2\).
- weQ2lnuSimple(maskName, option)#
Returns the momentum transfer squared, \(q^2\), calculated in CMS as \(q^2 = (p_l + p_\nu)^2\), where \(B \to H_1\dots H_n \ell \nu_\ell\). Lepton is assumed to be the last reconstructed daughter. By default, option is set to
1
(seeweMissE
). Unless you know what you are doing, keep this default value. The unit of the momentum transfer squared is \([\text{GeV}/\text{c}]^2\).
- weXiZ(maskName)#
Returns Xi_z in event (for Bhabha suppression and two-photon scattering). The unit of this variable is
1/c
Continuum Suppression#
For a detailed description of the continuum suppression, see Continuum suppression.
All variables in this group require the ContinuumSuppressionBuilder
module to be added to the path,
and a rest of event for the candidate particles. This can be done with both the
modularAnalysis.buildRestOfEvent
, and the modularAnalysis.buildContinuumSuppression
convenience functions.
- CleoConeCS(integer[, string, string])#
Returns i-th cleo cones from the continuum suppression. The allowed inputs for the
integer
argument are integers from 1 to 9. The second and third arguments are optional unless you have created multiple instances of the ContinuumSuppression with different ROE masks. In that case the desired ROE mask name must be provided as well. If the second argument is set to ‘ROE’, the CleoCones are calculated only from ROE particles. Otherwise, the CleoCones are calculated from all final state particles. The ROE mask name is then either the second or the third argument and must not be called ‘ROE’. The unit of the CleoConeCS isGeV/c
.Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- KSFWVariables(variable[, string, string])#
Returns variable et in
GeV/c
, mm2 in (GeV/c^2)^2, or one of the 16 KSFW moments. The second and third arguments are optional unless you have created multiple instances of the ContinuumSuppression with different ROE masks. In that case the desired ROE mask name must be provided as well. If the second argument is set to ‘FS1’, the KSFW moment is calculated from the B final state daughters. Otherwise, the KSFW moment is calculated from the B primary daughters. The ROE mask name is then either the second or the third argument and must not be called ‘FS1’. Allowed input values forvariable
argument are the following:mm2, et
hso00, hso01, hso02, hso03, hso04
hso10, hso12, hso14
hso20, hso22, hso24
hoo0, hoo1, hoo2, hoo3, hoo4.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- R2
Returns reduced Fox-Wolfram R2, defined as ratio of the i-th to the 0-th order Fox Wolfram moments.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- R2(maskname)#
Returns reduced Fox-Wolfram R2, defined as ratio of the i-th to the 0-th order Fox Wolfram moments.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- cosTBTO
Returns cosine of angle between thrust axis of the signal B and thrust axis of ROE.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- cosTBTO(maskname)#
Returns cosine of angle between thrust axis of the signal B and thrust axis of ROE.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- cosTBz
Returns cosine of angle between thrust axis of the signal B and z-axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- cosTBz(maskname)#
Returns cosine of angle between thrust axis of the signal B and z-axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- thrustBm
Returns magnitude of the signal B thrust axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- thrustBm(maskname)#
Returns magnitude of the signal B thrust axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- thrustOm
Returns magnitude of the ROE thrust axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- thrustOm(maskname)#
Returns magnitude of the ROE thrust axis.
Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
- transformedNetworkOutput(name, low, high)#
Transforms the network output \(C \to C'\) via: \(C'=\operatorname{log}((C-\mathrm{low})/(\mathrm{high}-C))\). The arguments of the metavariable are the following:
name
is theextraInfo
name, where the network output \(C\) has been stored. If particle is not specified, eventextraInfo
is used instead;low
,high
are floating point numbers.
Returns NaN, if the
extraInfo
has not been found.
- useBThrustFrame(variable, mode)#
Returns the variable with respect to rotated coordinates, in which z lies on the specified thrust axis. If mode is set to
Signal
it will use the thrust axis of the reconstructed B candidate, if mode is set to ROE it will use the ROE thrust axis. If mode is set toAuto
the function use the thrust axis based on Rest Of Event (ROE) particles. LikeisInRestOfEvent
, one has to use this metavariable in ROE loop.Warning
You have to run the Continuum Suppression builder module for this variable to be meaningful.
See also
Event Shape#
These variables are available after adding the event shape builder modules.
This can be done with the function modularAnalysis.buildEventShape
.
For a detailed description of the event shape variables, see EventShape
- aplanarity#
[Eventbased] Event aplanarity, defined as the 3/2 of the third sphericity eigenvalue.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- backwardHemisphereEnergy#
[Eventbased] Total energy of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV
- backwardHemisphereMass#
[Eventbased] Invariant mass of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/\(\text{c}^2\)
- backwardHemisphereMomentum#
[Eventbased] Total momentum of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- backwardHemisphereX#
[Eventbased] X component of the total momentum of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- backwardHemisphereY#
[Eventbased] Y component of the total momentum of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- backwardHemisphereZ#
[Eventbased] Z component of the total momentum of the particles flying in the direction opposite to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- cleoCone(i, axisName)#
[Eventbased] Returns i-th order Cleo cone, calculated with respect to the axis
axisName
. The orderi
can go from 0 up to 8th, theaxisName
can be either ‘thrust’ or ‘collision’.Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust0#
[Eventbased] 0th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust1#
[Eventbased] 1st Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust2#
[Eventbased] 2nd Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust3#
[Eventbased] 3rd Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust4#
[Eventbased] 4th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust5#
[Eventbased] 5th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust6#
[Eventbased] 6th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust7#
[Eventbased] 7th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- cleoConeThrust8#
[Eventbased] 8th Cleo cone calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- forwardHemisphereEnergy#
[Eventbased] Total energy of the particles flying in the same direction as the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV
- forwardHemisphereMass#
[Eventbased] Invariant mass of the particles flying in the same direction as the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/\(\text{c}^2\)
- forwardHemisphereMomentum#
[Eventbased] Total momentum of the particles flying in the same direction as the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- forwardHemisphereX#
[Eventbased] X component of the total momentum of the particles flying in the same direction as the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- forwardHemisphereY#
[Eventbased] Y component of the total momentum of the particles flying in the same direction as the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- forwardHemisphereZ#
[Eventbased] Z component of the total momentum of the particles flying in the same direction of the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- foxWolframH(i)#
[Eventbased] Returns i-th order Fox Wolfram moment. The order
i
can go from 0 up to 8th.”Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
\(\text{GeV}^2\)/\(\text{c}^2\)
- foxWolframR(i)#
[Eventbased] Ratio of the i-th to the 0-th order Fox Wolfram moments. The order
i
can go from 0 up to 8th.Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- foxWolframR1#
[Eventbased] Ratio of the 1-st to the 0-th order Fox Wolfram moments. This is just an alias of foxWolframR(1) defined for the user’s convenience.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- foxWolframR2#
[Eventbased] Ratio of the 2-nd to the 0-th order Fox Wolfram moments. This is just an alias of foxWolframR(2) defined for the user’s convenience.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- foxWolframR3#
[Eventbased] Ratio of the 3-rd to the 0-th order Fox Wolfram moments. This is just an alias of foxWolframR(3) defined for the user’s convenience.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- foxWolframR4#
[Eventbased] Ratio of the 4-th to the 0-th order Fox Wolfram moments. This is just an alias of foxWolframR(4) defined for the user’s convenience.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- harmonicMoment(i, axisName)#
[Eventbased] Returns i-th order harmonic moment, calculated with respect to the axis
axisName
. The orderi
can go from 0 up to 8th, theaxisName
can be either ‘thrust’ or ‘collision’. The unit of the harmonic moment isGeV/c
.Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- harmonicMomentThrust0#
[Eventbased] Harmonic moment of the 0th order calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- harmonicMomentThrust1#
[Eventbased] Harmonic moment of the 1st order calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- harmonicMomentThrust2#
[Eventbased] Harmonic moment of the 2nd order calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- harmonicMomentThrust3#
[Eventbased] Harmonic moment of the 3rd order calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- harmonicMomentThrust4#
[Eventbased] Harmonic moment of the 4th order calculated with respect to the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- sphericity#
[Eventbased] Event sphericity, defined as the linear combination of the sphericity eigenvalues \(\lambda_i\): \(S = (3/2)(\lambda_2+\lambda_3)\).
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- thrust#
[Eventbased] Event thrust.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- thrustAxisCosTheta#
[Eventbased] Cosine of the polar angle component of the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- thrustAxisX#
[Eventbased] X component of the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- thrustAxisY#
[Eventbased] Y component of the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- thrustAxisZ#
[Eventbased] Z component of the thrust axis.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
- useThrustFrame(variable)#
Evaluates a variable value in the thrust reference frame.
Warning
You have to run the Event Shape builder module for this variable to be meaningful.
See also
Event Kinematics#
These variables are available after adding the event kinematics module.
This can be done with the function modularAnalysis.buildEventKinematics
.
The variable collection event_kinematics
allows to add all of them comfortably to your ntuple.
- genMissingEnergyOfEventCMS#
[Eventbased] The missing energy in center-of-mass frame from generator.
- Unit:
GeV
- genMissingMass2OfEvent#
[Eventbased] The missing mass squared from generator
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- genMissingMomentumOfEventCMS#
[Eventbased] The magnitude of the missing momentum in center-of-mass frame from generator
- Unit:
GeV/c
- genTotalPhotonsEnergyOfEvent#
[Eventbased] The energy in laboratory frame of all the photons. from generator.
- Unit:
GeV
- genVisibleEnergyOfEventCMS#
[Eventbased] The visible energy in center-of-mass frame from generator.
- Unit:
GeV
- missingEnergyOfEventCMS#
[Eventbased] The missing energy in center-of-mass frame.
- Unit:
GeV
- missingMass2OfEvent#
[Eventbased] The missing mass squared.
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- missingMomentumOfEvent#
[Eventbased] The magnitude of the missing momentum in laboratory frame.
Warning
You have to run the Event Kinematics builder module for this variable to be meaningful.
See also
- Unit:
GeV/c
- missingMomentumOfEventCMS#
[Eventbased] The magnitude of the missing momentum in center-of-mass frame.
- Unit:
GeV/c
- missingMomentumOfEventCMS_Px#
[Eventbased] The x component of the missing momentum in center-of-mass frame.
- Unit:
GeV/c
- missingMomentumOfEventCMS_Py#
[Eventbased] The y component of the missing momentum in center-of-mass frame.
- Unit:
GeV/c
- missingMomentumOfEventCMS_Pz#
[Eventbased] The z component of the missing momentum in center-of-mass frame.
- Unit:
GeV/c
- missingMomentumOfEventCMS_theta#
[Eventbased] The theta angle of the missing momentum in center-of-mass frame.
- Unit:
rad
- missingMomentumOfEvent_Px#
[Eventbased] The x component of the missing momentum in laboratory frame.
- Unit:
GeV/c
- missingMomentumOfEvent_Py#
[Eventbased] The y component of the missing momentum in laboratory frame.
- Unit:
GeV/c
- missingMomentumOfEvent_Pz#
[Eventbased] The z component of the missing momentum in laboratory frame.
- Unit:
GeV/c
- missingMomentumOfEvent_theta#
[Eventbased] The theta angle of the missing momentum of the event in laboratory frame.
- Unit:
rad
- totalPhotonsEnergyOfEvent#
[Eventbased] The energy in laboratory frame of all the photons.
- Unit:
GeV
- visibleEnergyOfEventCMS#
[Eventbased] The visible energy in center-of-mass frame.
- Unit:
GeV
Flight Information#
Here is a list of flight time and distance variables of a (grand)daughter particle w.r.t. its (grand)mother decay vertex:
- flightDistance#
Returns the flight distance of particle. If a treeFit has been performed the flight distance calculated by TreeFitter is returned. Otherwise if a beam constrained rave fit has been performed the production vertex set by rave and the decay vertex are used to calculate the flight distance. If neither fit has been performed the i.p. is taken to be the production vertex.
- Unit:
cm
- flightDistanceErr#
Returns the flight distance error of particle. If a treeFit has been performed the flight distance error calculated by TreeFitter is returned. Otherwise if a beam constrained rave fit has been performed the production vertex set by rave and the decay vertex are used to calculate the flight distance error. If neither fit has been performed the i.p. is taken to be the production vertex.
- Unit:
cm
- flightDistanceOfDaughter(daughterN, gdaughterN = -1)#
Returns the flight distance between mother and daughter particle with daughterN index. If a treeFit has been performed the value calculated by treeFitter is returned. Otherwise the value is calculated using the decay vertices of the mother and daughter particle. If a second index granddaughterM is given the value is calculated between the mother and the Mth grandaughter (Mth daughter of Nth daughter).
- Unit:
cm
- flightDistanceOfDaughterErr(daughterN, gdaughterN = -1)#
Returns the flight distance error between mother and daughter particle with daughterN index. If a treeFit has been performed the value calculated by treeFitter is returned. Otherwise the value is calculated using the decay vertices of the mother and daughter particle. If a second index granddaughterM is given the value is calculated between the mother and the Mth grandaughter (Mth daughter of Nth daughter).
- Unit:
cm
- flightTime#
Returns the flight time of particle. If a treeFit has been performed the flight time calculated by TreeFitter is returned. Otherwise if a beam constrained rave fit has been performed the production vertex set by rave and the decay vertex are used to calculate the flight time. If neither fit has been performed the i.p. is taken to be the production vertex.
- Unit:
ns
- flightTimeErr#
Returns the flight time error of particle. If a treeFit has been performed the flight time error calculated by TreeFitter is returned. Otherwise if a beam constrained rave fit has been performed the production vertex set by rave and the decay vertex are used to calculate the flight time error. If neither fit has been performed the i.p. is taken to be the production vertex.
- Unit:
ns
- flightTimeOfDaughter(daughterN, gdaughterN = -1)#
Returns the flight time between mother and daughter particle with daughterN index. If a treeFit has been performed the value calculated by treeFitter is returned. Otherwise the value is calculated using the decay vertices of the mother and daughter particle. If a second index granddaughterM is given the value is calculated between the mother and the Mth grandaughter (Mth daughter of Nth daughter).
- Unit:
ns
- flightTimeOfDaughterErr(daughterN, gdaughterN = -1)#
Returns the flight time error between mother and daughter particle with daughterN index. If a treeFit has been performed the value calculated by treeFitter is returned. Otherwise the value is calculated using the decay vertices of the mother and daughter particle. If a second index granddaughterM is given the value is calculated between the mother and the Mth grandaughter (Mth daughter of Nth daughter).
- Unit:
ns
- mcFlightDistance#
Returns the MC flight distance of the particle
- Unit:
cm
- mcFlightDistanceOfDaughter(daughterN, gdaughterN = -1)#
Returns the MC flight distance between mother and daughter particle using generated info
- Unit:
cm
- mcFlightTime#
Returns the MC flight time of the particle
- Unit:
ns
- mcFlightTimeOfDaughter(daughterN, gdaughterN = -1)#
Returns the MC flight time between mother and daughter particle using generated info
- Unit:
ns
- vertexDistance#
Returns the distance between the production and decay vertex of a particle. Returns NaN if particle has no production or decay vertex.
- Unit:
cm
- vertexDistanceErr#
Returns the uncertainty on the distance between the production and decay vertex of a particle. Returns NaN if particle has no production or decay vertex.
- Unit:
cm
- vertexDistanceOfDaughter(daughterN[, option])#
If any integer is provided as second argument it returns the distance between the decay vertices of the particle and of its daughter with index daughterN. Otherwise, it is assumed that the particle has a production vertex (typically the IP) which is used to calculate the distance to the daughter’s decay vertex. Returns NaN in case anything goes wrong.
- Unit:
cm
- vertexDistanceOfDaughterErr(daughterN[, option])#
If any integer is provided as second argument it returns the uncertainty on the distance between the decay vertices of the particle and of its daughter with index daughterN. Otherwise, it is assumed that the particle has a production vertex (typically the IP) with a corresponding covariance matrix to calculate the uncertainty on the distance to the daughter’s decay vertex. Returns NaN in case anything goes wrong.
- Unit:
cm
- vertexDistanceOfDaughterSignificance(daughterN[, option)#
If any integer is provided as second argument it returns the distance between the decay vertices of the particle and of its daughter with index daughterN in units of the uncertainty on this value. Otherwise, it is assumed that the particle has a production vertex (typically the IP) with a corresponding covariance matrix and the significance of the separation to this vertex is calculated.
- vertexDistanceSignificance#
Returns the distance between the production and decay vertex of a particle in units of the uncertainty on this value, i.e. the significance of the vertex separation.
Vertex Information#
Here is a list of production and decay vertex variables:
- dcosTheta#
vertex or POCA polar angle with respect to IP
- distance#
3D distance between the IP and the particle decay vertex, if available.
In case the particle has been created from a track, the distance is defined between the POCA and IP. If the particle is built from an ECL cluster, the decay vertex is set to the nominal IP. If the particle is created from a KLM cluster, the distance is calculated between the IP and the cluster itself.
- Unit:
cm
- dphi#
vertex azimuthal angle of the vertex or POCA in degrees with respect to IP
- Unit:
rad
- dr#
transverse distance with respect to IP for a vertex; track abs(d0) relative to IP for a track.
- Unit:
cm
- dx#
vertex or POCA in case of tracks x with respect to IP
- Unit:
cm
- dy#
vertex or POCA in case of tracks y with respect to IP
- Unit:
cm
- dz#
vertex or POCA in case of tracks z with respect to IP
- Unit:
cm
- mcDecayVertexFromIPDistance#
Returns the distance of the decay vertex of the matched generated particle from the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexFromIPRho#
Returns the transverse position of the decay vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexFromIPX#
Returns the x position of the decay vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexFromIPY#
Returns the y position of the decay vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexFromIPZ#
Returns the z position of the decay vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexRho#
Returns the transverse position of the decay vertex of the matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexX#
Returns the x position of the decay vertex of the matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexY#
Returns the y position of the decay vertex of the matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcDecayVertexZ#
Returns the z position of the decay vertex of the matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexFromIPX#
Returns the x position of the production vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexFromIPY#
Returns the y position of the production vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexFromIPZ#
Returns the z position of the production vertex of the matched generated particle wrt the IP. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexX#
Returns the x position of production vertex of matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexY#
Returns the y position of production vertex of matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- mcProductionVertexZ#
Returns the z position of production vertex of matched generated particle. Returns nan if the particle has no matched generated particle.
- Unit:
cm
- prodVertexCov(i, j)#
Returns the ij covariance matrix component of particle production vertex, arguments i,j should be 0, 1 or 2. Returns NaN if particle has no production covariance matrix.
- Unit:
\(\text{cm}^2\)
- prodVertexX#
Returns the x position of particle production vertex. Returns NaN if particle has no production vertex.
- Unit:
cm
- prodVertexXErr#
Returns the x position uncertainty of particle production vertex. Returns NaN if particle has no production vertex.
- Unit:
cm
- prodVertexY#
Returns the y position of particle production vertex.
- Unit:
cm
- prodVertexYErr#
Returns the y position uncertainty of particle production vertex.
- Unit:
cm
- prodVertexZ#
Returns the z position of particle production vertex.
- Unit:
cm
- prodVertexZErr#
Returns the z position uncertainty of particle production vertex.
- Unit:
cm
- significanceOfDistance#
significance of distance from vertex or POCA to interaction point(-1 in case of numerical problems)
- x#
x coordinate of vertex in case of composite particle, or point of closest approach (POCA) in case of a track
- Unit:
cm
- x_uncertainty#
uncertainty on x (measured with respect to the origin)
- Unit:
cm
- y#
y coordinate of vertex in case of composite particle, or point of closest approach (POCA) in case of a track
- Unit:
cm
- y_uncertainty#
uncertainty on y (measured with respect to the origin)
- Unit:
cm
- z#
z coordinate of vertex in case of composite particle, or point of closest approach (POCA) in case of a track
- Unit:
cm
- z_uncertainty#
uncertainty on z (measured with respect to the origin)
- Unit:
cm
KFit variables#
Here is a list of variables that indicate the quality of a KFit.
- FourCKFitChi2#
Chi2 of four momentum-constraint kinematical fit in KFit
- FourCKFitProb#
Prob of four momentum-constraint kinematical fit in KFit
- KFit_nTracks#
number of tracks used in the vertex KFit
- TracksLBoostChi2#
indicator of vertex KFit quality based on accumulated change of track positions
Orca Kinematic Fitter#
Warning
In order to populate these variables, you need to run the Orca kinematic fitter. I.e.
import kinfit
kinfit.fitKinematic4C(your_candidates, path=mypath) # or similar
- OrcaKinFitChi2#
The \(\chi^2\) returned by the Orca kinematic fitter. Returns NaN if Orca was not run.
Warning
this is the raw \(\chi^2\), not over ndf.
- OrcaKinFitErrorCode#
The error code returned by the Orca kinematic fitter. Returns NaN if Orca was not run.
- OrcaKinFitProb#
The p-value returned by the Orca kinematic fitter. Returns NaN if Orca was not run.
- OrcaKinFitUnmeasuredE#
The energy of the “unmeasured photon” returned by Orca kinematic fitter for specific 1C fits with the
addUnmeasuredPhoton
parameter set. I.e. for fits with sufficient constraints remaining to constrain a missing 3-vector. Returns NaN if Orca was not run or if theaddUnmeasuredPhoton
parameter was not set.- Unit:
GeV
- OrcaKinFitUnmeasuredErrorE#
The uncertainty on
OrcaKinFitUnmeasuredE
.- Unit:
GeV
- OrcaKinFitUnmeasuredErrorPhi#
The uncertainty on
OrcaKinFitUnmeasuredPhi
.- Unit:
rad
- OrcaKinFitUnmeasuredErrorTheta#
The uncertainty on
OrcaKinFitUnmeasuredTheta
.- Unit:
rad
- OrcaKinFitUnmeasuredPhi#
The azimuthal angle of the “unmeasured photon” returned by Orca kinematic fitter for specific 1C fits with the
addUnmeasuredPhoton
parameter set. I.e. for fits with sufficient constraints remaining to constrain a missing 3-vector. Returns NaN if Orca was not run or if theaddUnmeasuredPhoton
parameter was not set.- Unit:
rad
- OrcaKinFitUnmeasuredTheta#
The polar angle of the “unmeasured photon” returned by Orca kinematic fitter for specific 1C fits with the
addUnmeasuredPhoton
parameter set. I.e. for fits with sufficient constraints remaining to constrain a missing 3-vector. Returns NaN if Orca was not run or if theaddUnmeasuredPhoton
parameter was not set.- Unit:
rad
For fully-inclusive particles#
Here is a list of useful variables to work with fully-inclusive particles, which are produced via Rest Of Event, AllParticleCombiner
or other inclusive reconstruction modules:
- daughterAverageOf(variable)#
Returns the mean value of a variable over all daughters.
- nCompositeAllGenerationDaughters(pdg)#
Returns the number of all generations’ composite daughters with the provided PDG code or the numberof all generations’ composite daughters if no argument has been provided. The variable is flavor agnostic and it returns the sum of the number of particle and anti-particle.
- nCompositeDaughters(pdg)#
Returns the number of primary composite daughters with the provided PDG code or the numberof all primary composite daughters if no argument has been provided. The variable is flavor agnostic and it returns the sum of the number of particle and anti-particle.
- nDaughterCharged(pdg)#
Returns the number of charged daughters with the provided PDG code or the number of all charged daughters if no argument has been provided. The variable is flavor agnostic and it returns the sum of the number of particle and anti-particle.
- nDaughterNeutralHadrons#
Returns the number of K_L0 or neutrons among the final state daughters.
- nDaughterPhotons#
Returns the number of final state daughter photons.
Specific kinematic variables#
This variables group is reserved for the variables for analyses of specific decays like, \(B \to h l \nu\), \(B \to h l^\pm l^\mp\), etc.
- recMissM2#
Returns the invariant mass squared of the missing momentum calculated assumings the reco B is at rest and calculating the neutrino (missing) momentum from \(p_\nu = p_B - p_{\rm had} - p_{\rm lep}\)
- Unit:
\([\text{GeV}/\text{c}^2]^2\)
- recQ2Bh#
Returns the momentum transfer squared, \(q^2\), calculated in CMS as \(q^2 = (p_B - p_h)^2\), where p_h is the CMS momentum of all hadrons in the decay \(B \to H_1\dots H_n \ell \nu_\ell\). This calculation uses a weighted average of the B meson around the reco B cone. Based on diamond frame calculation of \(q^2\) following the idea presented in https://www.osti.gov/biblio/1442697 It will switch to use of
recQ2BhSimple
if absolute ofcosThetaBetweenParticleAndNominalB
> 1.- Unit:
\([\text{GeV}/\text{c}]^2\)
- recQ2BhSimple#
Returns the momentum transfer squared, \(q^2\), calculated in CMS as \(q^2 = (p_B - p_h)^2\), where p_h is the CMS momentum of all hadrons in the decay \(B \to H_1 ... H_n \ell \nu_\ell\). The B meson momentum in CMS is assumed to be 0.
- Unit:
\([\text{GeV}/\text{c}]^2\)
Belle and b2bii
variables#
Several legacy Belle variables are provided.
Note
These are intended for studies with b2bii
and for comparison between Belle and Belle II.
- BelleACCQuality#
[Legacy] Returns the ACC quality flag. Original definition in the panther tables: if 0 normal, if 1 the track is out of ACC acceptance. (Belle only).
- BelleACCnPe#
[Legacy] Returns the number of photo-electrons associated to the track in the ACC. (Belle only).
- BelleFirstCDCHitX#
[Legacy] Returns x component of starting point of the track near the 1st SVD or CDC hit for SVD1 data (exp. 7 - 27) or the 1st CDC hit for SVD2 data (from exp. 31). (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BelleFirstCDCHitY#
[Legacy] Returns y component of starting point of the track near the 1st SVD or CDC hit for SVD1 data (exp. 7 - 27) or the 1st CDC hit for SVD2 data (from exp. 31). (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BelleFirstCDCHitZ#
[Legacy] Returns z component of starting point of the track near the 1st SVD or CDC hit for SVD1 data (exp. 7 - 27) or the 1st CDC hit for SVD2 data (from exp. 31). (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BelleLastCDCHitX#
[Legacy] Returns x component of end point of the track near the last CDC hit. (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BelleLastCDCHitY#
[Legacy] Returns y component of end point of the track near the last CDC hit. (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BelleLastCDCHitZ#
[Legacy] Returns z component of end point of the track near the last CDC hit. (Belle only, originally stored in mdst_trk_fit.)
- Unit:
cm
- BellePathLength#
[Legacy] Returns the track path length. This is defined from the closest point to the z-axis up to TOF counter. (Belle only).
- Unit:
cm
- BellePi0SigM#
[Legacy] Returns the significance of the \(\pi^0\) mass used in the FEI for B2BII. The significance is calculated as the difference between the reconstructed and the nominal mass divided by the mass uncertainty:
\[\frac{m_{\gamma\gamma} - m_{\pi^0}^\textrm{PDG}}{\sigma_{m_{\gamma\gamma}}}\]Since the \(\pi^0\)’s covariance matrix for B2BII is empty, the latter is calculated using the photon daughters’ covariance matrices.
- BelleTof#
[Legacy] Returns the time of flight of a track. (Belle only).
- Unit:
ns
- BelleTofMass#
[Legacy] Returns the TOF mass calculated from the time of flight and path length. (Belle only). :Unit: GeV/\(\text{c}^2\)
- BelleTofQuality#
[Legacy] Returns the quality flag of the time of flight of a track. Original description from the panther table: 0 if consistency between z of hit by charge Q and corrected times, 1 if zhit from Q NOT consistent with zhit from and correct times. (Belle only).
- BelleTofSigma#
[Legacy] Returns the expected resolution on the time of flight of a track. (Belle only).
- Unit:
ns
- BelledEdx#
[Legacy] Returns the dE/dx measured in the CDC. (Belle only).
- Unit:
keV/cm
- BelledEdxQuality#
[Legacy] Returns the quality flag of the dE/dx measured in the CDC. Sadly no information about the code meaning is given in the original panther tables. (Belle only).
- clusterBelleQuality#
[Legacy] Returns ECL cluster’s quality indicating a good cluster in GSIM (stored in deltaL of ECL cluster object). Belle analysis typically used clusters with quality == 0 in their \(E_{\text{extra ECL}}\) (Belle only).
- goodBelleGamma#
[Legacy] Returns 1.0 if the photon candidate passes the simple region dependent energy selection for Belle data and MC.
\[\begin{split}E > 50 \textrm{ MeV; barrel}\\ E > 100 \textrm{ MeV; forward endcap}\\ E > 150 \textrm{ MeV; backward endcap}\end{split}\]
- goodBelleKshort#
[Legacy] GoodKs Returns true if a \(K_{S}^0\to\pi\pi\) candidate passes the Belle algorithm: a momentum-binned selection including requirements on impact parameter of, and angle between the daughter pions as well as separation from the vertex and flight distance in the transverse plane.
See also
- goodBelleLambda#
[Legacy] Returns 2.0, 1.0, 0.0 as an indication of goodness of \(\Lambda^0\) candidates, based on:
The distance of the two daughter tracks at their interception at z axis,
the minimum distance of the daughter tracks and the IP in xy plane,
the difference of the azimuthal angle of the vertex vector and the momentum vector,
and the flight distance of the Lambda0 candidates in xy plane.
It reproduces the
goodLambda()
function in Belle.goodBelleLambda
selection 1 (selected with:goodBelleLambda>0
) maximizes the signal significance after applyingatcPIDBelle(4,2) > 0.6
, whilegoodBelleLambda
selection 2 (goodBelleLambda>1
) is tighter and maximizes the signal significance of a \(\Lambda^0\) sample without any proton PID cut. However, it might still be beneficial to apply a proton PID cut on top of it. Which combination of proton PID cut andgoodBelleLambda
selection scenario is ideal, is probably analysis-dependent.Warning
goodBelleLambda
is not optimized or tested on Belle II data.See also
BN-684 Lambda selection at Belle. K F Chen et al.
The
FindLambda
class can be found at/belle_legacy/findLambda/findLambda.h
PID for B2BII#
Warning
These variables are to be used only when analysing converted Belle samples.
- atcPIDBelle(i, j)#
[Legacy] Returns Belle’s PID atc variable:
atc_pid(3,1,5,i,j).prob()
. Parameters i,j are signal and background hypothesis: (0 = electron, 1 = muon, 2 = pion, 3 = kaon, 4 = proton) Returns 0.5 in case there is no likelihood found and a factor of 0.5 will appear in the product if any of the subdetectors don’t report a likelihood (Belle behaviour).Warning
The behaviour is different from Belle II PID variables which typically return NaN in case of error.
- eIDBelle#
[Legacy] Returns Belle’s electron ID
eid(3,-1,5).prob()
variable. Returns 0.5 in case there is no likelihood found (Belle behaviour).Warning
The behaviour is different from Belle II PID variables which typically return NaN in case of error.
- muIDBelle#
[Legacy] Returns Belle’s PID
Muon_likelihood()
variable. Returns 0.5 in case there is no likelihood found and returns zero if the muon likelihood is not usable (Belle behaviour).Warning
The behaviour is different from Belle II PID variables which typically return NaN in case of error.
- muIDBelleQuality#
[Legacy] Returns true if Belle’s PID
Muon_likelihood()
is usable (reliable). Returns zero/false if not usable or if there is no PID found.
Miscellaneous#
Other variable that can be handy in development:
- False#
returns always 0, used for testing and debugging.
- True#
returns always 1, used for testing and debugging.
- decayTypeRecoil#
type of the particle decay(no related mcparticle = -1, hadronic = 0, direct leptonic = 1, direct semileptonic = 2,lower level leptonic = 3.
- eventRandom#
[Eventbased] Returns a random number between 0 and 1 for this event. Can be used, e.g. for applying an event prescale.
- infinity#
returns std::numeric_limits<double>::infinity()
- mcMomTransfer2#
Return the true momentum transfer to lepton pair in a B(semi -) leptonic B meson decay.
- Unit:
GeV/c
- minET2ETDist(detName, detLayer, referenceListName, useHighestProbMassForExt=1)#
Returns the distance \(d_{\mathrm{i}}\) in [cm] between the particle and the nearest particle in the reference list at the given detector \(i\)-th layer surface. The definition is based on the track helices extrapolation.
The first argument is the name of the detector to consider.
The second argument is the detector layer on whose surface we search for the nearest neighbour.
The third argument is the reference particle list name used to search for the nearest neighbour.
The fourth (optional) argument is an integer (“boolean”) flag: if 1 (the default, if nothing is set), it is assumed the extrapolation was done with the most probable mass hypothesis for the track fit; if 0, it is assumed the mass hypothesis matching the particle lists’ PDG was used.
Note
This variable requires to run the
TrackIsoCalculator
module first. Note that the choice of input parameters of this metafunction must correspond to the settings used to configure the module!
- minET2ETDistVar(detName, detLayer, referenceListName, variableName)#
Returns the value of the variable for the nearest neighbour to this particle as taken from the reference list at the given detector \(i\)-th layer surface , according to the distance definition of
minET2ETDist
.The first argument is the name of the detector to consider.
The second argument is the detector layer on whose surface we search for the nearest neighbour.
The third argument is the reference particle list name used to search for the nearest neighbour.
The fourth argument is a variable name, e.g.
nCDCHits
.
Note
This variable requires to run the
TrackIsoCalculator
module first. Note that the choice of input parameters of this metafunction must correspond to the settings used to configure the module!
- minET2ETIsoScore(referenceListName, useHighestProbMassForExt, detectorList)#
Returns a particle’s isolation score \(s\) defined as:
\begin{split} s &= \sum_{\mathrm{det}} 1 - \left(-w_{\mathrm{det}} \cdot \frac{\sum_{i}^{N_{\mathrm{det}}^{\mathrm{layers}}} H(i)}{N_{\mathrm{det}}^{\mathrm{layers}}}\right), \\ H(i) &= \begin{cases} 0 & d_{\mathrm{i}} > D_{\mathrm{det}}^{\mathrm{thresh}} \\ 1 & d_{\mathrm{i}} <= D_{\mathrm{det}}^{\mathrm{thresh}}, \\ \end{cases} \end{split}where \(d_{\mathrm{i}}\) is the distance to the closest neighbour at the \(i\)-th layer of the given detector (c.f.,
minET2ETDist
), \(N_{\mathrm{det}}^{\mathrm{layers}}\) is the number of layers of the detector, \(D_{\mathrm{det}}^{\mathrm{thresh}}\) is a threshold length related to the detector’s granularity defined in theTrackIsoCalculator
module, and \(w_{\mathrm{det}}\) are (negative) weights associated to the detector’s impact on PID for this particle type, read from a CDB payload.The score is normalised in [0, 1], where values closer to 1 indicates a well-isolated particle.
The first argument is the reference particle list name used to search for the nearest neighbour.
The second argument is an integer (“boolean”) flag: if 1, it is assumed the extrapolation was done with the most probable mass hypothesis for the track fit; if 0, it is assumed the mass hypothesis matching the particle lists’ PDG was used.
The remaining arguments are a comma-separated list of detector names, which must correspond to the one given to the
TrackIsoCalculator
module.
Note
The PID detector weights \(w_{\mathrm{det}}\) are non-trivial only if
excludePIDDetWeights=false
in theTrackIsoCalculator
module configuration. Otherwise \(w_{\mathrm{det}} = -1\).Note
This variable requires to run the
TrackIsoCalculator
module first. Note that the choice of input parameters of this metafunction must correspond to the settings used to configure the module!
- minET2ETIsoScoreAsWeightedAvg(referenceListName, useHighestProbMassForExt, detectorList)#
Returns a particle’s isolation score \(s\) based on the weighted average:
\[s = 1 - \frac{\sum_{\mathrm{det}} \sum_{i}^{N_{\mathrm{det}}^{\mathrm{layers}}} w_{\mathrm{det}} \cdot \frac{D_{\mathrm{det}}^{\mathrm{thresh}}}{d_{\mathrm{i}}} }{ \sum_{\mathrm{det}} w_{\mathrm{det}}},\]where \(d_{\mathrm{i}}\) is the distance to the closest neighbour at the \(i\)-th layer of the given detector (c.f.,
minET2ETDist
), \(N_{\mathrm{det}}^{\mathrm{layers}}\) is the number of layers of the detector, \(D_{\mathrm{det}}^{\mathrm{thresh}}\) is a threshold length related to the detector’s granularity defined in theTrackIsoCalculator
module, and \(w_{\mathrm{det}}\) are (negative) weights associated to the detector’s impact on PID for this particle type, read from a CDB payload.The score is normalised in [0, 1], where values closer to 1 indicates a well-isolated particle.
The first argument is the reference particle list name used to search for the nearest neighbour.
The second argument is an integer (“boolean”) flag: if 1, it is assumed the extrapolation was done with the most probable mass hypothesis for the track fit; if 0, it is assumed the mass hypothesis matching the particle lists’ PDG was used.
The remaining arguments are a comma-separated list of detector names, which must correspond to the one given to the
TrackIsoCalculator
module.
Note
The PID detector weights \(w_{\mathrm{det}}\) are non-trivial only if
excludePIDDetWeights=false
in theTrackIsoCalculator
module configuration. Otherwise \(\lvert w_{\mathrm{det}} \rvert = 1\).Note
This variable requires to run the
TrackIsoCalculator
module first. Note that the choice of input parameters of this metafunction must correspond to the settings used to configure the module!
- nRemainingTracksInEvent#
Number of tracks in the event - Number of tracks( = charged FSPs) of particle.
- printParticle#
For debugging, print Particle and daughter PDG codes, plus MC match. Returns 0.
- random#
return a random number between 0 and 1 for each candidate. Can be used, e.g. for picking a randomcandidate in the best candidate selection.
- trackMatchType#
-1 particle has no ECL cluster
0 particle has no associated track
1 there is a matched track called connected - region(CR) track match
Warning
Deprecated since version light-2012-minos.
Use better variables like
trackNECLClusters
,clusterTrackMatch
, andnECLClusterTrackMatches
.
FEI Variables#
As known by many analysts by using the isSignal
flag for truth matching
for the tagging B meson from the FEI there is still a peak visible for the
background in e.g. the \(M_{\text{bc}}\) distribution making it hard to
determine e.g. a yield there.
New variables seem to be found to address this problem.
- mostcommonBTagIndex#
By giving e.g. a FEI B meson candidate the B meson index on generator level is determined, where most reconstructed particles can be assigned to. If no B meson found on generator level -1 is returned.
- percentageMissingParticlesBTag#
Get the percentage of missing particles by using the mostcommonBTagIndex. So the number of particles not reconstructed by e.g. the FEI are determined and divided by the number of generated particles using the given index of the B meson. If no B meson found on generator level -1 is returned.
- percentageWrongParticlesBTag#
Get the percentage of wrong particles by using the mostcommonBTagIndex. In this context wrong means that the reconstructed particles originated from the other B meson. The absolute number is divided by the total number of generated FSP from the given B meson index. If no B meson found on generator level -1 is returned.
Beam Background Overlay#
Here is a list of variables useful for studying the Beam Background Overlay events used for producing MC samples (both run-independent and run-dependent).
Warning
These variables will always return NaN
when running on data.
- beamBackgroundEvents#
[Eventbased] Total number of the background overlay events used for producing the file.
- beamBackgroundReuseRate#
[Eventbased] Reuse rate of the background overlay events used for producing the file.
Calibration#
There are several variables also available for calibration experts who are working on cdst
format files.
Warning
Many of these will not work for- and should not be used by- normal analyses.
They have a [Calibration] pretag.
- eventT0#
[Eventbased][Calibration] The Event t0, is the time of the event relative to the trigger time.
Note
The event time can be measured by several sub-detectors including the SVD, CDC, ECL, and TOP. This eventT0 variable is the final combined value of all the event time measurements. Currently, only the SVD and ECL are used in this combination.
- Unit:
ns
- eclEnergy3BWDBarrel#
[Calibration] Returns energy sum of three crystals in backward barrel.
- eclEnergy3BWDEndcap#
[Calibration] Returns energy sum of three crystals in backward endcap.
- eclEnergy3FWDBarrel#
[Calibration] Returns energy sum of three crystals in forward barrel.
- eclEnergy3FWDEndcap#
[Calibration] Returns energy sum of three crystals in forward endcap.
- clusterNumberOfTCs(i, j, k, l)#
[Calibration] Returns the number of TCs for this ECL cluster for a given TC theta ID range \((i, j)\) and hit window \((k, l)\).
- clusterTCFADC(i, j, k, l)#
[Calibration] Returns the total FADC sum related to this ECL cluster for a given TC theta ID range \((i, j)\) and hit window \((k, l)\).
- clusterTCIsMaximum#
[Calibration] Returns True if cluster is related to maximum TC.
- clusterTrigger#
[Calibration] Returns 1.0 if ECL cluster is matched to a trigger cluster (requires to run eclTriggerClusterMatcher (which requires TRGECLClusters in the input file)) and 0 otherwise. Returns -1 if the matching code was not run. NOT FOR PHASE2 DATA!
- eclEnergySumECLCalDigitInECLCluster#
[Eventbased][Calibration] Returns energy sum (in GeV) of all ECLCalDigits that are part of an ECL cluster above eclEnergySumTCECLCalDigitInECLClusterThreshold within TC thetaid 2-15.
- eclEnergySumTC(i, j)#
[Eventbased][Calibration] Returns energy sum (in FADC counts) of all TC cells between two theta ids i<=thetaid<=j, 1 based (1..17)
- eclEnergySumTCECLCalDigit(i, j, k, l)#
[Eventbased][Calibration] Returns energy sum (in GeV) of all TC cells between two theta ids i<=thetaid<=j, 1 based (1..17). k is the sum option: 0 (all), 1 (those with actual TC entries), 2 (sum of ECLCalDigit energy in this TC above threshold). l is the threshold parameter for the option k 2.
- eclEnergySumTCECLCalDigitInECLCluster#
[Eventbased][Calibration] Returns energy sum (in GeV) of all ECLCalDigits if TC is above threshold that are part of an ECLCluster above eclEnergySumTCECLCalDigitInECLClusterThreshold within TC thetaid 2-15.
- eclEnergySumTCECLCalDigitInECLClusterThreshold#
[Eventbased][Calibration] Returns threshold used to calculate eclEnergySumTCECLCalDigitInECLCluster.
- eclEnergyTC(i)#
[Eventbased][Calibration] Returns the energy (in FADC counts) for the \(i\)-th trigger cell (TC), 1 based (1..576).
- eclEnergyTCECLCalDigit(i)#
[Eventbased][Calibration] Returns the energy (in GeV) for the \(i\)-th trigger cell (TC) based on ECLCalDigits, 1 based (1..576).
- eclEventTimingTC#
[Eventbased][Calibration] Returns the ECL TC event time (in ns).
- eclHitWindowTC(i)#
[Eventbased][Calibration] Returns the hit window for the \(i\)-th trigger cell (TC), 1 based (1..576).
- eclMaximumTCId#
[Eventbased][Calibration] Returns the TC ID with maximum FADC value.
- eclNumberOfTCs(i, j, k)#
[Eventbased][Calibration] Returns the number of TCs above threshold (i=FADC counts) for this event for a given theta range (j-k)
- eclTimingTC(i)#
[Eventbased][Calibration] Returns the time (in ns) for the \(i\)-th trigger cell (TC), 1 based (1..576).
- eclTimingTCECLCalDigit(i)#
[Eventbased][Calibration] Returns the time (in ns) for the \(i\)-th trigger cell (TC) based on ECLCalDigits, 1 based (1..576)
7.3.3. Collections and Lists#
To avoid very long lists of variable names in variablesToNtuple
,
it is possible to use collections of variables or lists of variables instead.
Lists of variables are just python lists of variables names. One can use the list in the steering file as follows:
# Defining the list
my_list = ['p','E']
# Passing it as an argument to variablesToNtuple
modularAnalysis.variablesToNtuple(variables=my_list, ...)
It is also possible to create user-defined variable collections. The name of the variable collection can be treated as a variable name.
- variables.utils.add_collection(list_of_variables: Iterable[str], collection_name: str) str [source]#
The function creates variable collection from the given list of variables It wraps the
VariableManager.addCollection
method which is not particularly user-friendly.Example
Defining the collection >>> variables.utils.add_collection([‘p’,’E’], “my_collection”)
Passing it as an argument to variablesToNtuple >>> modularAnalysis.variablesToNtuple(variables=[‘my_collection’], …)
Predefined collections#
We provide several predefined lists of variables. For each predefined list, there is a collection with the same name:
- variables.collections.belle_track_hit = ['BelleFirstCDCHitX', 'BelleFirstCDCHitY', 'BelleFirstCDCHitZ', 'BelleLastCDCHitX', 'BelleLastCDCHitY', 'BelleLastCDCHitZ']#
Belle Track CDC hit variables
- variables.collections.cluster = ['clusterKlId', 'clusterPulseShapeDiscriminationMVA', 'clusterHasPulseShapeDiscrimination', 'clusterNumberOfHadronDigits', 'clusterDeltaLTemp', 'minC2TDist', 'nECLClusterTrackMatches', 'clusterZernikeMVA', 'clusterReg', 'clusterAbsZernikeMoment40', 'clusterAbsZernikeMoment51', 'clusterBelleQuality', 'clusterClusterID', 'clusterConnectedRegionID', 'clusterE1E9', 'clusterE9E21', 'clusterE9E25', 'clusterEoP', 'clusterErrorE', 'clusterErrorPhi', 'clusterErrorTheta', 'clusterErrorTiming', 'clusterHighestE', 'clusterHasFailedErrorTiming', 'clusterHasFailedTiming', 'clusterHasNPhotons', 'clusterHasNeutralHadron', 'clusterLAT', 'clusterNHits', 'clusterPhi', 'clusterR', 'clusterSecondMoment', 'clusterTheta', 'clusterTiming', 'clusterTrackMatch', 'goodBelleGamma']#
Cluster-related variables
- variables.collections.cluster_average = ['maxWeightedDistanceFromAverageECLTime', 'weightedAverageECLTime']#
Cluster averages
- variables.collections.dalitz_3body = ['daughterInvM(0, 1)', 'daughterInvM(0, 2)', 'daughterInvM(1, 2)']#
Dalitz masses for three body decays
- variables.collections.deltae_mbc = ['Mbc', 'deltaE']#
Replacement for DeltaEMbc
- variables.collections.event_kinematics = ['missingMomentumOfEvent', 'missingMomentumOfEvent_Px', 'missingMomentumOfEvent_Py', 'missingMomentumOfEvent_Pz', 'missingMomentumOfEvent_theta', 'missingMomentumOfEventCMS', 'missingMomentumOfEventCMS_Px', 'missingMomentumOfEventCMS_Py', 'missingMomentumOfEventCMS_Pz', 'missingMomentumOfEventCMS_theta', 'missingEnergyOfEventCMS', 'missingMass2OfEvent', 'visibleEnergyOfEventCMS', 'totalPhotonsEnergyOfEvent']#
Variables created by event kinematics module
- variables.collections.event_level_cluster = ['nECLOutOfTimeCrystals', 'nECLOutOfTimeCrystalsBWDEndcap', 'nECLOutOfTimeCrystalsBarrel', 'nECLOutOfTimeCrystalsFWDEndcap', 'nRejectedECLShowers', 'nRejectedECLShowersBWDEndcap', 'nRejectedECLShowersBarrel', 'nRejectedECLShowersFWDEndcap', 'nKLMMultistripHits', 'nKLMMultistripHitsBWDEndcap', 'nKLMMultistripHitsBarrel', 'nKLMMultistripHitsFWDEndcap', 'nECLShowers', 'nECLShowersBWDEndcap', 'nECLShowersBarrel', 'nECLShowersFWDEndcap', 'nECLLocalMaximums', 'nECLLocalMaximumsBWDEndcap', 'nECLLocalMaximumsBarrel', 'nECLLocalMaximumsFWDEndcap', 'nECLTriggerCells', 'nECLTriggerCellsBWDEndcap', 'nECLTriggerCellsBarrel', 'nECLTriggerCellsFWDEndcap']#
Cluster-related event variables
- variables.collections.event_level_tracking = ['nExtraCDCHits', 'nExtraCDCHitsPostCleaning', 'hasExtraCDCHitsInLayer(0)', 'hasExtraCDCHitsInLayer(1)', 'hasExtraCDCHitsInLayer(2)', 'hasExtraCDCHitsInLayer(3)', 'hasExtraCDCHitsInLayer(4)', 'hasExtraCDCHitsInLayer(5)', 'hasExtraCDCHitsInLayer(6)', 'hasExtraCDCHitsInLayer(7)', 'nExtraCDCSegments', 'trackFindingFailureFlag']#
Event level tracking variables
- variables.collections.event_shape = ['foxWolframR1', 'foxWolframR2', 'foxWolframR3', 'foxWolframR4', 'harmonicMomentThrust0', 'harmonicMomentThrust1', 'harmonicMomentThrust2', 'harmonicMomentThrust3', 'harmonicMomentThrust4', 'cleoConeThrust0', 'cleoConeThrust1', 'cleoConeThrust2', 'cleoConeThrust3', 'cleoConeThrust4', 'cleoConeThrust5', 'cleoConeThrust6', 'cleoConeThrust7', 'cleoConeThrust8', 'sphericity', 'aplanarity', 'thrust', 'thrustAxisCosTheta']#
Event shape variables
- variables.collections.extra_energy = ['roeEextra()']#
Extra energy variables
- variables.collections.flight_info = ['flightTime', 'flightDistance', 'flightTimeErr', 'flightDistanceErr']#
Flight info variables
- variables.collections.inv_mass = ['M', 'ErrM', 'SigM', 'InvM']#
Replacement for InvMass tool
- variables.collections.kinematics = ['px', 'py', 'pz', 'pt', 'p', 'E']#
Replacement to Kinematics tool
- variables.collections.klm_cluster = ['klmClusterKlId', 'klmClusterTiming', 'klmClusterPositionX', 'klmClusterPositionY', 'klmClusterPositionZ', 'klmClusterInnermostLayer', 'klmClusterLayers', 'klmClusterEnergy', 'klmClusterMomentum', 'klmClusterIsBKLM', 'klmClusterIsEKLM', 'klmClusterIsForwardEKLM', 'klmClusterIsBackwardEKLM', 'klmClusterTheta', 'klmClusterPhi', 'nKLMClusterTrackMatches', 'nMatchedKLMClusters']#
KLM cluster information
- variables.collections.mc_event_kinematics = ['genMissingMass2OfEvent', 'genMissingEnergyOfEventCMS', 'genMissingMomentumOfEventCMS', 'genTotalPhotonsEnergyOfEvent', 'genVisibleEnergyOfEventCMS']#
Variables created by MC version of event kinematics module
- variables.collections.mc_flight_info = ['mcFlightTime', 'mcFlightDistance']#
MC true flight info variables
- variables.collections.mc_kinematics = ['mcE', 'mcP', 'mcPT', 'mcPX', 'mcPY', 'mcPZ', 'mcPhi']#
Replacement for MCKinematics tool
- variables.collections.mc_tag_vertex = ['mcDeltaTau', 'mcDeltaT', 'mcDeltaBoost', 'mcTagVLBoost', 'mcTagVOBoost', 'mcLBoost', 'mcOBoost', 'mcTagVx', 'mcTagVy', 'mcTagVz']#
Tag-side related MC true variables
- variables.collections.mc_truth = ['isSignal', 'mcErrors', 'mcPDG']#
Replacement for MCTruth tool
- variables.collections.mc_variables = ['genMotherID', 'genMotherP', 'genMotherPDG', 'genParticleID', 'isCloneTrack', 'mcDecayVertexX', 'mcDecayVertexY', 'mcDecayVertexZ', 'mcDecayTime', 'mcE', 'mcErrors', 'mcInitial', 'mcP', 'mcPDG', 'mcPT', 'mcPX', 'mcPY', 'mcPZ', 'mcPhi', 'mcVirtual', 'nMCMatches']#
Truth-matching related variables
- variables.collections.mc_vertex = ['mcDecayVertexX', 'mcDecayVertexY', 'mcDecayVertexZ', 'mcDecayVertexFromIPDistance', 'mcDecayVertexRho', 'mcProductionVertexX', 'mcProductionVertexY', 'mcProductionVertexZ']#
Replacement for MCVertex tuple tool
- variables.collections.momentum_uncertainty = ['E_uncertainty', 'pxErr', 'pyErr', 'pzErr']#
Replacement for MomentumUnertainty tool
- variables.collections.pid = ['electronID', 'muonID', 'pionID', 'kaonID', 'protonID', 'deuteronID', 'binaryPID(11, 211)', 'binaryPID(13, 211)', 'binaryPID(211, 321)', 'binaryPID(321, 2212)', 'binaryPID(211, 2212)']#
PID variables
- variables.collections.reco_stats = ['nTracks']#
Replacement for RecoStats tool
- variables.collections.recoil_kinematics = ['pRecoil', 'eRecoil', 'mRecoil', 'pRecoilPhi', 'pRecoilTheta', 'pxRecoil', 'pyRecoil', 'pzRecoil']#
Recoil kinematics related variables
- variables.collections.roe_kinematics = ['roeE()', 'roeM()', 'roeP()', 'roeMbc()', 'roeDeltae()']#
Rest-Of-Event kinematics
- variables.collections.roe_multiplicities = ['nROE_Charged()', 'nROE_Photons()', 'nROE_NeutralHadrons()']#
Replacement for ROEMultiplicities tool
- variables.collections.tag_vertex = ['DeltaT', 'DeltaTErr', 'DeltaZ', 'DeltaZErr', 'DeltaBoost', 'DeltaBoostErr', 'TagVLBoost', 'TagVLBoostErr', 'TagVOBoost', 'TagVOBoostErr', 'TagVpVal', 'TagVNDF', 'TagVChi2', 'TagVChi2IP', 'TagVx', 'TagVxErr', 'TagVy', 'TagVyErr', 'TagVz', 'TagVzErr']#
CPV and Tag-side related variables
- variables.collections.track = ['dr', 'dx', 'dy', 'dz', 'd0', 'z0', 'pValue', 'ndf']#
Tracking variables, replacement for Track tool
- variables.collections.track_hits = ['nCDCHits', 'nPXDHits', 'nSVDHits', 'nVXDHits']#
Replacement for TrackHits tool
- variables.collections.trackfit_parameters = ['omega', 'omegaErr', 'tanLambda', 'tanLambdaErr', 'd0', 'd0Err', 'z0', 'z0Err', 'phi0', 'phi0Err', 'pValue', 'ndf']#
Track fit parameters
- variables.collections.vertex = ['distance', 'significanceOfDistance', 'dx', 'dy', 'dz', 'x', 'y', 'z', 'x_uncertainty', 'y_uncertainty', 'z_uncertainty', 'dr', 'dphi', 'dcosTheta', 'prodVertexX', 'prodVertexY', 'prodVertexZ', 'prodVertexXErr', 'prodVertexYErr', 'prodVertexZErr', 'chiProb']#
Replacement for Vertex tuple tool
7.3.4. Operations with variable lists#
It is possible to create new variable lists using meta-variables. For example,
one can define list of kinematic variables in LAB frame and create another
lists of kinematic variables in CMS using useCMSFrame(variable)
meta-variable:
from variables.utils import create_aliases
# Replacement to Kinematics tool
kinematics = ['px', 'py', 'pz', 'pt', 'p', 'E']
# Kinematic variables in CMS
cms_kinematics = create_aliases(kinematics, "useCMSFrame({variable})", "CMS")
Now we can use the list of aliases cms_kinematics
and add them to the
output in one go or modify them further. The following functions are provided
to help to easily create aliases.
- variables.utils.create_aliases(list_of_variables: Iterable[str], wrapper: str, prefix='') List[str] [source]#
The function creates aliases for variables from the variables list with given wrapper and returns list of the aliases.
If the variables in the list have arguments (like
useLabFrame(p)
) all non-alphanumeric characters in the variable will be replaced by underscores (for exampleuseLabFrame_x
) for the alias name.>>> list_of_variables = ['M','p','matchedMC(useLabFrame(px))'] >>> wrapper = 'daughter(1,{variable})' >>> prefix = 'pref' >>> print(create_aliases(list_of_variables, wrapper, prefix)) ['pref_M', 'pref_p', 'pref_matchedMC_useLabFrame_px'] >>> from variables import variables >>> variables.printAliases() [INFO] ===================================== [INFO] The following aliases are registered: [INFO] pref_M --> daughter(1,M) [INFO] pref_matchedMC_useLabFrame_px --> daughter(1,matchedMC(useLabFrame(px))) [INFO] pref_p --> daughter(1,p) [INFO] =====================================
- Parameters:
- Returns:
new variables list
- Return type:
- variables.utils.create_aliases_for_selected(list_of_variables: List[str], decay_string: str, prefix: str | List[str] | None = None, *, use_names=True, always_include_indices=False, use_relative_indices=False) List[str] [source]#
The function creates list of aliases for given variables so that they are calculated for particles selected in decay string. That is for each particle selected in the decay string an alias is created to calculate each variable in the
list_of_variables
.If
use_names=True
(the default) then the names of the aliases are assigned as follows:If names are unambiguous, it’s semi-laconic DecayString style: The aliases will be prefixed with the names of all parent particle names separated by underscore. For example given the decay string
B0 -> [D0 -> ^pi+ K-] pi0
the aliases for thepi+` will start with ``D0_pi_
followed by the variable name.>>> list_of_variables = ['M','p'] >>> decay_string = 'B0 -> [D0 -> ^pi+ K-] pi0' >>> create_aliases_for_selected(list_of_variables, decay_string) ['D0_pi_M', 'D0_pi_p'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'D0_pi_M' --> 'daughter(0,daughter(0,M))' [INFO] 'D0_pi_p' --> 'daughter(0,daughter(0,p))' [INFO] =========================
If names are ambiguous because there are multiple daughters with the same name these particles will be followed by their daughter index. For example given the decay string
B0 -> [D0 -> ^pi+:1 ^pi-:2 ^pi0:3 ] ^pi0:4
will create aliases with the following prefixes for particle with the corresponding number as list name:D0_pi_0_
D0_pi_1_
D0_pi0_
pi0_
>>> list_of_variables = ['M','p'] >>> decay_string = 'B0 -> [D0 -> ^pi+ ^pi- ^pi0] ^pi0' >>> create_aliases_for_selected(list_of_variables, decay_string) ['D0_pi_0_M', 'D0_pi_0_p', 'D0_pi_1_M', 'D0_pi_1_p', 'D0_pi0_M', 'D0_pi0_p', 'pi0_M', 'pi0_p'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'D0_pi0_M' --> 'daughter(0,daughter(2,M))' [INFO] 'D0_pi0_p' --> 'daughter(0,daughter(2,p))' [INFO] 'D0_pi_0_M' --> 'daughter(0,daughter(0,M))' [INFO] 'D0_pi_0_p' --> 'daughter(0,daughter(0,p))' [INFO] 'D0_pi_1_M' --> 'daughter(0,daughter(1,M))' [INFO] 'D0_pi_1_p' --> 'daughter(0,daughter(1,p))' [INFO] 'D0_pi_M' --> 'daughter(0,daughter(0,M))' [INFO] 'D0_pi_p' --> 'daughter(0,daughter(0,p))' [INFO] 'pi0_M' --> 'daughter(1,M)' [INFO] 'pi0_p' --> 'daughter(1,p)' [INFO] =========================
The user can select to always include the index even for unambiguous particles by passing
always_include_indices=True
The user can choose two different numbering schemes: If
use_relative_indices=False
the original decay string indices will be used if a index is added to a particle name.But if
use_relative_indices=True
the indices will just start at zero for each particle which is part of the prefixes. For example forB0-> e+ ^e-
>>> create_aliases_for_selected(['M'], 'B0-> mu+ e- ^e+ ^e-', use_relative_indices=False) ['e_2_M', 'e_3_M'] >>> create_aliases_for_selected(['M'], 'B0-> mu+ e- ^e+ ^e-', use_relative_indices=True) ['e_0_M', 'e_1_M']
If
use_names=False
the aliases will just start with the daughter indices of all parent particles prefixed with ad
and separated by underscore. So for the previous exampleB0 -> [D0 -> ^pi+:1 ^pi-:2 ^pi0:3 ] ^pi0:4
this would result in aliases starting withd0_d0_
d0_d1_
d0_d2_
d1_
In this case the
always_include_indices
anduse_relative_indices
arguments are ignored.The naming can be modified by providing a custom prefix for each selected particle. In this case the parameter
prefix
needs to be either a simple string if only one particle is selected or a list of strings with one prefix for each selected particle.>>> list_of_variables = ['M','p'] >>> decay_string = 'B0 -> [D0 -> ^pi+ ^pi- pi0] pi0' >>> create_aliases_for_selected(list_of_variables, decay_string, prefix=['pip', 'pim']) ['pip_M', 'pip_p', 'pim_M', 'pim_p'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'pim_M' --> 'daughter(0,daughter(1,M))' [INFO] 'pim_p' --> 'daughter(0,daughter(1,p))' [INFO] 'pip_M' --> 'daughter(0,daughter(0,M))' [INFO] 'pip_p' --> 'daughter(0,daughter(0,p))' [INFO] =========================
If the mother particle itself is selected the input list of variables will also be added to the returned list of created aliases. If custom prefixes are supplied then aliases will be created for the mother particle as well:
>>> create_aliases_for_selected(['M', 'p'], '^B0 -> pi+ ^pi-') ['M', 'p', 'pi_M', 'pi_p'] >>> create_aliases_for_selected(['M', 'p'], '^B0 -> pi+ ^pi-', prefix=['MyB', 'MyPi']) ['MyB_M', 'MyB_p', 'MyPi_M', 'MyPi_p']
- Parameters:
decay_string (str) – Decay string with selected particles
prefix (str, list(str)) – Custom prefix for all selected particles
use_names (bool) – Include the names of the particles in the aliases
always_include_indices (bool) – If
use_names=True
include the decay index of the particles in the alias name even if the particle could be uniquely identified without them.use_relative_indices (bool) – If
use_names=True
use a relative indicing which always starts at 0 for each particle appearing in the alias names independent of the absolute position in the decay string
- Returns:
new variables list
- Return type:
- variables.utils.create_daughter_aliases(list_of_variables: Iterable[str], indices: int | Iterable[int], prefix='', include_indices=True) List[str] [source]#
Create Aliases for all variables for a given daughter hierarchy
- Parameters:
list_of_variables (list(str)) – list of variables to create aliases for
indices (int or list(int)) – index of the daughter, grand-daughter, grand-grand-daughter, and so forth
prefix (str) – optional prefix to prepend to the aliases
include_indices (bool) – if set to True (default) the aliases will contain the daughter indices as dX_dY_dZ…
- Returns:
new variables list
- Return type:
create aliases for the second daughter as “d1_E”, “d1_M” (daughters start at 0)
>>> create_daughter_aliases(["E", "m"], 1) ['d1_E', 'd1_m'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'd1_E' --> 'daughter(1,E)' [INFO] 'd1_m' --> 'daughter(1,m)' [INFO] =========================
create aliases for the first grand daughter of the second daughter, starting with “my” and without including the indices, resulting in “my_E”, “my_m”
>>> create_daughter_aliases(["E", "m"], [1, 0], prefix="my", include_indices=False) ['my_E', 'my_m'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'my_E' --> 'daughter(1,daughter(0,E))' [INFO] 'my_m' --> 'daughter(1,daughter(0,m))' [INFO] =========================
create aliases for the second grand grand daughter of the third grand daughter of the fifth daughter, starting with my and including the indices, resulting in “my_d4_d2_d1_E”, “my_d4_d2_d1_m”
>>> create_daughter_aliases(["E", "m"], [4, 2, 1], prefix="my") ['my_d4_d2_d1_E', 'my_d4_d2_d1_m'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'my_d4_d2_d1_E' --> 'daughter(4,daughter(2,daughter(1,E))' [INFO] 'my_d4_d2_d1_m' --> 'daughter(4,daughter(2,daughter(1,m))' [INFO] =========================
- variables.utils.create_mctruth_aliases(list_of_variables: Iterable[str], prefix='mc') List[str] [source]#
The function wraps variables from the list with ‘matchedMC()’.
>>> list_of_variables = ['M','p'] >>> create_mctruth_aliases(list_of_variables) ['mc_M', 'mc_p'] >>> from variables import variables >>> variables.printAliases() [INFO] ========================= [INFO] Following aliases exists: [INFO] 'mc_M' --> 'matchedMC(M)' [INFO] 'mc_p' --> 'matchedMC(p)' [INFO] =========================
- variables.utils.create_isSignal_alias(aliasName, flags)[source]#
Make a
VariableManager
alias for a customizedisSignal
, which accepts specified mc match errors.See also
see Truth-matching for a definition of the mc match error flags.
The following code defines a new variable
isSignalAcceptMissingGammaAndMissingNeutrino
, which is same asisSignal
, but also accepts missing gamma and missing neutrino>>> create_isSignal_alias("isSignalAcceptMissingGammaAndMissingNeutrino", [16, 8])
Logically, this
isSignalAcceptMissingGammaAndMissingNeutrino
=isSignalAcceptMissingGamma
||isSignalAcceptMissingNeutrino
.In the example above, create_isSignal_alias() creates
isSignalAcceptMissingGammaAndMissingNeutrino
by unmasking (setting bits to zero) thec_MissGamma
bit (16 or 0b00010000) andc_MissNeutrino
bit (8 or 0b00001000) in mcErrors.For more information, please check this example script.
7.3.5. Miscellaneous helpers for using variables#
7.3.6. Writing your own variable#
The code of VariableManager lives inside the analysis package. If you want to write your own variables you have a couple of options. You can (and should) try to make your variables general, so that they are useful for many collaborators. In this case, we recommend you make a merge request. Then your variables will be made available in a central release to many people.
In case you have something really analysis-specific that no one else will need. You can still use the VariableManager.
Below are step-by-step instructions for implementation of helicity angle for arbitrary granddaughter particle.
Step 1. Check whether your function would fit in any of the existing source files#
If yes, go to the next step.
If not, create new header/source files. In case of our example, we will create AngularVariables.h
and AngularVariables.cc
AngularVariables.h
in analysis/VariableManager/include/
:
#pragma once
// include VariableManager
#include <analysis/VariableManager/Manager.h>
// include the Belle II Particle class
#include <analysis/dataobjects/Particle.h>
// put variable in the Belle2::Variable namespace
namespace Belle2 {
namespace Variable {
// Your code goes here
} // Variable namespace
} // Belle2 namespace
AngularVariables.cc
in analysis/VariableManager/src/
:
// Own include
#include <analysis/VariableManager/AngularVariables.h>
// put variable in the Belle2::Variable namespace
namespace Belle2 {
namespace Variable {
// Your code goes here
} // Variable namespace
} // Belle2 namespace
Step 2. Add function definition in the header file#
Here we define a method helicityAngle that has 3 arguments:
pointer to a Particle
index of the daughter Particle
index of the granddaughter (daughter’s daughter) Particle
in the AngularVariable.h
header file. The return value of every variable has to be double.
/**
* returns cosine of the helicity angle: angle between granddaughter and this particle in the daughter's rest frame.
* The daughter and granddaughter particles are specified with the additional parameter.
*/
double helicityAngle(const Particle* particle, const std::vector<double>& daughter_indices);
Step 3. Implement the function in the source file#
Info on getters for the Particle class
Info on getters for the LorentzVector class
Pictorial definition of the helicity angle
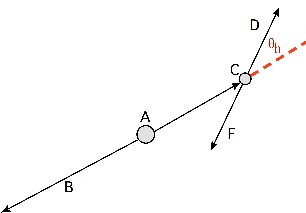
double helicityAngle(const Particle* particle, const std::vector<double>& daughter_indices) {
// for the calculation of the helicity angle we need particle (=particle in the argument)
// its daughter and granddaughter. The particle is given as an argument, but the daughter
// and granddaughter are specified as the indices in the vector (second argument)
// daughter_indices[0] = index of the daughter
// daughter_indices[1] = index of the granddaughter
if (!particle)
return std::numeric_limits<float>::quiet_NaN();
int nDaughters = particle->getNDaughters();
if(nDaughters < 2)
return std::numeric_limits<float>::quiet_NaN();
if (daughter_indices.size() != 2)
return std::numeric_limits<float>::quiet_NaN();
// get the daughter particle
int daughterIndex = daughter_indices[0];
const Particle *daughter = particle->getDaughter(daughterIndex);
nDaughters = daughter->getNDaughters();
if(nDaughters < 2)
return std::numeric_limits<float>::quiet_NaN();
// get the granddaughter
int grandDaughterIndex = daughter_indices[1];
const Particle *grandDaughter = daughter->getDaughter(grandDaughterIndex);
// do the calculation
PxPyPzEVector particle4Vector = particle->get4Vector();
PxPyPzEVector daughter4Vector = daughter->get4Vector();
PxPyPzEVector gDaughter4Vector = grandDaughter->get4Vector();
Boost boost2daughter(daughter4Vector.BoostToCM());
particle4Vector = boost2daughter * particle4Vector;
gDaughter4Vector = boost2daughter * gDaughter4Vector;
B2Vector3D particle3Vector = particle4Vector.Vect();
B2Vector3D gDaughter3Vector = gDaughter4Vector.Vect();
double numerator = gDaughter3Vector.Dot(particle3Vector);
double denominator = (gDaughter3Vector.Mag())*(particle3Vector.Mag());
return numerator/denominator;
}
Step 4. Register the new variable#
At the end of the source file, add the following lines:
VARIABLE_GROUP("AngularVariables");
REGISTER_VARIABLE("helicityAngle(i,j)", helicityAngle,
"cosine of the angle between particle->getDaughter(i)->getDaughter(j) and this particle in the particle->getDaughter(i) rest frame.");
Step 5. Compile#
Execute scons in your local release directory
Step 6. Done!#
You can check if your variable is visible to VariableManager
>>> basf2 analysis/scripts/variables.py
AngularVariables: helicityAngle(i,j) cosine of the angle between particle->getDaughter(i)->getDaughter(j) and this particle in the particle->getDaughter(i) rest frame.
You can use your variable in the same way as you use standard variables.
How to use my variable at grid?#
Prepare the environment with the
b2analysis-create
tool.
>>> b2analysis-create myanalysis <current central release, e.g. release-04-00-00>
>>> cd myanalysis
>>> b2setup
Define the new variables/functions in a .cc and register them with the variable manager. This means that in the new .cc you should add:
#include <framework/core/Module.h>
#include <analysis/VariableManager/Manager.h>
#include <analysis/dataobjects/Particle.h>
namespace Belle2 {
namespace Variable {
double myVarFunction(const Particle* particle) { **your code** }
VARIABLE_GROUP("CUSTOM_VARIABLES");
REGISTER_VARIABLE("myVar", myVarFunction, "My custom variable");
}
// Create an empty module which does nothing at all. What it does is allowing
// basf2 to easily find the library and load it from the steering file
class EnableMyVariableModule: public Module {}; // And register this module to create a .map lookup file.
REG_MODULE(EnableMyVariable);
}
Then:
Run scons and you will get a
.so
and a.b2modmap
files inmodules/Linux_x86_64/opt
.Load the libraries and make the variables available you need to add these lines to your steering file:
import basf2
basf2.register_module("EnableMyVariable") # This is the relevant line: now you can use your variable
from variables import variables
print(variables.getVariable("myVar").description)
These lines will add the local directory to the module search path and instantiate the module we created. This is enough to load the library and register the variables. Now you should be able to use your custom variables for your analysis and there is no need to add this module to the path, it just needs to be registered to load the library.
In the end you can run your analysis on the grid adding the .so
and .map
files to the input sandbox
with the -f option of gbasf2
:
>>> gbasf2 ./steering.py -p project -i dataset -f myanalysis.so myanalysis.b2modmap
Warning
This line implies that you already have working gbasf2
installation and gbasf2
syntax didn’t
change since the moment of writing. Please refer to the gbasf2 documentation for more details.